Build Node.js RESTful APIs in 5 minutes (Updated 2019)
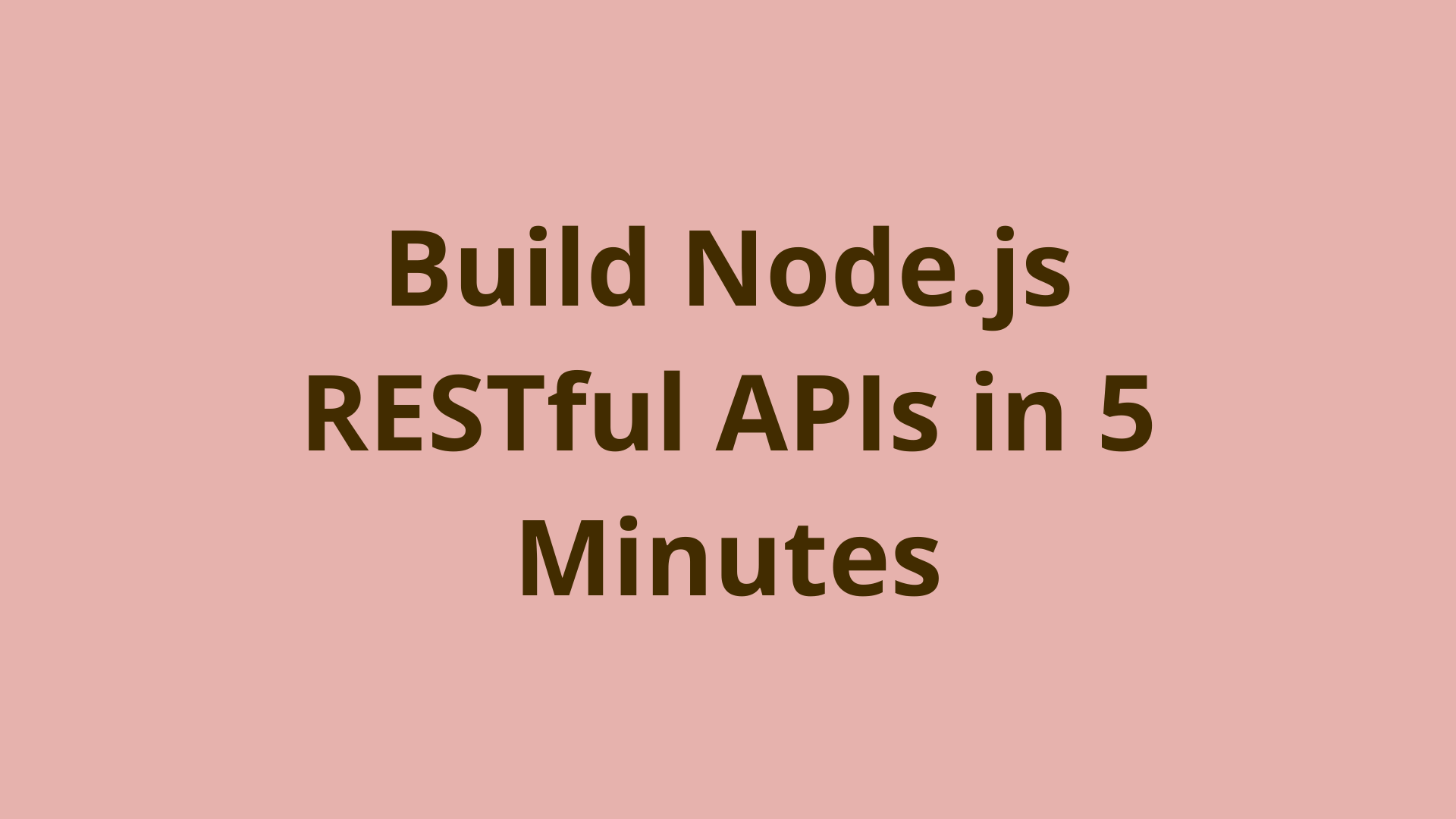
ADVERTISEMENT
Table of Contents
- Introduction
- What is REST?
- Some Benefits of REST?
- Get Your REST API Template
- Add a New API Route
- Conclusion
Introduction
In this article, we'll discuss using Node.js to build a RESTful APIs in 5 minutes. Feel free to follow along with our YouTube tutorial here:
What is REST?
REST or Representational State Transfer is another way of implementing a Web Service. It is the ideology that everything is a resource. Every resource is identified by a unique uniform resource indicator (URI). An important point to note with REST is that it is stateless. This means that when you access the REST web service to retrieve data, you receive the most current state of the resource (ie. most recent data available). You might go for a quick walk, come back, send another request to the web service, and now you have different data. REST APIs usually take advantage of HTTP, however it's also possible to use it with other protocols.
Some Benefits of REST?
- Lightweight
- Scalable - Supports large numbers of requests
- Reliable - No single point of failure
Get Your REST API Template
git clone https://github.com/majikarp/node-rest-api-template.git
cd node-rest-api-template
npm install
node index.js
Your REST API default route is now running at http://localhost:3000
You should now see the following response...
{"hello":"world"}
As you can see this is a very simple example of a REST API. The index.js
file is the entry point to the application and will start running your REST API. Let's take a look at the contents of index.js
to see how simple it really is:
// Require the framework and instantiate it
const fastify = require('fastify')({ logger: true })
// Declare an API route
fastify.get('/', async (request, reply) => {
return { hello: 'world' }
})
// Run the server!
const start = async () => {
try {
await fastify.listen(3000)
fastify.log.info(`server listening on ${fastify.server.address().port}`)
} catch (err) {
fastify.log.error(err)
process.exit(1)
}
}
start()
This project uses Fastify as the default framework to serve the web resources of the API. So what can we do with a REST API? Say, for example, you have been asked to build an E-commerce API, publishing an "inventory" of items and their prices, so that other systems can see your inventory in real-time. By serving your data via the endpoint /inventory
, this will allow easy access to view the inventory data.
Add a New API Route
Let's open the index.js
file and add a new fastify route for the inventory. We want want to build the list of items and their associated prices as a fastify route. So how do we go about doing that? We simply create a new endpoint and return a JSON object with the items and their corresponding prices. Here is what the updated index.js file...
// Require the framework and instantiate it
const fastify = require('fastify')({ logger: true })
// Declare an API route
fastify.get('/', async (request, reply) => {
return { hello: 'world' }
})
// Declare an API route - "inventory"
fastify.get('/inventory', async (request, reply) => {
return {
hat: 5,
socks: 5,
shirt: 25,
pants: 40
}
})
// Run the server!
const start = async () => {
try {
await fastify.listen(3000)
fastify.log.info(`server listening on ${fastify.server.address().port}`)
} catch (err) {
fastify.log.error(err)
process.exit(1)
}
}
start()
Now, restart your REST API server...
node index.js
Your REST API route is now running at http://localhost:3000/inventory
After this address, you should now see the following result...
{"hat":5,"socks":5,"shirt":25,"pants":40}
The above data are the inventory items and their associated prices. This data can be parsed and used by other systems. It is in JSON format (Javascript-Object-Notation), an open-standard file format that uses human-readable text.
Conclusion
Why not try deploying your application and see it running in action? After deploying, your inventory API endpoint should behave in the same way. By accessing your URL https://yourhostname.com/inventory
you should see the same result!
We hope you enjoyed this tutorial on building a REST API in 5 minutes! You can find the template for this tutorial here. If you liked the template, don't forget to leave a star!'
Final Notes
Recommended product: Coding Essentials Guidebook for Developers