Getting Started with Java Visualizer
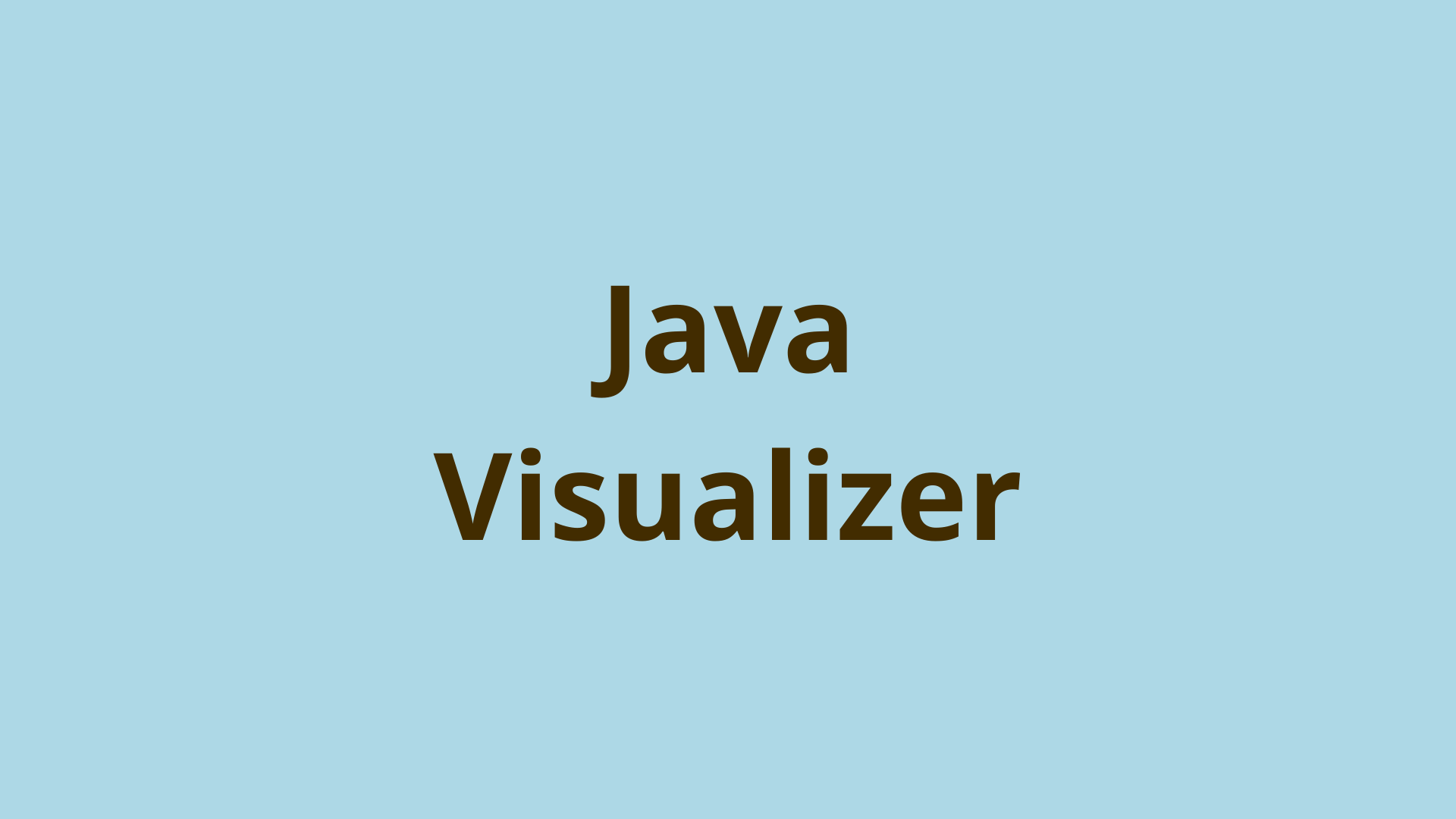
ADVERTISEMENT
Table of Contents
- Introduction
- What is Java Visualizer?
- Benefits of Using Java Visualizer
- Using Java Visualizer to explore your code
- Example 2: For Loops
- Sharing your visualization
- Summary
- Next Steps
Introduction
In this article, we'll discuss getting started with Java Visualizer - a visual tool that can be useful for developers of all skill levels to better learn Java and understand their code.
What is Java Visualizer?
The Java Visualizer is an in-browser interactive development environment that developers can use to easily step through their Java code. After entering code in the website, users can click visualize execution to start stepping through their code. From there, users can click forward or back to move stepwise through their code in either direction or click first or last to jump to the start or end points of execution.
Compared to traditional debuggers, Java Visualizer focuses on providing an easy-to-use learning environment for beginning coders. It provides visual cues, highlighting lines as they’re executed as well as showing current outputs and object values as the code executes. Because users can move both forward and backward through the code, it also provides a way for users to really explore how their code is executing.
Benefits of Using Java Visualizer
This tool works particularly well to help new developers better understand their code. When first starting out, it can be difficult to learn the execution order and effects of various calls. The Visualizer provides a simple way for users to walk through the steps of execution.
Additionally, the Java Visualizer can be helpful for visual debugging. While it’s in-browser nature limits its utility for larger projects, it can be useful for beginner programmers looking to debug their code. Traditional debugging tools can be tricky and some bugs can be hard to root out. With the visualizer, however, new developers can easily step through their code using an intuitive interface.
Using Java Visualizer to explore your code
The Java visualizer can be used to examine code of varying code complexity but here, we’ll focus on some simple use cases to determine how the visualizer works.
Example 1: Sequential Statements
First, we’ll step through an example where we just print two sequential statements:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("hello");
System.out.println("world");
}
}
This code simply prints out "hello" on one line and "world" on the next. By clicking "forward" on the Java Visualizer example below, you can step through the execution line by line, with the active line highlighted in yellow:
Example 2: For Loops
Next, we’ll show an example where we traverse a for loop:
public class LoopExample {
public static void main(String[] args) {
for (int i=0; i<=2; i++)
{
System.out.println(String.format("Executing loop: Count %d", i));
}
}
}
This example prints the line "Executing loop: Count i" for each of the three integers in the for loop. By stepping through the Java Visualizer example here, you can see the code move through each of the conditions in the for loop before moving to the print statement. After that, the loop continues:
Example 3: Function calls
Finally, we’ll perform a basic function call:
public class FunctionExample {
public static void main(String[] args) {
int num = 3;
num = double_int(3);
System.out.println(String.format("Value of num is now %d", num));
}
private static int double_int(int x) {
return 2 * x;
}
}
When stepping through this example, the Java Visualizer shows the integer being passed to the function call before being returned to main. Again, the Java Visualizer displays the execution line by line:
Sharing your visualization
After entering code into the Java visualizer, there are multiple ways for users to share their visualizations with others. The quickest way to share the visualization with anyone is to simply click "Generate URL" to create a link to a Java Visualizer page that contains the current code. With that link, anyone can access the Java Visualizer code from anywhere. Using a similar technique, users can also generate code to embed the visualization on their website. We’ve used that approach to embed our examples throughout this article. Including embedded references can be especially helpful when writing educational articles.
Summary
In this article, we presented the Java Visualizer, the benefits it can provide to software developers, and several examples of using the tool to get a visual representation of Java code execution. We also showed how to share your visualizations with others for collaboration purposes.
Next Steps
As a further exercise to hone your skills, you could fire up the Java visualizer and test out our example code on how to convert int to string in Java.
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers