Python Newline Character \n | Use Cases and Examples
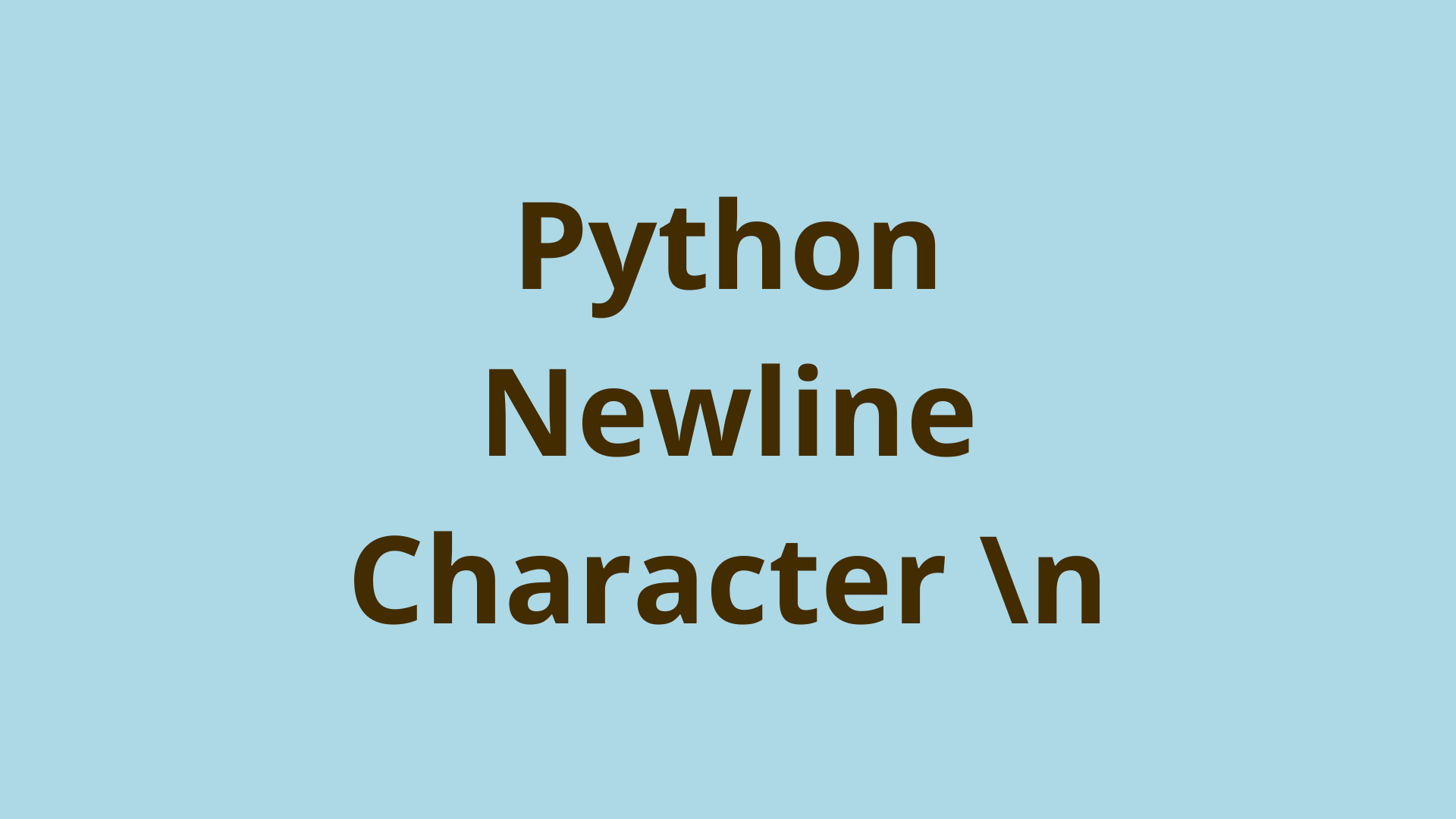
ADVERTISEMENT
Table of Contents
- Introduction
- What is the the newline character \n?
- Using the Newline Character in Python
- Python Newline \n Examples
- The Newline Character in a Python Function
- The Newline Character and Printing in Python
- Summary
- Next steps
- References
Introduction
The newline character is a sequence that performs a simple function and its use can be found in multiple programming languages including Python, Java, C++, and many others. An understanding of what the newline character does is useful knowledge for any programmer to have.
In this article, we will be exploring the use of the newline character in Python and the applications it has behind the scenes. It has a very simple purpose, but versatile nonetheless, let’s start at the top.
What is the the newline character \n?
To answer this question, let's start by mentioning escape sequences and note that the newline character is one of them. In essence, an escape sequence character allows the programmer to interrupt a certain procedure, carry out an instruction, and resume the procedure.
In this case, the procedure is the addition of a newline. Essentially, the use of \n
escapes the current line of code and resumes it on a new line. In Python, the backslash denotes the start of an escape sequence, indicating the the following n should not be treated as a regular letter, but instead as a new line, also known as a carriage return.
This allows you to link multiple lines together in a single Python string object, and have them display on different lines when output to the end user. New lines can be used to format text output in ways that are visually appealing and easy for the end user to understand, without requiring them to search hard to find a piece of text.
For example, the code below shows the difference in executing a string with and without the new line character:
The newline character \n signifies the end of a line. It can be used by itself, or in conjunction with other escape sequences.
>>> print("Initial Commit");
Initial Commit
>>> print("Initial\nCommit")
Initial
Commit
As you can see, the original Python print line statement places all characters on the same line however, the second print statement makes use of \n
and continues printing the string on the following line. For example, these strings could simply be printed in the Python interpreter, as text output to a program user, or written to a file.
Using the Newline Character in Python
The new line character can be used in many ways depending on what the programmer is utilizing it for. Yet, here are some common applications for using it:
Managing \n in a String
There are times when you may find it useful to use the Python print newline character to make the output look neater and less cramped. Take, for instance, the example below.
>>> print("1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20")
1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20
>>> print("1,2,3,4,5,6,7,8,9,10,\n11,12,13,14,15,16,17,18,19,20")
1,2,3,4,5,6,7,8,9,10,
11,12,13,14,15,16,17,18,19,20
The first Python print string statement outputs characters that are clumped together and hard to read. Scaled to hundreds, thousands, or millions of numbers, it would be incredibly difficult for a programmer to understand what the output means. The second print statement resolves this problem by utilizing a newline character to break up the output after the first 10 numbers.
Newline Character and Python Output
Having covered the basics of what the new line character is and what it does, it’s only fitting to explore some more use cases any programmer might eventually run into. The chief of which is formatting text.
Text formatting is something that isn’t often thought about but can be very important. When developing programs that process or output text, it’s important to define boundaries using \n
instead of subjecting yourself to walls of text.
>>> # (User input is 123, followed by ABC)
>>> numbers = input()
>>> letters = input()
>>> print(numbers + "\n" + letters)
123
ABC
As can be seen in the code above, using \n
can be used to control the output given user input. This is one simple way of controlling the flow between input and output with the newline character in python.
Python Newline \n Examples
Now let's go over a few Python new line examples.
Inserting a Newline
>>> print("This text is up here\n and this text is down here")
This text is up here
And this text is down here
Newline in an Iterator
>>> example_list = ["Python","Java","Swift"]
>>> for item in example_list:
... print(item)
Python
Java
Swift
The newline is still being used here, as it is included inside the "print" statement by default. There is no need to include \n
in this block of code.
The Newline Character in a Python Function
def example_error():
print("This line of code could not be compiled because the variable 'x' \n could not be found within the given scope.")
>>> example_error()
This line of code could not be compiled because the variable 'x'
could not be found within the given scope.
The Newline Character and Printing in Python
When printing in Python, it should be noted that the newline character \n
is automatically added to the end of a print statement by default. So there’s no need to add the escape sequence when printing normally.
However, there can still be confusion in Python as to the difference between printing a string and processing a string. Python allows you to work with its interpreter directly; while this is an advanced topic, here’s the breakdown.
When using python, if the user inputs raw data instead of a command, they will come across REPL (Read, Evaluate, Print, Loop) or Python’s way of user/interpreter interaction. Essentially, when provided raw data, REPL will spit out what it has interpreted that data to be.
>>> example_string = "Initial\nCommit"
>>> print(example_string) # Invoking the print function
Initial
Commit
>>> example_string = "Initial\nCommit"
>>> example_string # Invoking use of python's REPL
'Initial\nCommit'
In the example above, the first block of code executes a print statement, so the interpreter knows to interpret the given code as a string and prints thusly. In the second block of code, there was no print statement, instead, there was raw input. The REPL detected the raw data, extracted the data’s properties, then printed them onscreen.
Summary
This article went over what the newline character is, how to identify it, how to use it, and cleared up some confusion in its use. However, there’s that can be done with \n
than was shown in this article. Of course, the best way is to play around with it and find out yourself, but If you want to know more, we have an article detailing Python multiline strings use as well as others to explore the world of Python coding further.
Next steps
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- REPL (Read, Evaluate, Print, Loop) - https://www.learnpython.dev/01-introduction/02-requirements/05-vs-code/04-the-repl-in-vscode/
- Escape Sequences - https://www.scaler.com/topics/escape-sequence-in-python/
Final Notes
Recommended product: Coding Essentials Guidebook for Developers