Return Outside Function error in Python
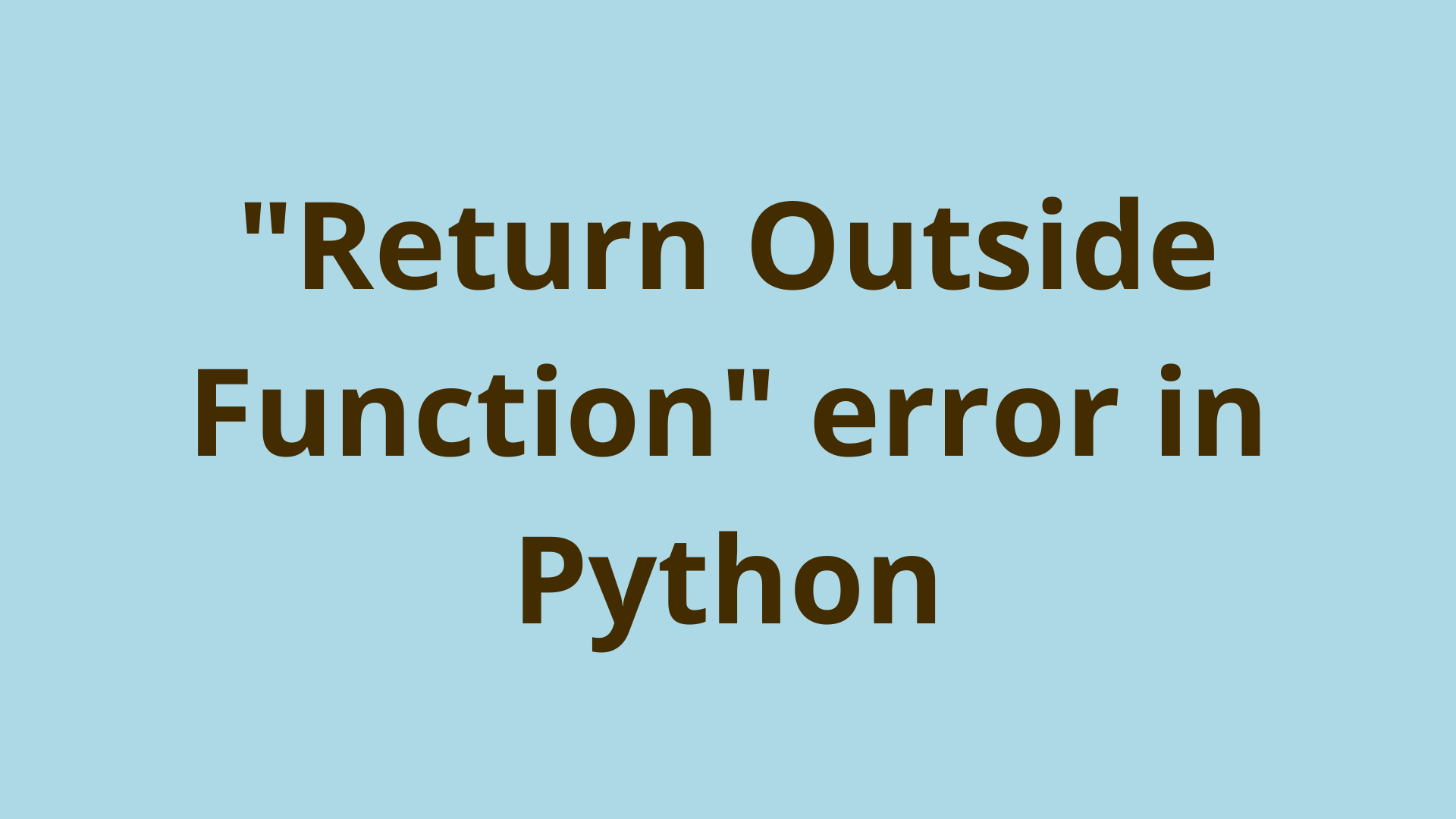
ADVERTISEMENT
Table of Contents
- Introduction
- The Return Statement
- Return Outside Function Python SyntaxError
- Return Outside Function Python SyntaxError due to Indentation
- Return Outside Function Python SyntaxError due to Looping
- Summary
- Next Steps
Introduction
Functions are an extremely useful tool when it comes to programming. They allow you to wrap a block of code into one neat package and give it a name. Then, you can call that block from other places in the code, and perform the defined task as many times as you'd like.
What's more, functions allow you to return the result of some computation back to the main Python program. However, you need to be careful how you do this; otherwise, you might get an error.
The Python language can throw a variety of errors and exceptions, such as AttributeError, TypeError, and SyntaxError.
In this article, you'll take a closer look at the return
statement and how to fix the return outside function Python error, which you may have encountered as:
SyntaxError: ‘return’ outside function
The Return Statement
The return
statement is used to end a function’s execution and pass a value back to where a function was called. For example:
def return_variable():
a = 1
return a
You define a function called return_variable()
that assigns the value 1 to the variable a
. Then, it returns that value, passing it back to the main Python program for further processing.
If you run this code in the interpreter, then calling the function name will immediately display the result of the return statement:
>>> def return_variable():
... a = 1
... return a
...
>>> return_variable()
1
The function call runs the code inside the function block. Then, the function sends the result of a
back to the interpreter.
If you instead defined this function in a file, then you could use the print statement to show the result instead:
# return_variable.py
def return_variable():
a = 1
return a
print(return_variable())
Now, the return statement sends the result back to the main Python program that's running. Here, it's the file return_variable.py
. In other words, you can now use the result of that return statement anywhere else in the file - for instance, in the print statement at the end. When you run this file from the terminal, the result will be displayed:
$ python3 return_variable.py
1
Another common technique is to assign the result of the function to a variable:
# return_variable_2.py
def return_variable():
a = 1
return a
b = return_variable()
print(b)
No matter where you define the function, the return
statement will allow you to work with the result of a function.
Return Outside Function Python SyntaxError
If the return statement is not used properly, then Python will raise a SyntaxError
alerting you to the issue. In some cases, the syntax error will say ’return’ outside function
. To put it simply, this means that you tried to declare a return statement outside the scope of a function block.
The return statement is inextricably linked to function definition. In the next few sections, you'll take a look at some improper uses of the return statement, and how to fix them.
Return Outside Function Python SyntaxError due to Indentation
Often, when one is confronted with a return outside function Python SyntaxError, it's due to improper indentation. Indentation tells Python that a series of statements and operations belong to the same block or scope. When you place a return statement at the wrong level of indentation, Python won't know which function to associate it with.
Let's look at an example:
>>> def add(a, b):
... c = a + b
... return c
File "<stdin>", line 3
return c
^
SyntaxError: invalid syntax
Here, you try to define a function to add two variables, a
and b
. You set the value of c
equal to the sum of the others, and then you want to return this value.
However, after you enter return c
, Python raises a syntax error. Notice the caret symbol ^
pointing to the location of the issue. There's something wrong with the return statement, though the interpreter doesn't say exactly what.
If you place the function in a file and run it from the terminal, then you'll see a bit more information:
$ python3 bad_indentation.py
File "bad_indentation.py", line 5
return c
^
SyntaxError: 'return' outside function
Now you can see Python explicitly say that there's a return statement declared outside of a function.
Let's take a closer look at the function you defined:
The red line shows the indentation level where the function definition starts. Anything that you want to be defined as a part of this function should be indented four spaces in the lines that follow. The first line, c = a + b
, is properly indented four spaces over, so Python includes it in the function block.
The return statement, however, is not indented at all. In fact, it's at the same level as the def
keyword. Since it's not indented, Python doesn't include it as part of the function block. However, all return statements must be part of a function block - and since this one doesn’t match the indentation level of the function scope, Python raises the error.
This problem has a simple solution, and that's to indent the return statement the appropriate number of spaces:
Now, the return statement doesn't break the red line. It's been moved over four spaces to be included inside the function block. When you run this code from a file or in the interpreter, you get the correct result:
>>> def add(a, b):
... c = a + b
... return c
...
>>> add(2, 3)
5
Return Outside Function Python SyntaxError due to Looping
Another place where one might encounter this error is within a loop body. Loops are a way for the programmer to execute the same block of code as many times as needed, without having to write the block of statements explicitly each time.
The way a loop is defined may look similar to how a function is defined. Because of this, some programmers may confuse the syntax between the two. For instance, here's an attempt to return a value from a while loop:
>>> count = 0
>>> while count < 10:
... print(count)
... if count == 7:
... return count
... count += 1
...
File "<stdin>", line 4
SyntaxError: 'return' outside function
This code block starts a count at zero and defines a while loop. As long as count
is less than ten, then the loop will print its current value. However, when count
reaches 7, then the loop should stop and return its value to the main program.
You can clearly see that the interpreter raises a return outside function Python syntax error here as well. The interpreter points out the location of the error: it's in line 4, the fourth line of the while loop body, which says return count
.
This raises an error because return statements can only be placed inside function definitions. You cannot return a value from a standalone loop. There are two solutions to this sort of problem. One is to replace return
with a break
statement, which will exit the loop at the specified condition:
>>> count = 0
>>> while count < 10:
... print(count)
... if count == 7:
... break
... count += 1
...
0
1
2
3
4
5
6
7
>>> count
7
Now, the fourth line of the loop body says break
instead of return count
. You can see that the loop executes with no errors, printing the value of count
and incrementing it by one on each iteration. When it reaches 7, the loop breaks. Since you update the value on each iteration, the value is still stored when the loop breaks, and you can print it out to see that it is equal to 7.
However, there is a way to use a return statement with loops. All you have to do is wrap the loop in a function definition. Then, you can keep the return count
without any syntax errors.
Wrap the while loop in a function and save the file as return_while.py
:
# return_while.py
def counter():
count = 0
while count < 10:
print(count)
if count == 7:
return count
count += 1
counter()
The while loop still has a return
statement in it, but since the entire loop body is included in counter()
, Python correctly associates this with the function definition. When you run this code in the terminal, the function will run without issue:
$ python3 return_while.py
0
1
2
3
4
5
6
7
To see the return statement in action, modify the file return_while.py
to store the result of the function call and then print it out:
# return_while.py
def counter():
count = 0
while count < 10:
print(count)
if count == 7:
return count
count += 1
result = counter()
print("The result is: ", result)
Now, when you run this file in the terminal again, the output will more clearly show you the result returned from the function call:
$ python3 return_while.py
0
1
2
3
4
5
6
7
The result is: 7
Summary
In this article, you learned how to fix the return outside function Python syntax error.
You reviewed how the return statement works in Python, then took a look at two common instances where you might see this error raised. Now, you know to check for proper indentation when using return statements and what to do when you want to include them in a loop body.
Next Steps
Functions are an essential part of programming, and when you learn how to write your own, you can really take your code to the next level. If you need a refresher, then our tutorial on functions in Python 3 is a great place to get started.
You don't have to define your own functions for everything, though. The Python standard library comes equipped with dozens you can use straight out of the box, like min() and floor().
If you're interested in learning more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers