Replace Character in String Python | Python String Replace()
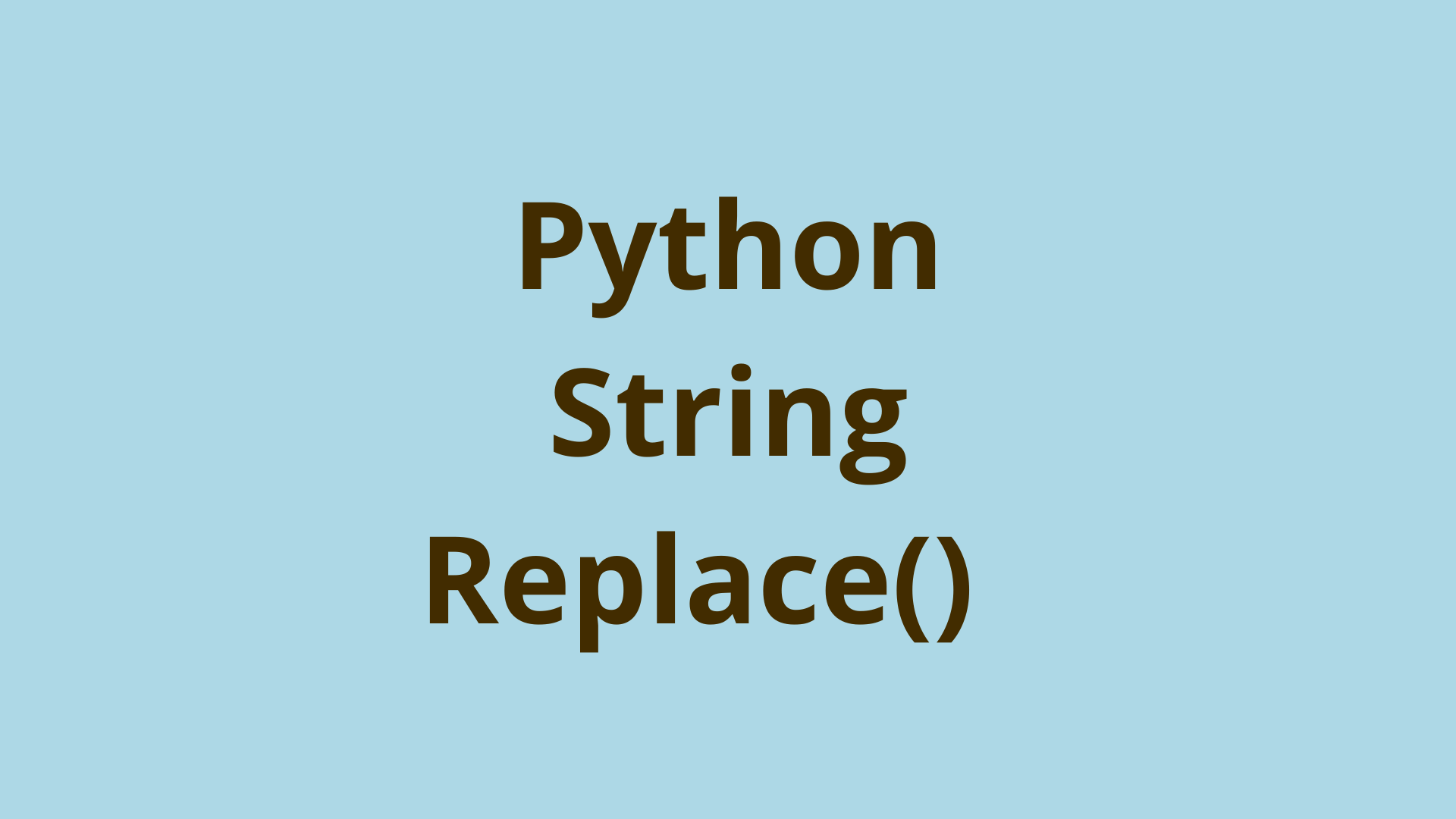
ADVERTISEMENT
Table of Contents
- Introduction
- What are strings?
- How do you replace a character in a string in Python?
- How do you replace a character in a string in Python without using replace method?
- How do you replace multiple characters in a string in Python?
- Summary
- Next Steps
- References
Introduction
Strings are one of the most essential data types in Programming, and one that every developer should know. There are various ways to manipulate python strings, and there are many functions and methods that allow you to do so with ease.
In this article, you will learn how to string replace in a python string using the replace() method and build your own replace function.
What are strings?
A python string is a collection of characters, and a character is a single letter or symbol. For example: 'a'
or '%'
. In Python, characters are also strings.
Strings are just like arrays/lists in Python, but they are immutable. So manipulating strings always costs extra space.
Strings also follow zero-based indexing like arrays, which allow us to get specific characters from the string. For example:
>>> s = "apple"
>>> s[0]
'a'
>>> s[2]
'p'
>>>
How do you replace a character in a string in Python?
The replace()
string method allows us to replace all occurrences of a specific character old in a python string with another character new. The syntax for replace() is:
string.replace(old, new, count)
Let's now look at an example using the replace() method.
>>> s = "apple"
>>> s.replace('a', 'Z')
'Zpple'
>>> s.replace('p', 'W')
'aWWle'
>>> s
'apple'
>>>
Here, we have a python string s. First, we replace the character 'a' with 'Z', and next, we replace 'p' with 'W', which changes all occurrences of 'p' with 'W'. Notice, how none of the operations alter the original string but produce a new string with the alterations.
By default, the replace() method replaces all occurrences of a character, but using the optional count parameter, we can choose how many occurrences of the character we want to replace. The count parameter would replace a certain number of characters starting from the start of the replacement string.
>>> s.replace('p', 'W', 1)
'aWple'
>>> s1 = "appppple"
>>> s2 = "appapple"
>>>
>>> s1.replace('p', 'W', 3)
'aWWWpple'
>>> s2.replace('p', 'W', 3)
'aWWaWple'
Here, you can see the output of the count parameter for different types of strings.
How do you replace a character in a string in Python without using replace method?
If you don't want to use the replace method, we can easily build our own Python function to replace a character in a string.
An efficient approach would be to go through every character in the python string and replace it with the new character.
The function would look like this:
def myReplace(string, old, new):
newStr = list(string)
for i in range(len(newStr)):
if newStr[i] == old:
newStr[i] = new
return "".join(newStr)
>>> myReplace('apple', 'p', 'W')
'aWWle'
Here, we first convert the string into an array, since a string is immutable and we want to be able to change the characters easily. Next, we iterate through every index in the string, and if the character at that index equals to the old character, we replace it with the new character. Finally, we convert our array back into a string using the join method. This is a very efficient approach that takes O(n) time complexity and O(n) space complexity.
However, we can further add the count parameter to this function, like in the original replace() method. We can simply count how many characters we replaced and stop once we replace count characters.
def myReplace(string, old, new, count=float('inf')):
newStr = list(string)
for i in range(len(newStr)):
if newStr[i] == old:
newStr[i] = new
count -= 1
if count == 0:
break
return "".join(newStr)
>>> myReplace('apple', 'p', 'W')
'aWWle'
>>> myReplace('apple', 'p', 'W', 1)
'aWple'
>>> myReplace('apple', 'p', 'W', 2)
'aWWle'
>>> myReplace('apple', 'p', 'W', 5)
'aWWle'
>>>
By default we keep the count as infinity, so all characters are replaced if no count is given. Every, time the old character is found count decrements by 1. When count reaches 0, the loop breaks, making sure no further characters are changed. The efficiency of this approach is the same as the other.
How do you replace multiple characters in a string in Python?
The replace() method even allows us to replace entire substrings with another substring in a string. Let us look at some examples:
>>> s = 'abcdef'
>>> s.replace('bcd', 'ghik')
'aghikef'
>>>
>>> s = 'abcdefgbcd'
>>> s.replace('bcd', 'ghik')
'aghikefgghik'
>>> s.replace('bcd', 'ghik', 1)
'aghikefgbcd'
>>>
Here, we have a string s, and the replace method is able to replace the substring 'bcd' with 'ghik', which returns a new string. Then we do the same with another string, but 'bcd' occurs twice in the string, so both occurrences are replaced. Finally, using the count parameter, we can only replace the first occurrence of 'bcd'.
Replacing substrings follows the same concept as replacing single characters
Summary
In this article, you learned how to replace characters in a string in Python using the replace() method and by building your own efficient replace function.
First, you learned what a string is, what characters are in Python and how strings are very much similar to arrays. Next, you learned what the replace() method does, its syntax, its parameters, and its default behavior which you can change using the count parameter. Then you understood how you can implement your own replace function, using a simple for loop, and then you learned how we can even add the count feature to our function to make it identical to the original replace(). Finally, you learned that not only can the replace() method replace characters in a string, but it can even replace entire substrings with another substring, just as easily.
Next Steps
To learn more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Python Docs - https://docs.python.org/3/library/stdtypes.html