Python List Length
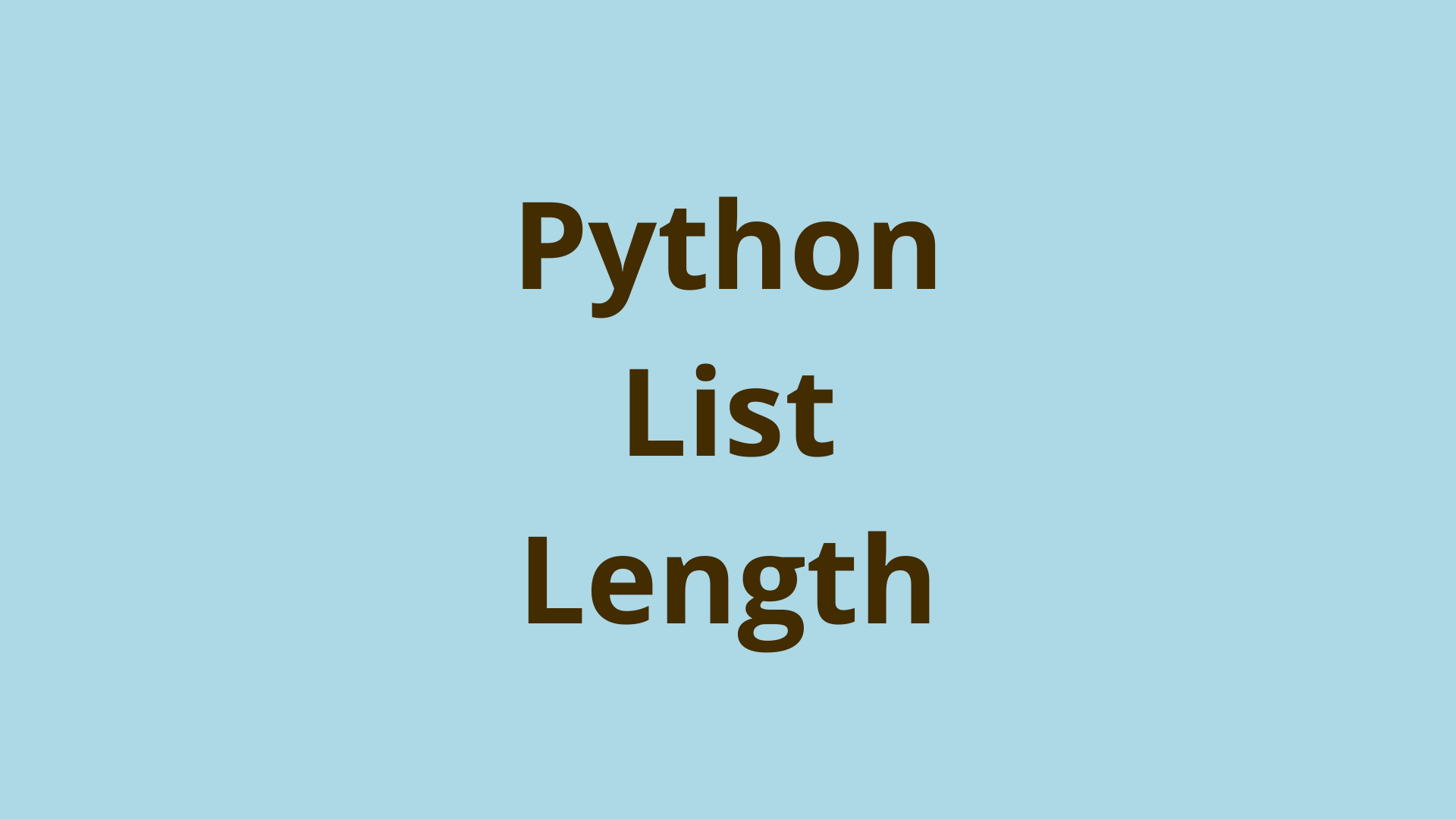
ADVERTISEMENT
Table of Contents
- Introduction
- Python List Length With len()
- Python Length of List With Counter
- Python Length of List With Indexing
- Summary
- Next Steps
Introduction
Python has a number of different data structures that you can use to hold collections of information. The one you're most likely to come across early on in your developer journey is the list, which can dynamically hold any number of elements, even elements of differing data types.
The key phrase here is "any number of." How would you determine, then, exactly what number of items a list holds? In other words, how can you find the length of a Python list?
In this article, you'll take a quick look a few different ways to find the length of a list in Python.
Python List Length With len()
As with most basic operations, there's a built-in function that comes with every installation of Python for list length. The len()
function (which is short for length) has the following syntax:
len(x)
The argument x
can be any of several different types of iterable objects, such as collections (sets and dictionaries) or sequences (such as strings, tuples, or - relevant to this article - lists). The function then returns the number of items in the given object.
To find a Python list's length, you would then use len()
as follows:
>>> my_list = [1, 2, 3, 4, 5]
>>> len(my_list)
5
Here, you define a list my_list
with the integers 1
through 5
as elements. You then pass in your list to len()
, which correctly returns 5
as the number of objects the list currently holds.
Python Length of List With Counter
In the previous example, you defined a list and then used the Python function len()
to return the number of elements in it. However, in several cases you'll want to know the length of a list that doesn't exist yet.
This might be the case whenever you're using iteration. For instance, you may be processing all the rows in a CSV file, updating all the tables in a database, or looping over a code block until an error state is triggered. In each of these scenarios, you'll likely be using a counter to keep track of how many iterations have completed.
You can use the same concept to figure out the length of a list. Let's continue on with my_list
, since we know the true answer and can verify our implementation.
To use a counter, you start with some arbitrary number, usually 0
, and increment this number each time a loop is performed. A for
loop is a good candidate for finding Python list length using counters:
>>> count = 0
>>> for i in my_list:
... count += 1
...
>>>
Here, you initialize a variable count
to zero, then you start a for loop. The loop states that for each element i
in the list, the counter should be incremented by one. When the iterator reaches the end of the list, the counter will stop and return the last number it was incremented to. This should be the total number of items in the list, and since we know the true length of my_list
we can verify that this is indeed the case:
>>> count
5
Python Length of List With Indexing
There's another property of iterable objects that you can exploit to find the length of a Python list. Most iterable objects allow you to locate the index of the desired element. This is the sequential numeric position of the element in the iterable, where the first item is at location 0
, the second is at 1
, and so on.
There's even a built-in method, .index()
, that you can append to the object in question to locate the index of a given element:
>>> letters = ["a", "b", "c", "d"]
>>> letters.index("a")
0
>>> letters.index("d")
3
Here, a new list letters
is defined, with four elements. Calling the index method on the list and passing in the string "a"
shows that it is at index 0
, whereas the string "d"
is at the index 3
.
While this method will return the positive signed integer for the location of the given element, you as the developer can use bracket notation to index the list with a negative signed integer, like so:
>>> letters[-1]
'd'
When you ask for the negative first object in a given list, Python understands that you want it to find the object that's the first item in the list if the list were reversed.
So, what's this got to do with finding the length of a list in Python? Well, negative indexing will give you the index (the numeric position) of the last element in a list:
>>> letters.index(letters[-1])
3
This line of code operates on letters
twice, first calling the index method, and then passing in the negative index for the last element in the list. We know that iterable objects in Python are indexed from zero, so the index of the final element will be one less than the total length of the list. So, what happens if we add one to that number? Let's find out:
>>> letters.index(letters[-1]) + 1
4
With this, you're saying, "Give me the index of the last element in the list; and since I know Python starts counting from zero, add one to that number, which will give the actual length of the list."
You can check and see that this is the case:
>>> len(letters)
4
Note that even if a particular element occurs twice in the same list, the .index()
method will retrieve the first occurrence of that element in the list, which could throw off the calculation. The last example above was shown for informative purposes, but in general you'll usually want to stick with the len()
function.
Summary
In this article, you saw three different methods for determining a list's length in Python. First, you used the built-in function len()
to return the length of a known list. Then, you initialized a counter and returned its maximum value as the equivalent for the length of a list you were iterating over. Finally, you saw how indexing could be exploited to return the length of a list.
Next Steps
Finding the length of a list is one operation that you can perform on this sequence, but there are others. For example, you can find the sum of the elements of a list or even the smallest number in a list just as quickly using other built-in Python functions.
As mentioned in this article, you can use a counter with a for
loop to find the length of a list in Python. You can also use a while
loop to iterate over an object and execute code multiple times. To learn more, check out Writing a Python While Loop With Multiple Conditions.
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers