Python Absolute Value
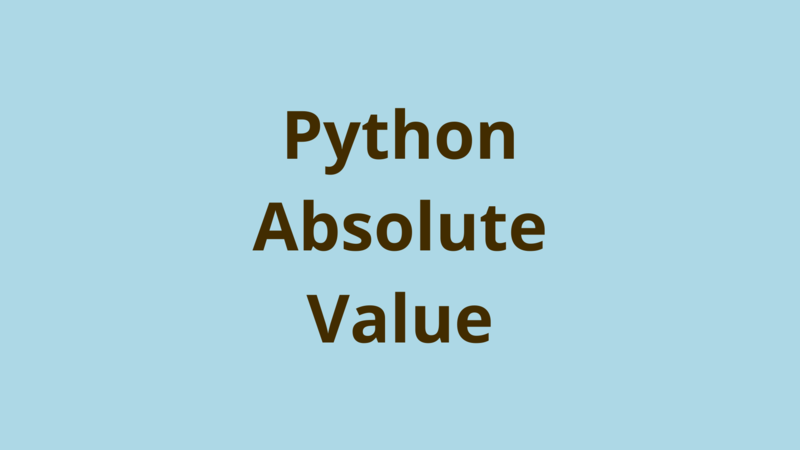
ADVERTISEMENT
Table of Contents
- Introduction
- Overview of the Python Absolute Value Function
- Absolute Value of an Integer
- Absolute Value of a Floating Point Number
- Absolute Value of a Complex Number
- Absolute Value of a Special Numbers
- Absolute Value of a List of Numbers
- Overview of the fabs function
- Summary
- Next Steps
Introduction
Most programming languages come prepackaged with functions that allow you to perform basic mathematical calculations. Developers often take advantage of such operations to build useful software applications for end users.
For example, say you were interested in building a ride sharing application. The app would need to keep track of various factors, including the total distance traveled between two locations. If your program stores the direction of travel as signed integers---for instance, +3 miles in one direction and -7 miles in its opposite - then you'd need to consider the absolute value of each of these in order to find the total distance traveled.
You could easily build such an application using the abs() function in Python function. It's part of the standard library, meaning it comes prepackaged with every installation of Python and is ready for you to use as soon as the installation is finished.
In this article, you'll take a quick look at how to find the absolute value of a given number in Python.
Overview of the Python Absolute Value Function
abs()
is a function that takes in a number and returns its absolute value. The basic syntax is as follows:
abs(x)
This function takes in one positional argument, x
, which must be an item with a numeric data type.
In the following sections, you'll see how to find the absolute value of various numeric data types in Python.
Absolute Value of an Integer
Integers are the most common data type for which one would need to find the absolute value. Doing so will return the total distance the given number is from zero on the number line. Python will quickly determine this value for you. Simply pass the signed integer in as the x
parameter to abs()
like so:
>>> abs(12345)
12345
>>> abs(-12345)
12345
Here, you want to find the absolute value (total distance from zero) of both +12345 and -12345. You pass each of these integers in to abs(), taking care to include the sign where necessary, and the Python function returns the absolute value (which is 12345) for both numbers.
Absolute Value of a Floating Point Number
Floating point numbers behave similarly when you pass them in to abs(). The following code block calculates the absolute value of a negative floating point number:
>>> abs(-73.48)
73.48
-73.48 is 73.48 units away from zero in the negative direction, and abs() correctly returns this value.
Absolute Value of a Complex Number
When you pass a complex number in to the Python abs() function, the return value you get will be slightly different than just the number's distance from zero. This is because the absolute value of a complex number actually returns the magnitude, or the length of the resulting vector when measured from the origin. In other words, when applied to a complex number, abs() will return the theoretical distance between the origin and the coordinates that the complex number represents:
>>> abs(3+4j)
5.0
Here, the result tells us that the complex number 3+4j
represents a vector that is a distance of length 5 away from the origin in the vector space.
Absolute Value of a Special Numbers
Python abs() is also capable of returning the absolute value of special numbers. These include Boolean truth values and concepts like infinity.
Unsurprisingly, the absolute value of a bool resolves to its related numeric value:
>>> abs(True)
1
>>> abs(False)
0
Remember, the absolute value is the distance from zero. Since True
is rendered numerically as 1, and 1 is one unit away from zero, then abs(True) returns 1. Similarly, False
is rendered numerically as 0, which is at the same location as zero on the number line, so its absolute value is 0.
Likewise, infinite values also return an intuitive result:
>>> import math
>>> abs(math.inf)
inf
>>> abs(-math.inf)
inf
Infinity is a number that you can access by importing the math
module, an extension of the Python standard library that lets you perform more advanced mathematical calculations. Here, you see inf
returned as the absolute value of both positive and negative infinity, which are both an infinite distance away from zero on the theoretical number line.
Absolute Value of a List of Numbers
Recall that x
must be an item with a numeric data type. You'll raise an error if you pass in an inappropriate argument.
For example, passing in a list of numbers will result in a TypeError
:
>>> abs([-13, -23.93, 5-10j])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: bad operand type for abs(): 'list'
The error message points out that abs() is looking for an operand of a valid datatype - that is, a data type on which a mathematical operation can be performed.
If you'd like to find the absolute value of a list of numbers, you can use the map
function instead:
>>> num_list = [-13, -23.93, 5-10j]
>>> map(abs, num_list)
<map object at 0x7f04556abf10>
>>> print(list(map(abs, num_list)))
[13, 23.93, 11.180339887498949]
This allows your code to access each element of the list directly and pass a single operand at a time to abs(). Once the map object has been created, you can coerce it to a list in order to print the results.
Overview of the fabs function
Python has one other function that you can use to find the absolute value of a number. It's located in the math
module, so you'll need to import math
before you can access it:
>>> import math
>>> # Integer
>>> math.fabs(19)
19.0
>>>
>>> # Float
>>> math.fabs(-25.9)
25.9
>>>
>>> # Bool
>>> math.fabs(True)
1.0
This code block shows how to use fabs()
on each of the numeric data types seen previously. fabs() will coerce the results to a float, which is useful if your program requires the result to be a floating point number.
Note that as a result, fabs() will not work on data types that cannot be coerced to floats:
>>> # Complex
>>> math.fabs(3+2i)
File "<stdin>", line 1
math.fabs(3+2i)
^
SyntaxError: invalid syntax
>>> math.fabs(3+2j)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: can't convert complex to float
Here, you raise a TypeError
when trying to pass in a complex number to fabs(). For complex numbers, its best to stick with the built-in Python absolute value function.
Summary
In this article, you learned how to use abs()
to find the absolute value of integers, floating points, special numbers, and complex numbers in Python. You also took a look at the fabs()
function from the math module, which is a useful alternative for when your output is required to be of the floating point data type.
Next Steps
Python comes prepackaged with several functions you can use to perform basic mathematical operations. For instance, you can find the sum or the minimum value in a list of numbers.
The fabs()
function is accessible via the math module, which contains various other additional functions for advanced mathematical calculations, like the floor function.
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers