Python Square Root
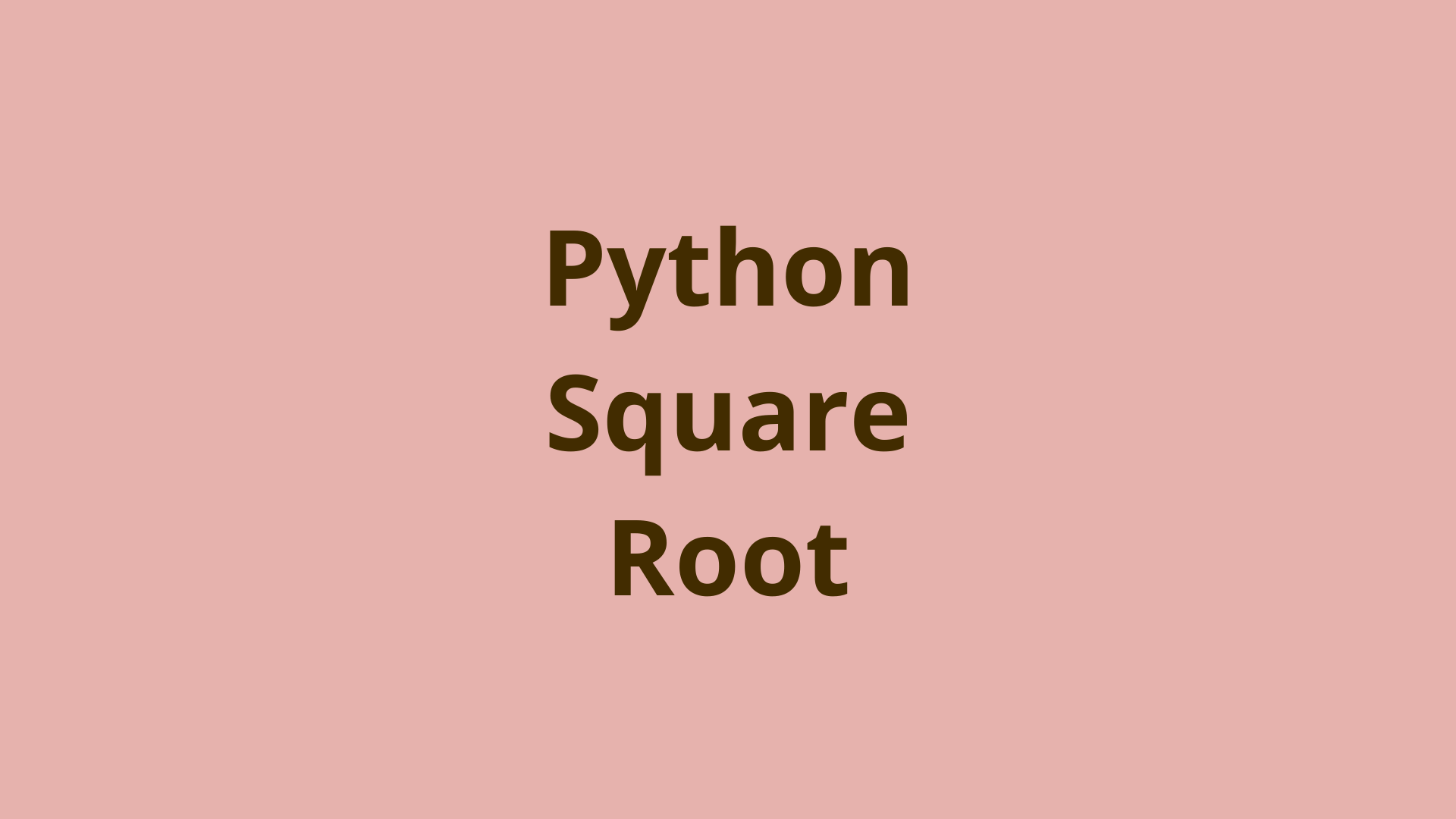
ADVERTISEMENT
Table of Contents
- Introduction
- Calling the Python Square Root Function
- Calling the Numpy Square Root Function
- Calling the Python Power Function
- Calling the Cmath Square Root Function
- Summary
- Next Steps
Introduction
One of the benefits of being a programmer is that you'll always have a calculator on hand. All programming languages contain a plethora of functions that allow you to perform various mathematical calculations on numbers.
For example, say you want to find the square root of a number. This is the number, b, that when multiplied by itself yields the number a: a = b * b
or a = b²
. Here, b is called the square root of a.
In this article, you'll take a look at several different methods you can use to find the square root of a given number.
Calling the Python Square Root Function
The first and most common method you can use to find the square root of a given number is to use the Python square root function located in the math
module. The math module contains additional functions you can use to perform mathematical calculations. It's part of the Python standard library, meaning it comes prepackaged with your Python installation and can be imported immediately:
>>> import math
Then, you specify the function from the math module that you want to call - in this case, math.sqrt()
:
>>> math.sqrt(4.0)
2.0
The Python square root function found in the math module takes in a number and returns its square root. The number you pass in can either be an integer or a float. However, note that math.sqrt() will always return a floating point number:
>>> math.sqrt(4) # passing in an integer
2.0 # still returns a floating point number
If instead you want to return an integer, then you can round down the result like so:
>>> round(math.sqrt(4))
2
You can also call the math.isqrt()
function, which will return an integer value by rounding down the result to the nearest whole integer:
>>> math.sqrt(12) # returns the precise float
3.4641016151377544
>>> math.isqrt(12) # returns the nearest lowest whole number
3
You may recall that square roots are only real for numbers that are greater than zero. In other words, the domain of numbers that you are allowed to find real square roots for are limited to n > 0
:
>>> math.sqrt(-4)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: math domain error
As you can see, if you try to pass a negative number to the Python square root function located in the math module, you get a ValueError
letting you know that the argument passed in is outside the domain of allowed values.
This makes intuitive sense: a negative times a negative always equals a positive, and the square root is by definition a number multiplied by itself. As such, negative real squares can't exist, and Python won't return a real value for such a number. (It's a bit complex, as you'll see later on.)
For now, you can coerce the input to always be positive by using the Python absolute value function:
>>> math.sqrt(abs(-4))
2.0
Calling the Numpy Square Root Function
NumPy is another library that can be added to your Python installation. It stands for Numerical Python and allows programmers to perform advanced mathematical calculations; however, it of course offers functionality for simpler operations, like finding the square root of a number. NumPy does not come prepackaged with Python, so you may need to download it with your package manager first:
$ python3 -m pip install numpy
Once you have it installed, you import it just like you did the math module:
>>> import numpy as np
By convention, the NumPy module is aliased to the letters np
, which can save you some keystrokes. Finally, call the square root function from numpy
:
>>> np.sqrt(4)
2.0
One benefit to using numpy over the standard Python square root function is if you needed to calculate multiple square roots at once. The standard square root function requires a real number as an argument:
>>> math.sqrt([4,9,16,25])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: must be real number, not list
NumPy, on the other hand, works with arrays and can therefore handle multiple inputs at once - for example, in the form of a list:
>>> np.sqrt([4,9,16,25])
array([2., 3., 4., 5.])
The result is an array containing the square root for each matching element in the input object.
However, the NumPy square root function won't return a value for numbers outside the appropriate domain:
>>> np.sqrt([4,9,-16,25])
<stdin>:1: RuntimeWarning: invalid value encountered in sqrt
array([ 2., 3., nan, 5.])
Instead, it does two things. First, it raises a RuntimeWarning
which tells you there's an invalid value somewhere in your input. Still, it produces output instead of erroring out, with a nan
value in the location where the invalid input was found. This can help you to debug your input.
Calling the Python Power Function
You may recall that squaring and exponentiation are related. In other words, finding the square root is the same as raising a number to the one-half power. In that case, the Python power function can also be used to find the square root of a number.
This function is also located in the math module and accepts two arguments:
math.pow(base, exponent)
The first number is the base
, or the number that you want to raise to some power (for the purposes of this article, it's the number you want to find the square root of). The second number is the exponent
, or the power you want to raise the number to. For square root finding, that's going to be 1/2
.
Here's an example:
>>> math.pow(4, 0.5)
2.0
Four raised to the one-half power is the same thing as the square root of four, so math.pow()
appropriately returns 2.0.
As usual, you need to consider the domain of numbers for which square roots can be found when using this method:
>>> math.pow(-4, 0.5)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: math domain error
However, Python also has a built-in power function - not the one from the math module - that will return a value for a negative number:
>>> pow(-4,0.5)
(1.2246467991473532e-16+2j)
This output may look a bit strange if you've never seen complex numbers before, but a complex number is the result of squaring a negative number. So, while the previous methods have been unable to give a result for numbers outside the traditional domain, the in-built Python power function will indeed return a result in the form of a complex number.
That depends, however, on which version of Python you're using. For instance, 2.7 will raise a ValueError
:
>>> pow(-4,0.5)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: negative number cannot be raised to a fractional power
In that case, you need to explicitly tell Python 2.7 that you're looking to work with complex numbers:
>>> pow(complex(-4),0.5)
(1.2246467991473532e-16+2j)
Now, you get a complex number as expected.
Calling the Cmath Square Root Function
You can also work with complex numbers by importing the cmath
module, or the complex math module. This module will handle square roots of negative numbers beautifully, but be careful: it will also coerce the results of positive numbers to complex ones! Be sure that's what you want before proceeding. If so, then import it like so:
>>> import cmath
Then, you can call the square root function and have a complex number returned:
>>> cmath.sqrt(-4)
2j
You can even look at the real and imaginary parts of the number separately:
>>> result.real
0.0
>>> result.imag
2.0
Again, note that this module will return a complex result even for a number that's within the square root domain:
>>> cmath.sqrt(4)
(2+0j)
Summary
In this article, you learned several different ways to calculate the square root of numbers in Python. You considered the domain of allowable numbers when calculating square roots, explored the various error messages you might encounter, and figured out a few different ways to work with negative squares and retrieve complex results.
Next Steps
The Python math module is absolutely packed with several different functions you can use to perform mathematical calculations. For instance, you can use it to floor a number down to its next lowest integer.
If you don't want to use other modules, then you can still do quite a number of operations with just the initial Python installation. You can can find the absolute value of a number without any fancy imports.
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers