Python Multiline String
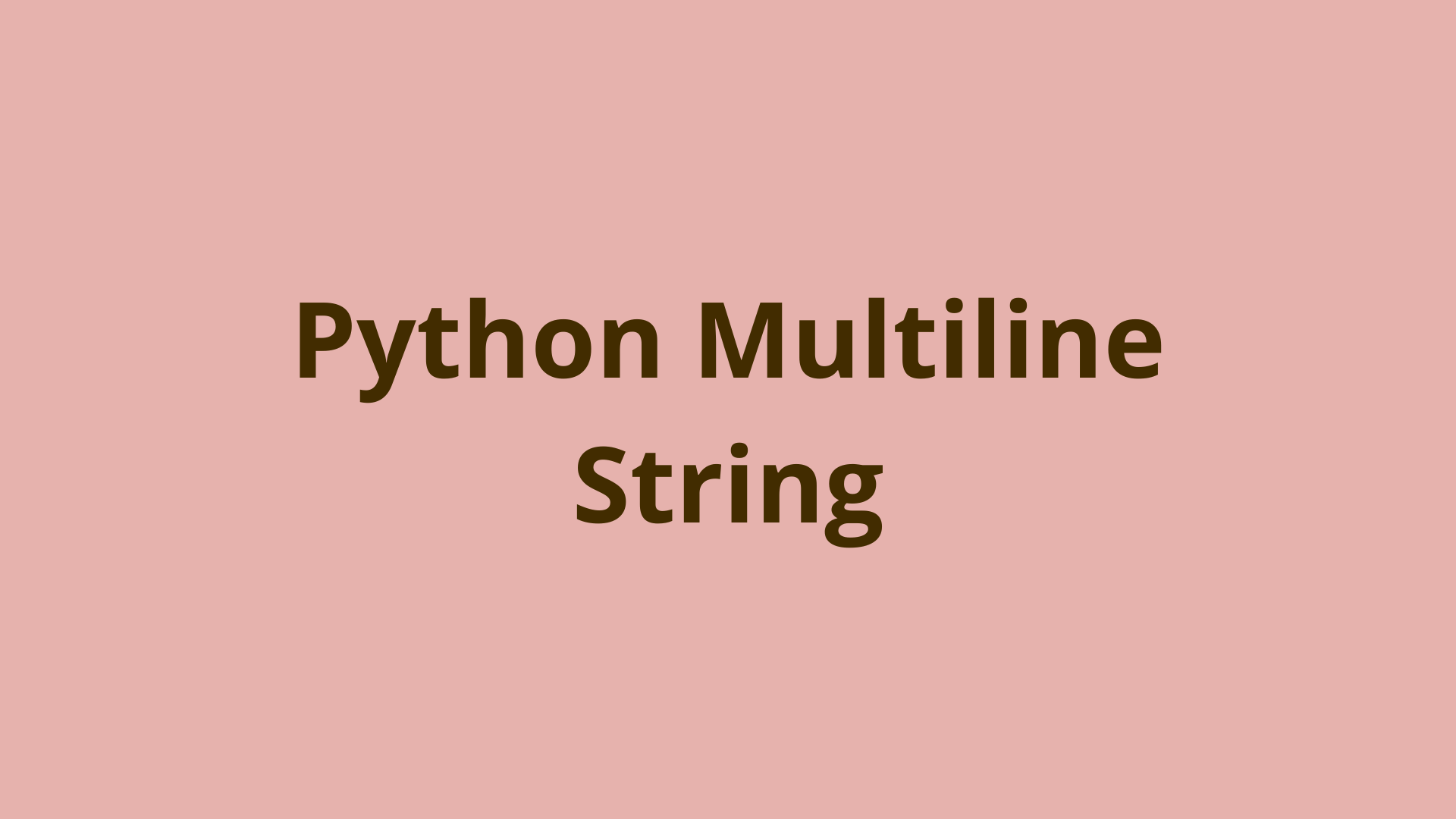
ADVERTISEMENT
Table of Contents
- Introduction
- What is a Python string?
- What is multi line string?
- How do you read a multiline string in Python?
- Multiline strings and examples
- Using only escape character
- Using Triple Quotes
- Multiline string using join() and a list/tuple
- Summary
- Next Steps
- References
Introduction
Strings are used to represent text and are therefore a fundamental datatype in any high level programming language. In Python, there are various ways to manipulate strings using built-in methods and functions. But, what if you wanted to write strings with multiple lines in Python for your program? Well, there are many ways to accomplish this. However, it is important to understand the benefits and drawbacks of each approach and which to use in a particular situation.
In this tutorial, you will learn several ways to define multi-line strings efficiently in Python, while also taking into account code readability.
What is a Python string?
A python string is a collection of characters (alphabets, numbers, spaces, etc.). In Python, single line strings are enclosed in either single quotes ('') or double quotes (""), and they both work in the same way. Strings are very similar to lists (arrays), however they both have different representations
strings are immutable while arrays are mutable
For example:
x = 'string #1'
y = "string2 string2"
With strings you can also include escape characters. Escape characters are used to define special characters and deal with specific cases. In Python escape characters should follow a backslash ().
For example, you can use escape characters to use double quotes inside a double-quoted string:
>>> x = "A story called \"The Sky and the Sea.\""
>>> print(x)
A story called "The Sky and the Sea."
The backslash tells Python that the quote following it is part of the string. Of course, you can also do the same thing with a single quote as well. There are also several other escape characters you can use
newline (\n), tab (\t), backspace(\b)
The '\n' escape character is used to define a newline (which is what we will be mostly using in this article). For example:
>>> x = "line1\nline2"
>>> print(x)
line1
line2
What is multi line string?
In python strings are a strings that contains several lines, rather than just one line. There are several ways to create a multiline string in python. Python provides a wide variety of ways to format strings to your liking.
How do you read a multiline string in Python?
Reading multi-line strings in Python is similar to reading single line strings. There are multiple ways to make multi-line strings in Python, and the output of each is the same, code readability is our main concern here.
Multiline strings and examples
Let's look at several ways you can create a python multiline string with some useful examples.
Using only escape character
As showcased in the previous section, you can use the '\n' escape character to get a new line character and separate the string into multiple lines. For example:
>>> x = "abc\ndef\nghi"
>>> print(x)
abc
def
ghi
>>>
This is a very simple approach and could be great for simple 2-3 line strings. But, if you want to have more lines, this approach would result in very unclean code like this:
>>> x = 'abcde\nfghij\nklmno\npqrst\nuvwxy\nzabcd\nefghi\njklmn\nopqrs\ntuvwx\nyzabc\ndefgh\nijklm\nnopqr\nstuvw\nxyz'
>>> print(x)
abcde
fghij
klmno
pqrst
uvwxy
zabcd
efghi
jklmn
opqrs
tuvwx
yzabc
defgh
ijklm
nopqr
stuvw
xyz
You would end up with endless long horizontal lines of string.
Using Parentheses
You can also use parenthesis to separate the lines in the code. For example:
>>> x = ("abc\n"
... "efg")
>>> print(x)
abc
efg
>>>
The parenthesis allows you to move to the next line, and align the lines to make the string more readable. Overall, this has the same effect as concatenating the strings like: 'abc\n' + 'efg'
. Notice, that the newline escape character is still needed here.
Using backslash
Similar to the last approach, you can use also backlash (outside the string) to separate the lines of code:
>>> x = "abc\n" \
... "efg\n" \
... "hij"
>>>
>>> x
'abc\nefg\nhij'
>>> print(x)
abc
efg
hij
The backlash in Python (outside the string) allows you to continue your line of code into the next line.
The main drawback of this approach is that you have to add the backslash to the end of every line in addition to the '\n' character, which can get very tedious.
Using Triple Quotes
Python provides a default way to write multi-line strings: using triple quotes. Although it is very simple to use, it still has its drawbacks. First, let's look at an example:
>>> x = """abc
... def
... hij"""
>>> print(x)
abc
def
hij
Note, that you can use single or double quotes here. Starting from the first triple quote, everything inside is treated as a string, including the next lines, until you close with triple quotes.
However, there are times when this could hinder code readability. For example, if you have many indents, and you want to use this approach, you cannot align the string with the rest of your code since the indents will also count as part of the string like so:
>>> def f():
... x = """abc
... def
... ghi"""
... print(x)
...
>>> f()
abc
def
ghi
>>>
This can lead to messy code, especially when you have multiple indents. This method is best used when you want to create a top-level comment.
Multiline string using join() and a list/tuple
There is another method you can use which would solve many of the drawbacks encountered with other approaches.
In this approach, you can first store the multiline string in a list or tuple, as a list of lines. Then you can join all the lines with the newline character and the join() string method. For example:
>>> x = ('abcd',
... 'efghi',
... 'jklmn')
>>> print('\n'.join(x))
abcd
efghi
jklmn
Here, the lines are stored in the tuple x, which are then joined using the join() method and the newline character. The join() method joins all the elements in a list or tuple with some string between them. This way you can indent the code and, unlike the triple quote method, it is much easier to separate each line with a comma than with a '\n' at the end of every string.
Overall, this way is much more Pythonic and makes the code very readable.
Summary
In this article, you learned how to define python multiline strings and the pros/cons of each approach.
First, you briefly learned what strings are and how they are defined in Python. Then, you learned what escape characters are and how to use some common ones. Next, you dived into the different approaches to defining multi-line strings and understood our focus on code readability. You learned the simplest approach and quickly understood its drawback. Next, you learned how we can separate lines of strings in the code using either parentheses or backslashes. Then, you learned Python's default way of defining multi-line strings and its drawbacks. Finally, you looked at the approach using a list/tuple and the join() method, which solves many of the drawbacks we encountered with the previous approaches.
Next Steps
To learn more about the basics of Python, tutorials, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this tutorial. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Python Escape Characters - https://www.w3schools.com/python/gloss_python_escape_characters.asp
- Python Strings - https://developers.google.com/edu/python/strings
- Triple Quotes in Python - https://www.geeksforgeeks.org/triple-quotes-in-python/
Final Notes
Recommended product: Coding Essentials Guidebook for Developers