Software-defined networking (SDN) with Node.js
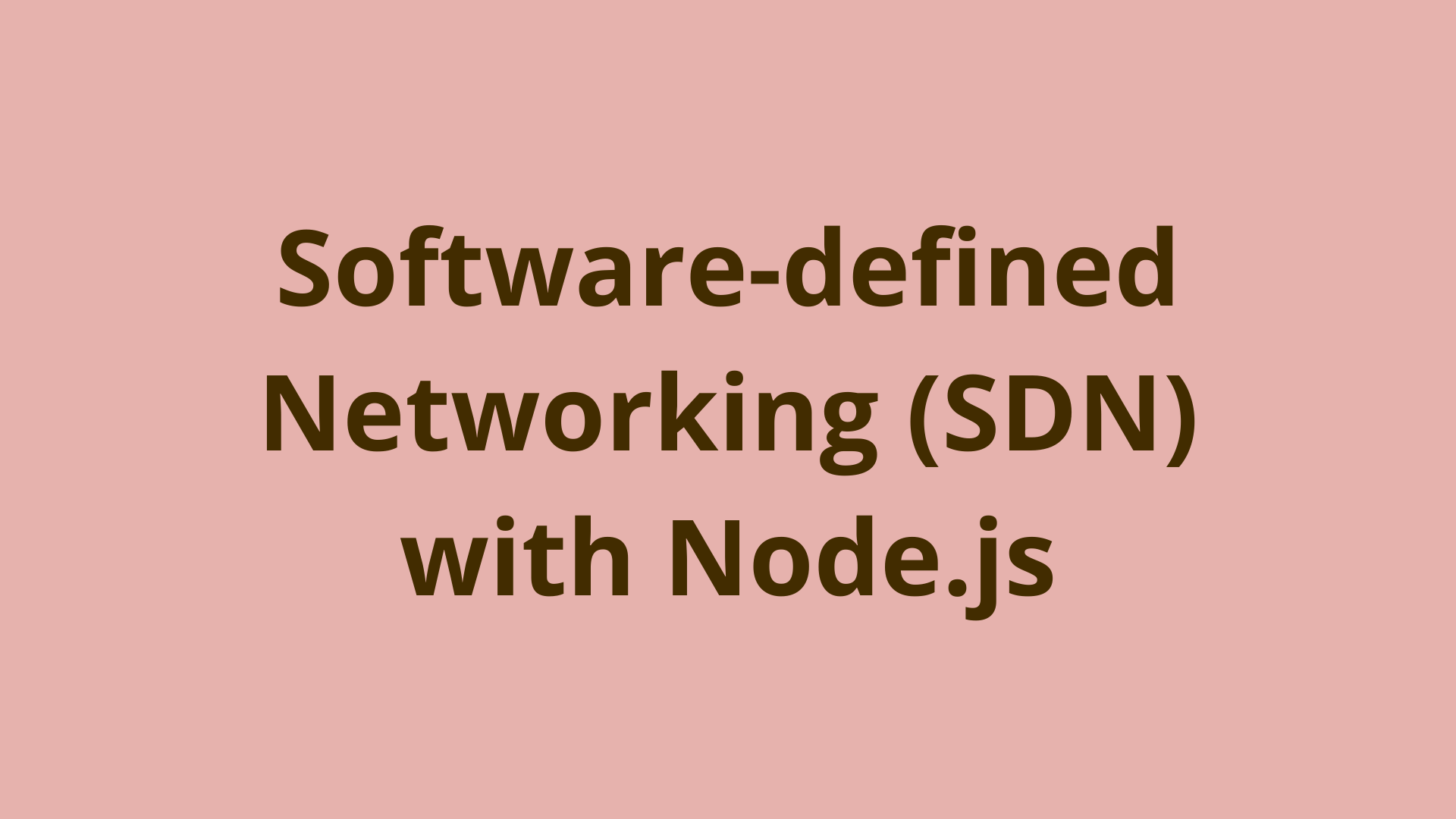
ADVERTISEMENT
Table of Contents
- Introduction
- Getting started with Node.js
- Creating a Node project
- Creating a networking module
- Publishing a module to NPM (Node Package Manager)
- Testing modules
- Summary
- Next Steps
Introduction
Software-defined networking is an emerging field with lots of room for innovation. There have been many companies, cough BitTorrent cough, which have really managed to wrangle this topic to create great Software. Companies are often in the SDN news, take Twitch, for example, a live video gaming streaming company, requiring heavy use of strong computer networks to relay live streaming data. Developers and organizations are getting behind SDN and Node.js to build interoperability between Software applications.
In this post, we will build a Software-defined Networking application, showing how easy the Node.js build process really is. We are blown away by how fast it was to develop the app from idea, right through to delivery. Node.js is an excellent framework choice for a number of reasons, they are:
- Interoperability between Software Applications
- Configuration of the Network Layer from the Application Layer
- Easy to publish updates and allows for Application versioning
- Increases Build Velocity supporting different types of Applications
Getting started with Node.js
Get up and running with Node.js by downloading the latest version:
https://nodejs.org/en/download/
Creating a Node project
Create an empty directory:
mkdir networking-module
Initialize a new node project:
cd networking-module
npm init
Creating a networking module
To create a networking module, we need to write Javascript code and upload it to npm
.
Below we have written a simple application to display the TCP/IP Networking Configuration information of a host machine.
This information is quite useful in diagnosing network problems and outages.
It provides information based on the local host machine, such as the IP address, type of Network running, Subnet mask, etc.
'use strict';
var os = require('os');
var ifaces = os.networkInterfaces();
exports.displayNetworkInfo = function() {
Object.keys(ifaces).forEach(function(ifname) {
var alias = 0;
ifaces[ifname].forEach(function(iface) {
if ('IPv4' !== iface.family || iface.internal !== false) {
return;
}
if (alias >= 1) {
//many hosts
console.log();
console.log('Network information...');
console.log();
console.log('Network type:' + ifname);
console.log('Local IP:' + iface.address);
console.log('IP Version: ' + iface.family);
console.log('Mac address v6:' + os.networkInterfaces()[ifname][0].address);
console.log('Subnet Mask:' + os.networkInterfaces()[ifname][1].netmask);
} else {
//one host
console.log();
console.log('Network information...');
console.log();
console.log('Network type: ' + ifname);
console.log('Local IP: ' + iface.address);
console.log('IP Version: ' + iface.family);
console.log('Mac address v6: ' + os.networkInterfaces()[ifname][0].address);
console.log('Subnet Mask: ' + os.networkInterfaces()[ifname][1].netmask);
}
++alias;
});
});
}
You can view the full source code here.
Publishing a module to NPM (Node Package Manager)
https://www.npmjs.com/signup
Once signed up, we can log in using the terminal to the account created.
npm login
After logging in, you're ready to publish!
npm publish
Testing modules
When testing NPM modules, you can think of it like someone else is now using your software networking application in their project. We will create a test project and test
the functionality as if someone else was using your software.
mkdir TestProject
cd TestProject
Create the test node project:
npm init
Install your created module:
npm install network-info
var testmodule = require('network-info')
testmodule.displayNetworkInfo();
Execute the test script:
node index.js // Inside the TestProject directory
After running the test script, the following output will show:
Network information...
Network type: _____
Local IP: _____
IP Version: _____
Mac address v6: _____
Subnet Mask: _____
Summary
In this article we discussed creating a Software-defined Networking module in Node.js. The repo has been published to the Node registry.
Next Steps
If you're interested in learning more about the basics of coding and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers