Python List Print
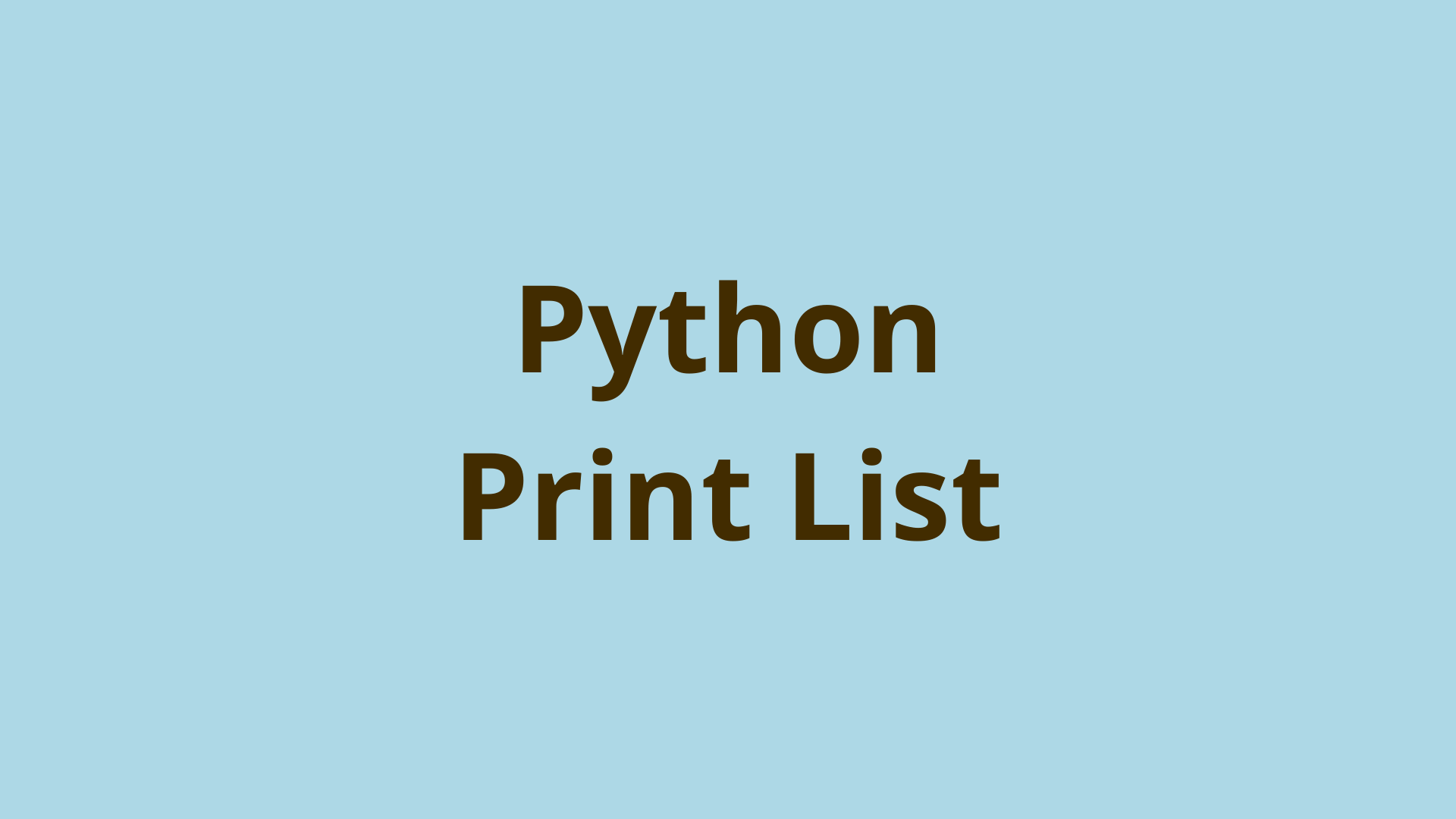
ADVERTISEMENT
Table of Contents
- Introduction
- Python print list with the
print()
function - Print list with loops
- Print list with join() function
- Print list in reverse
- Summary
- Next Steps
Introduction
Arrays - sometimes known as lists - are a basic, but powerful data structure present in almost all programming languages. They make it easy to store ordered data, which then allows for fast and easy to access that data using a numeric index. In Python, arrays are referred to as lists, and they are known for being easier to use in Python compared to other programming languages.
Efficiently printing all the data elements stored in a list can be a very useful, if not essential task for certain workflows.
In this article, we will explore various ways to print lists in Python along with the benefits of each.
Python print list with the print()
function
The simplest way of printing a list is directly with the print()
function:
>>> x = [1, 2, 3, 4]
>>> print(x)
[1, 2, 3, 4]
This method prints the raw Python list syntax (including the brackets and commas), but you can use the *
symbol to print the list much more elegantly. For example:
>>> x = [1, 2, 3, 4]
>>> print(*x)
1 2 3 4
>>>
Here, only the items in the list are printed in order with a space separating them.
This simple method of printing lists seems to work fine, so one might wonder why not just use this method to print lists all the time. This method works well for small, simple lists like the one shown above. But if you were to use a list with larger and more complex data, it quickly becomes difficult to comprehend the output.
For example, let's say you have a list with a bunch of lengthy strings:
>>> longStrings = ['first long string first long string first long string first long string first long string first long string first long string first long string first long string first long string ', 'second long string second long string second long string second long string second long string second long string second long string second long string second long string second long string ', 'third long string third long string third long string third long string third long string third long string third long string third long string third long string third long string ']
>>>
>>> print(longStrings)
['first long string first long string first long string first long string first long string first long string first long string first long string first long string first long string ', 'second long string second long string second long string second long string second long string second long string second long string second long string second long string second long string ', 'third long string third long string third long string third long string third long string third long string third long string third long string third long string third long string ']
>>>
In the example above, it is hard to find where one string starts and ends, and this becomes even more difficult for bigger lists. You can print this kind of list in a more organized manner, with the *
symbol and the sep
argument in the print() function. The sep
argument allows you to replace the default space that separates items in a print() function with any other character.
>>> print(*longStrings, sep='\n------\n')
first long string first long string first long string first long string first long string first long string first long string first long string first long string first long string
------
second long string second long string second long string second long string second long string second long string second long string second long string second long string second long string
------
third long string third long string third long string third long string third long string third long string third long string third long string third long string third long string
>>>
Now each item in the list is separated in a way that makes the output much easier to read.
Print list with loops
Another way to print a list in Python is using loops. For
loops are the more intuitive and simple option, but you can also use while
loops. So we will explore both ways here.
With for loops, you can print the list by simply looping through each item and printing it:
>>>
>>> x = [1, 2, 3, 4]
>>>
>>> for item in x:
... print(item)
...
1
2
3
4
>>>
This allows you to individually format how each item will be printed out. However, each item will always be printed on a new line. But, you can fix this with the end
argument in the print() function. This argument allows you to replace the '\n' (newline) character, which is default after every print() output element, with another string:
>>> for item in x:
... print(item, end=" - ")
...
1 - 2 - 3 - 4 - >>>
You can also print a list with a for loop by looping through the range of indices:
>>> for idx in range(len(x)):
... print(x[idx])
...
1
2
3
4
>>>
This can prove useful when you want to specify the way you print the items in the list based on their index value.
We can use this same idea of using indices to print items and instead use a while loop.
>>> idx = 0
>>> while idx < len(x):
... print(x[idx])
... idx += 1
...
1
2
3
4
>>>
First, the idx
variable is set to 0, and then the while loop prints the value of x
at index idx
and increments idx
by 1. The loop ends when idx
reaches the length of the list x
.
Print list with join() function
Another way to print lists is to use the join()
method to join the items in the list into a single string, and then print it.
>>> y = ['a', 'b', 'c', 'd']
>>> joinedList = ' '.join(y)
>>> print(joinedList)
a b c d
>>>
Here, the items in list y
are joined with space between them. You can replace the space with any string you want to use as a separator.
However, there is one caveat to using the join() method. join() is a string method, which means that all the elements in the list must strings. If you know that the items in the list are not all strings or you are uncertain, you can use the map()
function to make use of join().
With the map() function you can map all items in the list to the str
function which will convert the items to string before they are joined:
>>> x = [1, 2, 'a', 'b']
>>> joinedList = ' - '.join(map(str, x))
>>> print(joinedList)
1 - 2 - a - b
>>>
Here, even though the list x
consists of both integers and strings, the items are converted to string datatype with map() and then joined.
The key advantage of using the join() method to print lists is that you can store the printed output to a variable beforehand. This can be useful in certain situations, where you want to do something else with the output rather than printing it.
Print list in reverse
Another way you might want to print a list is in reverse. For this, python has the reverse()
method and the reversed()
function. The reverse() method will reverse the original list in-place - the original list will be modified. The reversed() function will return an iterator that will output the items in reversed order, leaving the original list unaffected.
>>> x = [1, 2, 3, 4]
>>> x.reverse()
>>> print(x)
[4, 3, 2, 1]
>>>
>>> y = [1, 2, 3, 4]
>>> reversed(x)
<list_reverseiterator object at 0x7fd4c81cb910>
>>>
With the reverse() method you can reverse the original list and then use the techniques shown in the previous section to print the list accordingly. For the reversed() function you can convert the iterator output into a list with the list()
function and then print the list. But, when using a for loop to print the list you can directly loop through the iterator output of reversed():
>>> for item in reversed(y):
... print(item)
...
4
3
2
1
>>>
Reversing a list and then printing its output can be very useful in situations dealing with sorted lists.
Summary
In this article, we discussed various ways to print lists in Python and the benefits of each method.
First, you saw the most basic way to print a list directly with the print() function. Then we discussed why the basic way might not be sufficient. Next, you saw how you can print lists with for loops and while loops. Then you saw how you can use the join() method to print a list and understood why it might be advantageous in certain situations. Finally, you saw how to print a list in reverse with the reversed() function and reverse() method.
Next Steps
If you're interested in learning more about the basics of coding, programming, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers