Convert Int to String Java
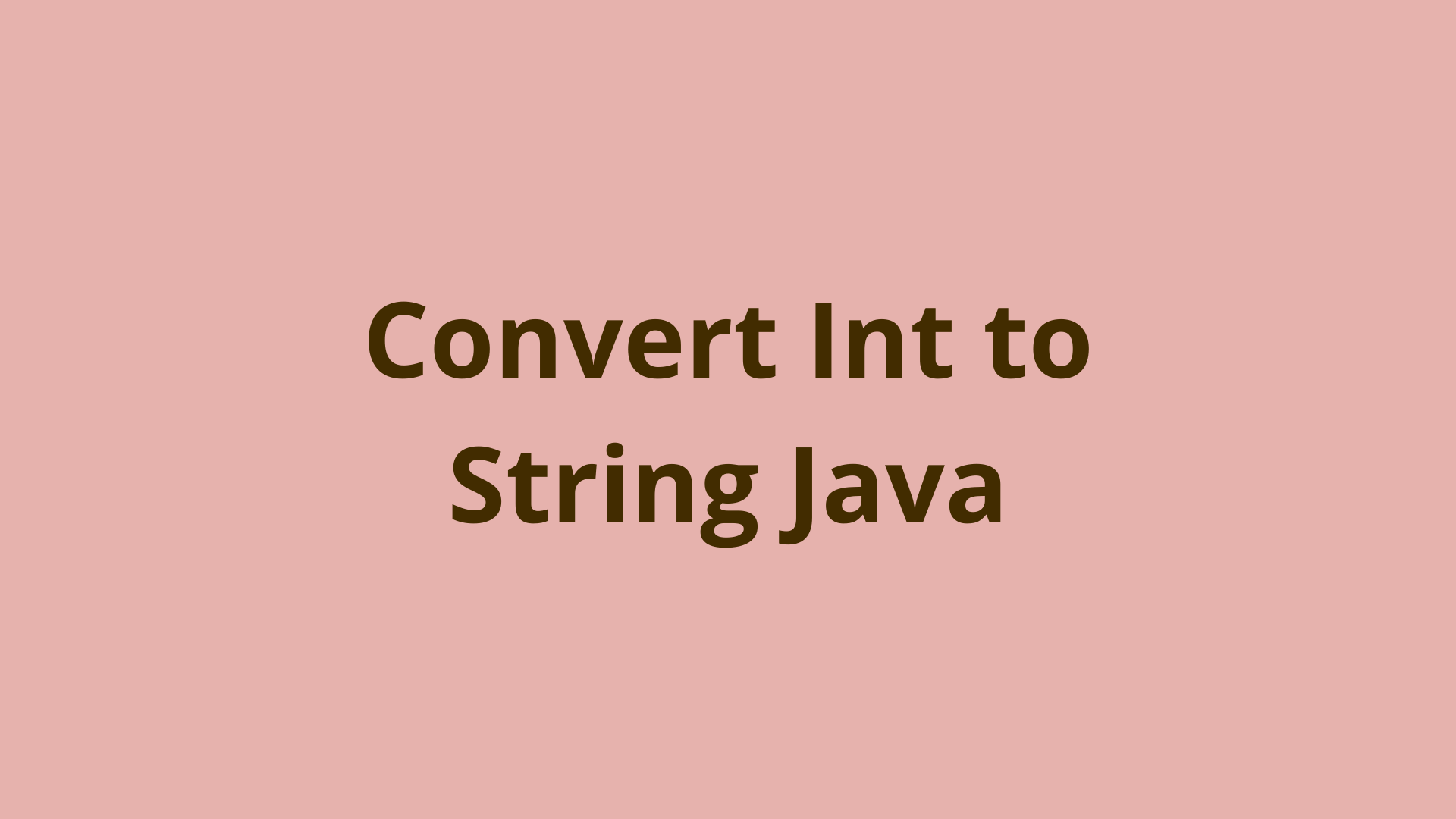
ADVERTISEMENT
Table of Contents
- Introduction
- 1) String.valueOf()
- 2) .toString()
- 3) Concatenating an empty string
- 4) String.Format()
- 5) StringBuilder()
- Summary
- Next Steps
Introduction
When working with numerical values such as integers, floats, doubles, and longs in Java, it's often necessary to convert them to strings. For example, you might need to include a number in a formatted string such as a debug message or a text field.
In this article, we'll discuss several methods to convert int to string in Java, and as a bonus we'll touch on conversion from other numeric data types as well.
1) String.valueOf()
Using the String.valueOf()
method, developers can get the string value of various numeric primitive types and objects. Primitive types include int
, float
, double
, and long
. Object types such as Integer
, Float
, Double
, and Long
are also supported. It also provides protection against calls on null objects. For example, if String.valueOf(x)
is called on a variable that has not been initialized to a value, the function returns a null string instead of throwing a null pointer exception. Additionally, since this method supports a variety of object types, this method is flexible enough to be easily applied to various use cases.
In the following example, the String.valueOf()
method is used to convert the variable x
(of primitive type 'int') and y
(of numeric object type Integer
), into the string "365":
public class Converter {
public static void main(String []args){
int x = 365;
String x_as_str = String.valueOf(x);
System.out.println(x_as_str);
Integer y = 365;
String y_as_str = String.valueOf(y);
System.out.println(y_as_str);
}
}
2) .toString()
Developers can also use the Integer.toString()
method to convert integer to string. The static Integer.toString()
method belongs to the Integer class and can be used by passing the integer to be converted as an argument: Integer.toString(3)
. Other classes offer similar functionality, and the namespace must match the argument type. For example, a float can be converted to string using the method Float.toString(3.6f)
.
This method can also be called directly on objects that support it. This includes all primitive types including integers, floats, doubles, and longs. With this approach, developers can call x.toString()
without having to specify the namespace or provide any arguments.
Unlike the String.valueOf()
approach, this method does not provide protection against calls performed on null objects. A call of Integer.toString(x)
or x.toString()
on a variable x
that has not yet been set will throw a null pointer exception.
In the following example, we convert the integer 365 into a string:
public class Converter {
public static void main(String []args){
int x = 365;
// Method 1: Using the static method
String x_as_str = Integer.toString(x);
System.out.println(x_as_str);
// Method 2: Calling the variable's toString() method
String x_as_str = x.toString();
System.out.println(x_as_str);
}
}
This examples works similarly for other primitive types. Here, we show a similar method applied to convert a double into a string:
public class Converter {
public static void main(String []args){
double x = 3.0;
// Method 1: Using the static method
String x_as_str = Double.toString(x);
System.out.println(x_as_str);
// Method 2: Calling the variable's toString() method
String x_as_str = x.toString();
System.out.println(x_as_str);
}
}
3) Concatenating an empty string
A quick and dirty way to convert a number to string in Java is to simply concatenate the number to an empty string using the +
operator. In addition to converting an int to string, this approach can also be used as a quick way to build longer strings from the original integer.
Here, we convert the integer 365 to a string, and then generate a longer string, "The integer x is 365."
public class Converter {
public static void main(String []args){
int x = 365;
String x_as_str = "" + x;
System.out.println(x_as_str);
String x_extended = "The integer x is " + x;
System.out.println(x_extended);
}
}
To do this conversion using this method, Java actually invokes StringBuilder
(as of Java 7) behind the scenes, which we will discuss in a minute. This added overhead reduces performance slightly, but is likely not an issue for most programs.
4) String.Format()
The String.Format()
method allows developers to convert int to string in Java with a higher degree of control over how the resulting string is formatted. It also provides a neater way for developers to easily embed an integer within a longer string. There are numerous format specifiers that developers can use to specify how the strings should be formatted. For example, developers can specify how many decimal places should be included in the string and pad the output with numbers or symbols.
This is a great option for developers who require a richer set of features than the previous methods in this list provide. When building longer strings, this technique also provides a safer approach than the previous example of string concatenation. However, for developers in need of only a quick conversion, this method may provide more functionality than they really need.
In the following example, we convert the integer 365 to a string using String.Format()
and then build a longer formatted string:
public class Converter {
public static void main(String []args){
int x = 365;
// %d is a formatting specifier indicating that x is a decimal integer
String x_as_str = String.format("%d", x);
System.out.println(x_as_str);
// This method can also be used to embed an integer within a string
String x_extended = String.format("The integer x is %d", x);
}
}
5) StringBuilder()
As with the previous two approaches, the StringBuilder()
class provides a way to both convert an integer to a string and to build a longer string that contains that integer. Rather than creating a regular String, however, this creates a StringBuilder()
object. Numeric variables can be added to an existing StringBuilder()
object using the .append()
method. By appending an integer to the StringBuilder()
, that integer is converted into a string in the process. This class can also be used to build longer strings by consecutively appending additional objects.
In the following example, we convert the integer 365 to a string and then build a longer string:
public class Converter{
public static void main(String []args){
int x = 365;
StringBuilder sb = new StringBuilder();
sb.append(x);
System.out.println(sb);
StringBuilder sb_extended = new StringBuilder();
sb_extended.append("The integer x is ");
sb_extended.append(x);
System.out.println(sb_extended);
}
}
Like many other objects in Java, a StringBuilder()
can be converted to a string using the .toString()
method, like this:
String sb_to_string = sb_extended.toString();
Summary
In Java, int to string conversion can be achieved using any of the methods described above. For quick conversions, String.valueOf()
tends to be the safest approach since it supports numerous argument types and provides checks for null objects. Integer.toString()
does not provide checks for null objects, but can be especially useful when performing a quick check on defined variable using the syntax x.toString()
. The remaining methods, including string concatenation, String.Format()
, and StringBuilder()
provide more heavy-weight approaches to string conversion. While these approaches can be used to simply convert numerical values into a string, they are typically more useful when developers need a higher degree of control over the format of the string or when they are looking to incorporate a numerical variable into a longer string.
The best approach to converting numerical values to strings in Java depends on a developer's specific use case. With any of these commands, developers can easily convert numerical values to strings in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers