Writing a Python While Loop with Multiple Conditions
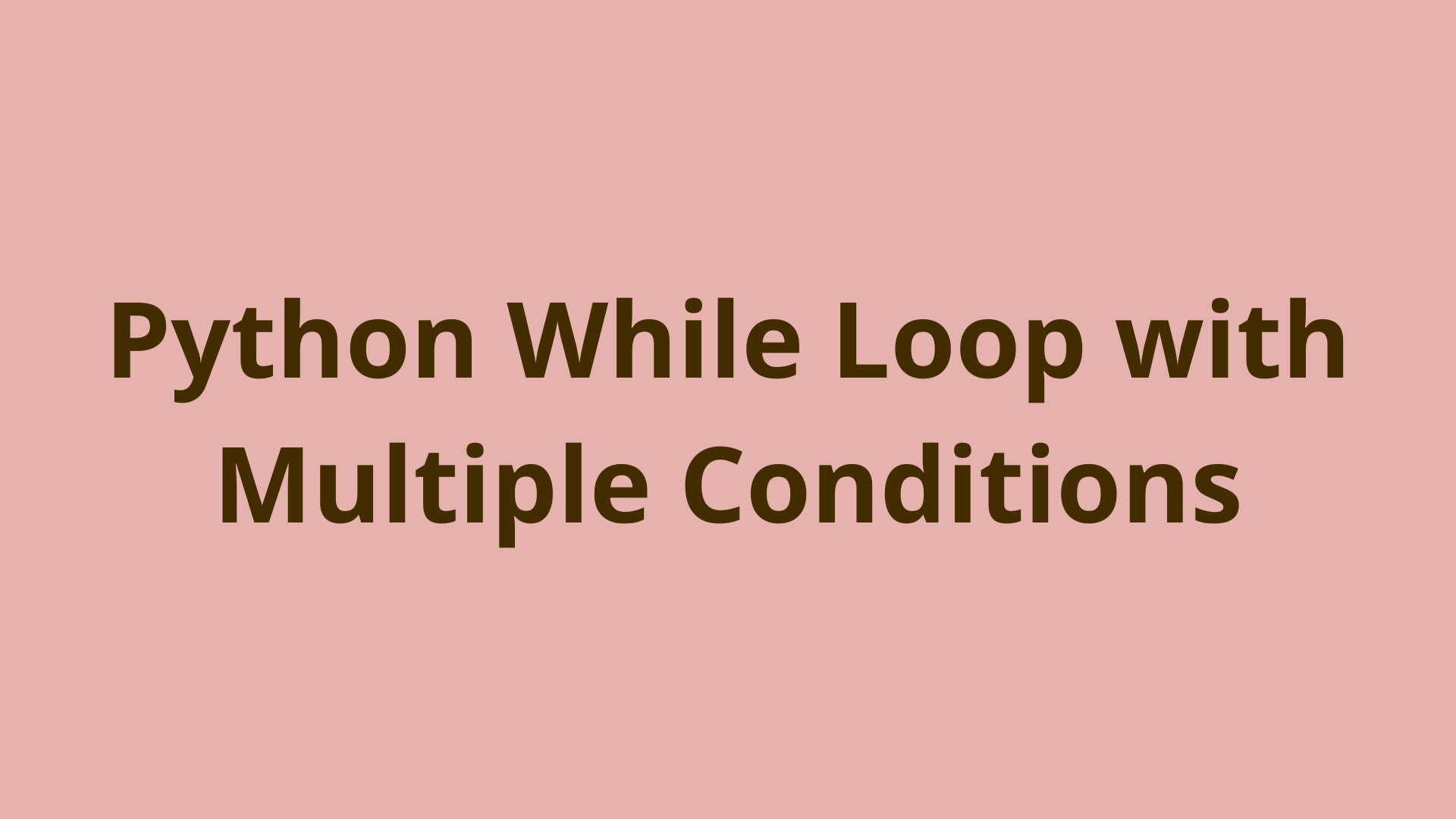
ADVERTISEMENT
Table of Contents
- Introduction
- Review of Python While Loops
- Python While Loop with One Condition
- Python While Loop Multiple Conditions
- Logical OR Operator
- Evaluating Multiple Conditions
- Grouping Multiple Conditions
- Summary
- Next Steps
Introduction
Iteration is the process by which a program repeats a series of steps a certain number of times or while a certain condition is met. These two forms of iteration are known as definite (often implemented using a for
loop and Python next function) and indefinite (often implemented using a while
loop).
In this article, you'll take a more advanced look at indefinite iteration in Python. More specifically, you'll learn how to write a Python while loop with multiple conditions. Let's get started!
Review of Python While Loops
In Python, indefinite iteration generally takes the following form:
while ( CONDITIONAL EXPRESSION ):
EXECUTE STATEMENTS
You initiate the loop with the while
keyword, then set the condition to be any conditional expression. A conditional expression is a statement that evaluates to True
or False
. As long as the condition is true, the loop body (the indented block that follows) will execute any statements it contains. As soon as the condition evaluates to false, the loop terminates and no more statements will be executed.
The simplest while loops in Python are while True
and while False
:
>>> while True:
... print("I'll print forever")
...
I'll print forever
I'll print forever
I'll print forever
I'll print forever
I'll print forever
...
In this case, the condition is always True, so the loop will run forever. In order to stop it, you'll need to close the interpreter or send Python a KeyboardInterrupt
by pressing CTRL+C.
A "while True" loop is always true and runs forever. A "while False" loop, on the other hand, is never true:
>>> while False:
... print("I'll never run")
...
>>>
Since the condition is never true to begin with, the loop body never executes, and no statement is printed.
Python While Loop with One Condition
One of the most common uses of while loops in Python is to execute a series of steps a specific number of times. Often, you'll use a “counter” variable to control how many times the loop executes. For example:
>>> count = 5
>>> while count > 0:
... print("Current value of count: ", count)
... count -= 1
...
Current value of count: 5
Current value of count: 4
Current value of count: 3
Current value of count: 2
Current value of count: 1
>>>
Here, you define a counter and set it to the number 5. As long as the counter is greater than zero, you want the loop body to execute, so your conditional expression is count > 0
. The output shows that the loop body successfully executes for the five iterations where the value of count is greater than zero. Each iteration prints the current value of count, then decrements count by one. On the final iteration, count is now equal to zero, and the conditional expression is now false. The while loop terminates, and the loop body stops execution.
In this example, you know the value of the counter ahead of time. However, while loops are a form of indefinite iteration, and that usually means you won't know how many times the loop body should execute beforehand. You may want to change this value depending on the goals of your code, how many records are in your database, or how soon you want your machine learning algorithm to converge. The possibilities are endless, but the underlying while loop structure is much the same.
Let's define a variable max_iterations
to simulate an unknown number of iterations:
>>> import random
>>> max_iterations = random.randint(0,10)
The Python random module has a method .randint()
that allows you to choose any random integer between two numbers. Here, you set the max number of iterations to a random number between 0 and 10.
You don't know what the number is, but you can use a while loop to find out. To do this, set your counter to 0. The conditional expression will be "while the value of the counter is less than the maximum number of iterations." Here's what that looks like in code:
>>> count = 0
>>> while count < max_iterations:
... print(f"count is {count}. max_iterations is {max_iterations}")
... print(f"Incrementing count by 1.")
... count += 1
...
count is 0. max_iterations is 4
Incrementing count by 1.
count is 1. max_iterations is 4
Incrementing count by 1.
count is 2. max_iterations is 4
Incrementing count by 1.
count is 3. max_iterations is 4
Incrementing count by 1.
On each iteration, Python will check to see if the current value of count
is less than the maximum number of iterations. If this is true, then the loop body executes. You can see here that each iteration displays the value of both variables and increments the counter by one. On the last iteration, where count is equal to max_iterations
, the conditional expression becomes false and the loop terminates.
You can verify the value of max_iterations
like so:
>>> max_iterations
4
So far, you've seen how Python while loops work with one conditional expression. Python compares the two values and if the expression evaluates to true, it executes the loop body. However, it's possible to combine multiple conditional expressions in a while loop as well.
Python While Loop Multiple Conditions
To combine two conditional expressions into one while loop, you'll need to use logical operators. This tells Python how you want all of your conditional expressions to be evaluated as a whole.
Logical AND Operator
The first logical operator is and
. Here's how you would use it to combine multiple conditional expressions:
while ( CONDITIONAL EXPRESSION A ) and ( CONDITIONAL EXPRESSION B ):
EXECUTE STATEMENTS
Here, the while loop has two conditional expressions, A and B, that it needs to evaluate. The and
operator says to evaluate them separately and then consider their results as a whole. If (and only if) both A and B are true, then the loop body will execute. If even one of them is false, then the entire while statement evaluates to false and the loop terminates.
Let's take a look at a code example.
>>> a = 5
>>> b = 10
>>> count = 0
>>> while count < a and count < b:
... print(f"count: {count}, a: {a}, b: {b}")
... count += 1
...
count: 0, a: 5, b: 10
count: 1, a: 5, b: 10
count: 2, a: 5, b: 10
count: 3, a: 5, b: 10
count: 4, a: 5, b: 10
You define two new variables, a
and b
, which are equal to 5 and 10, respectively. Again, you've defined a counter and set it to zero. The while statement now contains two conditional expressions. The first checks to see if count is less than a
, and the second checks to see if count is less than b
. The logical operator and
combines these two conditional expressions so that the loop body will only execute if both are true.
The loop runs for five iterations, incrementing count by 1 each time. In the last iteration, where the loop terminates, the value of count is now 5, which is the same value as a
. This means the first conditional expression is now false (5 is not less than 5), so the while loop terminates.
Note that the second condition count < b
is still true, even when count is 5. What if you wanted the loop to keep running as long as count is less than one of the variables?
Logical OR Operator
Logical or
is the second operator you can use to combine multiple conditional expressions:
while ( CONDITIONAL EXPRESSION A ) or ( CONDITIONAL EXPRESSION B ):
EXECUTE STATEMENTS
There are still two conditional expressions, A and B, that need to be evaluated. Like logical AND, the or
operator says to evaluate them separately and then consider their results as a whole. Now, however, the loop body will execute if at least one of the conditional expressions is true. The loop will only terminate if both expressions are false.
Let's take a look at the previous example. The only change is that the while loop now says or
instead:
>>> a = 5
>>> b = 10
>>> count = 0
>>> while count < a or count < b:
... print(f"count: {count}, a: {a}, b: {b}")
... count += 1
...
count: 0, a: 5, b: 10
count: 1, a: 5, b: 10
count: 2, a: 5, b: 10
count: 3, a: 5, b: 10
count: 4, a: 5, b: 10
count: 5, a: 5, b: 10
count: 6, a: 5, b: 10
count: 7, a: 5, b: 10
count: 8, a: 5, b: 10
count: 9, a: 5, b: 10
Now, the loop doesn't stop after the count
variable reaches the same value as a
. That's because the second condition, count < b
, still holds true. Since at least one condition is true, the loop body continues to execute until count reaches a value of 10. Then, the second condition evaluates to false as well, and the loop terminates.
Logical NOT Operator
There's one other logical operator that you can use to write Python while loops with multiple conditions, and that's logical not
:
while ( not CONDITIONAL EXPRESSION ):
EXECUTE STATEMENTS
This operator simply reverses the value of a given boolean expression. In other words, not True
will return false, and not False
will return true.
Evaluating Multiple Conditions
Let's add a third conditional expression to the code. Earlier, you defined the random variable max_iterations
to control the execution of the loop body. Define this again to set a new random number and add it to the while statement:
>>> a = 5
>>> b = 10
>>> max_iterations = random.randint(0,10)
>>>
>>> count = 0
>>> while count < a or count < b and not count >= max_iterations:
... print(f"count: {count}, a: {a}, b: {b}")
... count += 1
...
count: 0, a: 5, b: 10
count: 1, a: 5, b: 10
count: 2, a: 5, b: 10
count: 3, a: 5, b: 10
count: 4, a: 5, b: 10
count: 5, a: 5, b: 10
count: 6, a: 5, b: 10
count: 7, a: 5, b: 10
This Python while loop has multiple conditions that all need to be evaluated together. The first condition checks whether count is less than a
. The second condition checks whether count is less than b
. The third condition uses the logical not
operator to check that the value of count has not reached the maximum number of iterations. Python has set this value to a random number (here, max_iterations
was 8). So, the loop runs for eight iterations and then terminates - but which condition caused this?
Python evaluates conditional expressions from left to right, comparing two expressions at a time. One can use parentheses to better visualize how this works:
while ( CONDITIONAL EXPRESSION A or CONDITIONAL EXPRESSION B ) and not CONDITIONAL EXPRESSION C
Python groups the first two conditional expressions and evaluates them together. In the code block above, count is compared to a
and then to b
. The logical or
operator that combines these two statements says to return true if either one of them is true. This is the case for all eight iterations of the loop (count
is always less than a
or b
).
Next, Python combines the result of the first evaluation with the third condition:
while ( RESULT and not CONDITIONAL EXPRESSION C )
Here, RESULT
will either be true or false. Python uses the logical and
operator to combine this with the third condition. If both are true, then the loop body will continue to execute. If one is false, then the loop will terminate.
Truth tables are a great tool to help visualize how a Python while loop with multiple conditions will evaluate each one.
Note that the loop terminates on the ninth iteration. This is something you may not see in your terminal output. That's because the ninth iteration causes the while statement to evaluate to false. Here, count is still less than a
or b
, but the maximum number of iterations has been reached. Even though the second condition is still true, the logical and
requires the third condition to be true as well. Since it's false, the loop body terminates.
Grouping Multiple Conditions
Parentheses can help you to see how Python evaluates while loops with multiple conditions, but they can also be used to control how Python evaluates multiple conditional expressions.
Recall the previous example:
while ( CONDITIONAL EXPRESSION A or CONDITIONAL EXPRESSION B ) and not CONDITIONAL EXPRESSION C
Here, you used parentheses to visualize how Python evaluates conditional expressions from left to right. You can also use parentheses directly in your code to tell Python which expressions should be considered together first.
Let's set max_iterations
to a smaller number to see this in action. Set it to 3 and start the while loop again, grouping the first two expressions in parentheses:
>>> max_iterations = 3
>>> count = 0
>>> while (count < a or count < b) and not count >= max_iterations:
... print(f"count: {count}, a: {a}, b: {b}")
... count+=1
...
count: 0, a: 5, b: 10
count: 1, a: 5, b: 10
count: 2, a: 5, b: 10
The parentheses tells Python to group the first two statements together, then to combine their result with whatever statement comes next. You've now reduced the multiple conditions into two groups: "count is less than a or b AND count is not greater than or equal to max_iterations." This statement evaluates to false after the third iteration, so the loop stops here.
What happens if you move the parentheses and group the last two statements together? Let's take a look:
>>> max_iterations = 3
>>> count = 0
>>> while count < a or (count < b and not count >= max_iterations):
... print(f"count: {count}, a: {a}, b: {b}")
... count+=1
...
count: 0, a: 5, b: 10
count: 1, a: 5, b: 10
count: 2, a: 5, b: 10
count: 3, a: 5, b: 10
count: 4, a: 5, b: 10
Here, the loop runs for 5 iterations, which is far above the maximum number of 3. What happened?
Python still evaluates the conditional expressions from left to right. First, it checks to see whether count is less than a
. If this is true, Python then moves to the next statement, which is a group of two conditional expressions. Python then considers this group as a whole, and combines the result with the first conditional statement. The multiple conditions are again reduced into two groups: "count is less than a OR count is less than b and not greater than or equal to max_iterations." When count is 3 - the maximum number of iterations - the expression still evaluates to true due to the logical or
. The loop runs until both the expression before the logical or
and the grouped expressions after it cause the while statement to evaluate to false.
Summary
In this article, you learned how to write a Python while loop with multiple conditions.
You reviewed indefinite iteration and how while statements evaluate conditional expressions. Then, you saw how to use logical operators to combine multiple conditions in while statement. Lastly, you looked at how grouping multiple conditions together in parentheses can help both to visualize statement evaluation and control loop body execution.
Next Steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Indefinite iteration is one of the first techniques you'll learn on your Python journey. For other beginning Python tutorials, check out our guides on functions in Python 3, the Python min() function, and the Python floor() function.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers