Python Ceiling
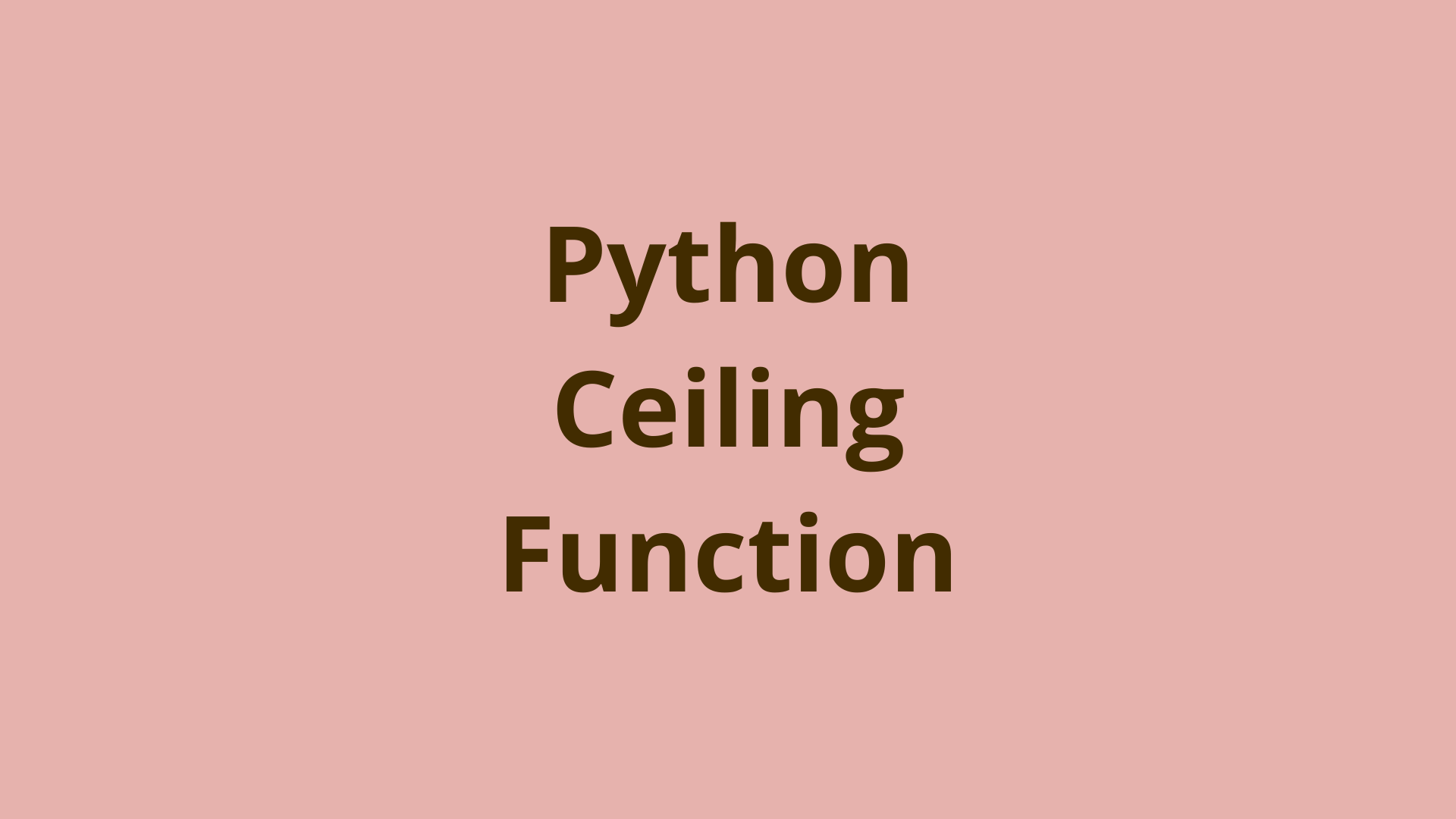
ADVERTISEMENT
Table of Contents
- Introduction
- How does rounding numbers work in Python?
- Overview of the Ceiling function
- math.floor(...) vs math.ceil(...)
- Ceiling constants in Python
- Ceiling negative numbers
- Summary
- Next Steps
Introduction
Rounding decimals is a basic mathematical operation that can prove very useful when programming. With Python, there are various ways to round decimal numbers. You can use the built-in function round(...)
, or you can access other functions from the standard library math
. Two of such functions are the math.floor(...)
and the math.ceil(...)
functions.
In this article, we will explore the math.ceil(...)
function. We'll learn how it interacts with mathematical constants and negative numbers and compare it to the Python floor function math.floor(...)
.
How does rounding numbers work in Python?
Rounding decimal numbers in basic mathematics has three simple rules:
- The number stays the same if the decimal is already a whole number.
- If the value after the decimal point is above 0.0 and below 0.5, the number rounds down.
- If the value after the decimal point is 0.5 or above, the number rounds up to the next whole number.
This form of rounding can be done with Python’s built-in round(...)
function, as shown below:
>>> round(1)
1
>>> round(1.5)
2
>>> round(1.49)
1
>>> round(1.8)
2
But then what is the point of the functions math.floor(...)
and math.ceil(...)
, which we can access from the math
library? Well, they both round floating point numbers in different ways.
Overview of the Ceiling function
The ceiling function in Python is part of the math
standard library. Essentially, the ceiling function always rounds decimal numbers up to the next whole number and returns an integer. For example:
>>> import math
>>>
>>> math.ceil(5.0)
5
>>> math.ceil(5.1)
6
>>> math.ceil(5.0000001)
6
>>> math.ceil(5.5)
6
>>> math.ceil(5.8)
6
Here, we first import the math
library. Then call the math.ceil(...)
function, with integer 5 as the argument, which returns the same number as an integer. But, using float 5.1 returns 6. In the next lines of code, we see that even if the value after the decimal point is as small as 0.0000001, or as large as 0.8, the ceiling function always rounds to the next whole number.
math.floor(...) vs math.ceil(...)
The math.floor(...)
and math.ceil(...)
differ in the direction that the rounding occurs. The ceiling function rounds up to the next whole number, while the floor function rounds down to the previous whole number. Their names represent this exactly. One is called floor (below), and the other is ceil, referring to the ceiling (above). So, when rounding the number 1.5, 1 would be the floor, and 2 would be the ceiling.
Let’s look at how each function rounds floats side by side:
>>> from math import ceil, floor
>>>
>>> print(ceil(8.0), floor(8.0))
8 8
>>> print(ceil(8.49), floor(8.49))
9 8
>>> print(ceil(8.7), floor(8.7))
9 8
In this example, we print the outputs of the ceil(...)
and floor(...)
with the same float. Using the float 8.0, we get the same value back as an integer. But we can see no matter what the decimal value of the float is, the ceil(...)
function will always round to the next whole number, while the floor(...)
function will always round down. Both always return an integer.
Ceiling constants in Python
The Python standard library math
contains several mathematical constants:
>>> math.pi # pi: ratio of a circle's circumference to its diameter
3.141592653589793
>>> math.e # e: base of the natural logarithm
2.718281828459045
>>> math.tau # tau: ratio of a circle's circumference to its radius, equal to 2?
6.283185307179586
>>> math.inf # floating-point positive infinity
inf
>>> -math.inf # floating-point negative infinity
-inf
>>> math.nan # not a number
nan
Below, we can understand how the ceil(...)
function interacts with these constants:
>>> from math import ceil
>>> import math
>>>
>>> ceil(math.pi)
4
>>> ceil(math.e)
3
>>> ceil(math.tau)
7
>>> ceil(math.inf)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
OverflowError: cannot convert float infinity to integer
>>> ceil(-math.inf)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
OverflowError: cannot convert float infinity to integer
>>> ceil(math.nan)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: cannot convert float NaN to integer
The pi, e, and tau constants work like any other float; they round to the next whole number. But, positive infinity, negative infinity, and NaN
produce throw a Python exception.
Both infinites produce the OverflowError
because the ceil(...)
function tries to output the next whole number, but infinity is too large, and the function cannot calculate it. NaN
produces an error because it is not a number and cannot be converted to a numeric type to be ceilinged.
Ceiling negative numbers
The math.ceil(...)
function works the same way with negative numbers, but it can be a bit confusing to the the reversed directions of positive and negative magnitudes. When the input is a negative number, the ceiling is still the closest whole integer that is greater than the input. This means that the ceiling of a negative decimal number is the next greatest integer toward zero. The opposite it true for floor.
>>> import math
>>>
>>> print(math.floor(1.4), math.ceil(1.4))
1 2
>>> print(math.floor(-1.4), math.ceil(-1.4))
-2 -1
>>> print(math.floor(5.7), math.ceil(5.7))
5 6
>>> print(math.floor(-5.7), math.ceil(-5.7))
-6 -5
In this example, two numbers 1.4 and 5.7, are inputted as positive and negative to the math.floor(...)
and the math.ceil(...)
function.
Looking at the output from negative numbers allows us to better grasp how the ceiling function works. For positive numbers, the output of the ceiling function is the next whole number. But specifically, the next whole number on the right side of the number line. While flooring outputs the next whole number on the left side of the number line.
Summary
In this article, we learned how the math.ceil(...)
function works in Python. First, we looked at how rounding decimal numbers in Python works with the built-in round(...)
function. Then we compared the math.floor(...)
and math.ceil(...)
functions. We saw examples of using the ceiling function with different mathematical constants. Finally, we saw how ceiling works with negative numbers and its relation to flooring.
Next Steps
In this article, we learned about ceiling in Python. To learn more about its counterpart, we wrote a more detailed look at the Python floor function.
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers