The AttributeError: __enter__ Python Error Solved
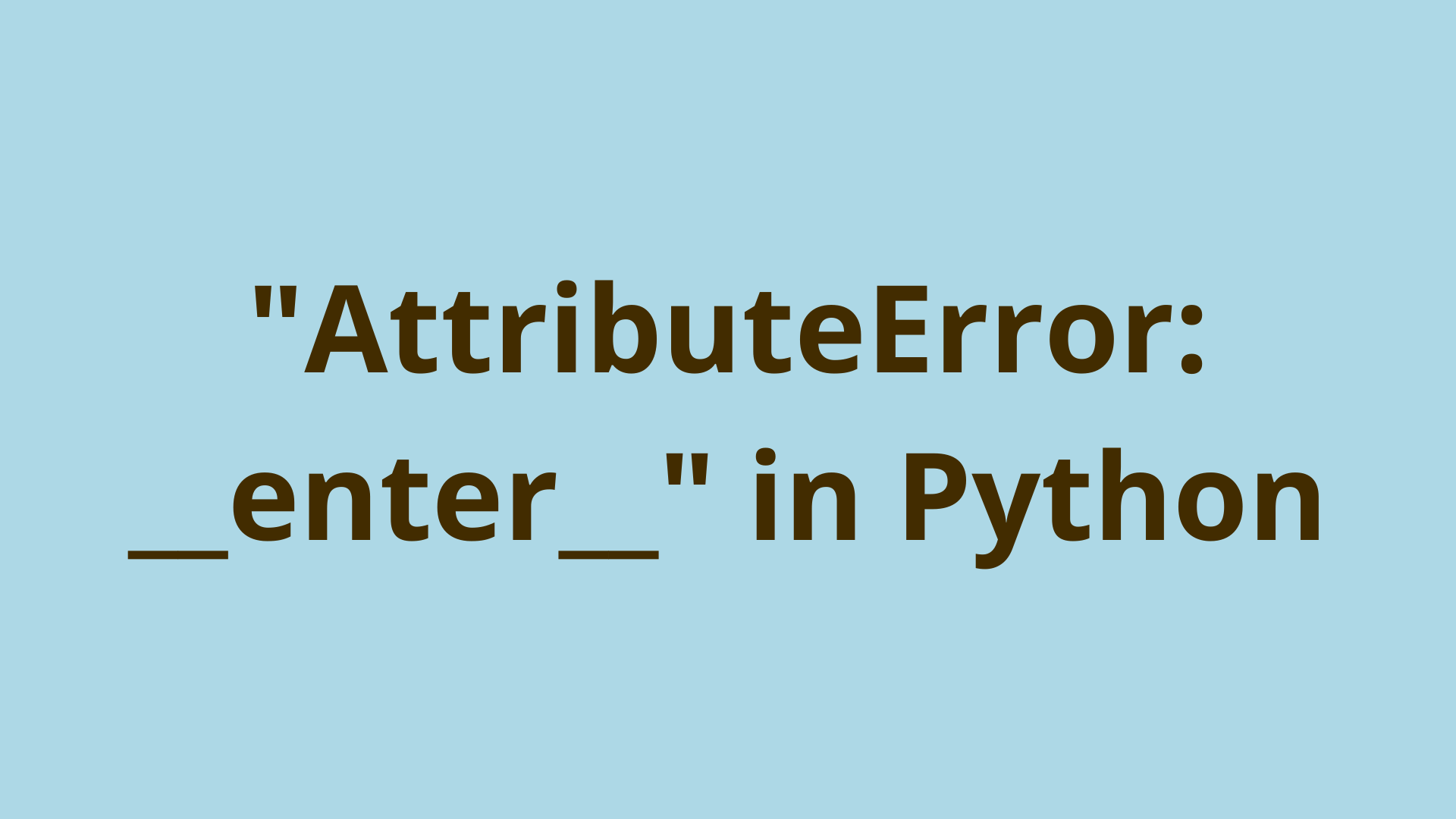
ADVERTISEMENT
Table of Contents
- Introduction
- What are attributes in Python?
- How do you write a context manager in Python?
- What is attributeerror __enter__?
- How do you fix attribute errors in Python?
- Summary
- Next Steps
- References
Introduction
If you run into the common Python error message AttributeError: __enter__
, then you likely have been using the with
statement in Python. The with
statement allows you to use context managers, which enable you to automatically set up and release resources.
This Python error attributeerror can be very confusing and lead you to question what's wrong, but understanding how context managers work will help you to know why the error occurs and prevent it from appearing in the future.
In this article, you'll learn how to fix and prevent the AttributeError: __enter__ error message by understanding what the attributes, context managers, and common mistakes are that can lead to its occurrence.
What are attributes in Python?
An attribute is used to describe a characteristic of an object, and is of the syntax object.attribute
. In Python, there are two types of attributes: class attributes and instance attributes. Both of these store data related to the class.
A class attribute can store simple variable datatypes like integers and strings, or a more complex datatype like a list (array) or dictionary.
Class attributes are shared across all objects and can also be accessed directly from the class variable. Instance attributes are unique to each instance of a class and are often initialized inside the constructor of the class, also known as the __init__ method.
An easy way to identify instance attributes is that they begin with the prefix "self." The word self is a placeholder for the instance being supplied.
For example:
>>> class Example:
... classAttr = 1
...
... def __init__(self, instanceAttr):
... self.instanceAttr = instanceAttr
...
... def exampleFunc(self):
... return 3
...
>>>
Here, you have an Example
class. The classAtrr can be accessed either from the instance of the class or the class variable itself. Methods are also considered to be class attributes. So, the method exampleFunc is also a class attribute.
>>> ins = Example(5)
>>> ins.classAttr
... 1
>>> Example.classAttr
... 1
>>> Example.exampleFunc
<function Example.exampleFunc at 0x7fa20525f280>
The instance attribute on the other hand cannot be accessed from the class variable because it is defined when the instance is created.
>>> Example.instanceAttr
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: type object 'Example' has no attribute 'instanceAttr'
>>> ins = Example(2)
>>> ins.instanceAttr
2
How do you write a context manager in Python?
The context manager in Python allows you to automatically set up resources, and then release them when done.
Context managers are used alongside the with
statement. Understanding the inner workings of a context manager will allow you to better comprehend why you get the AttributeError: __enter__ error message.
The most common example a context manager is accessing a file using the with
statement:
>>> with open("test.txt") as f:
... print(f.read())
...
Test file
>>>
Here, you open the file, read it, and then the close file.
All of this happens automatically.
Without the context manager, you would have to do the same task like so:
>>> f = open("test.txt")
>>> print(f.read())
Test file
>>> f.close()
>>>
In other words, you'd need separate lines of code to open, read, and close the file.
Under the hood, a context manager is a class that uses the __enter__ and __exit__ special methods to define how resources are set up and released, respectively. Let's look at how you can create a context manager with a class and then use it alongside the with
statement:
>>> class ContextManager:
... def __init__(self):
... print("initialized")
... def __enter__(self):
... return "entered!"
... def __exit__(self, exc_type, exc_value, traceback):
... print("exited!")
...
Here, you create the class ContextManager, with the __init__, __enter__ and __exit__ methods. The __enter__ method doesn't need any arguments and returns the string "entered!", but the __exit__ method requires the three arguments shown. Now, let's see how the context manager is used alongside a with
statement:
>>> with ContextManager() as cm:
... print("cm:", cm)
... print("processing 1...")
... print("processing 2...")
...
initialized
cm: entered!
processing 1...
processing 2...
exited!
>>>
The output sheds light on the sequence in which each method of the class is executed. First, the __init__ is run, followed by the __enter__ method at the beginning of the with
statement. The output returned from the __enter__ statement is stored in the variable cm
. Next, the code inside the with block is executed. Finally, the __exit__ method is run.
To summarize, the __enter__ method runs some code before the with
statement, and __exit__ method runs code after.
What is attributeerror __enter__?
A Python error object (or exception) in is a problem in the code that causes the program to not work as intended and terminate.
In Python, errors are also known as exceptions.
The AttributeError exception is raised when you try to access an attribute of an object that does not exist.
As you learned in the previous sections, the __enter__ and __exit__ methods are essential for creating a Context Manager, and these methods are also considered to be attributes. So, the AttributeError: __enter__ is raised when using a with
statement with a class that does not have the __enter__ method.
You can simulate the AttributeError: __enter__ error message by creating a ContextManager without the __enter__ method:
>>> class ContextManager:
... def __init__(self):
... print("initialized")
... def __exit__(self, exc_type, exc_value, traceback):
... print("exited!")
...
>>>
>>> with ContextManager() as cm:
... print("processing...")
...
initialized
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: __enter__
>>>
Note, that both __enter__ and __exit__ are required for a context manager, so if you only have __enter__ method you would run into the AttributeError: __exit__. If neither of the methods are present, then you would only run into the AttributeError: __enter__ because the __enter__ method is always executed first.
How do you fix attribute errors in Python?
If you defined the context manager yourself and you run into this error, then you should make sure both the __enter__ and __exit__ methods are defined correctly with no typos in their names.
However, if using well-known libraries such as TensorFlow or SQLAlchemy, it's very unlikely there are any problems with the context manager under the hood. In this case, you should make sure you properly defined the class in the with
statement. There are two common mistakes that would lead to the AttributeError: __enter__ error message.
The first common mistake is if you made a typo in the name of the class of the context manager and there happens to be another object of that name. For example:
>>> class ontextManager:
... pass
...
>>>
>>> with ontextManager() as cm:
... print("cm:", cm)
... print("processing 1...")
... print("processing 2...")
...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: __enter__
Notice, that instead of calling ContextManager
we call ontextManager
, and there is no class ontextManager in the namespace. This causes us to get the error. It's important that ontextManager is a class in the namespace; otherwise, this would lead to a NameError.
The second common mistake is where you did not add the parentheses to the class of the context manager. For example:
>>> with ContextManager as cm:
... print("cm:", cm)
... print("processing 1...")
... print("processing 2...")
...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: __enter__
Note that if you run into the exception AttributeError: __exit__ there must be a problem under the hood, because the __enter__ must be defined, so the context manager would be partially defined.
Summary
In this article, you learned how to fix the AttributeError: __enter__ error message and understood what attributes, context managers, and errors are in Python.
First, you started by understanding what class and instance attributes are in Python, and recalled that methods are also classified as attributes. Next, you learned exactly what context managers are, how you can use them alongside the with
statement, and how to create them with a class in Python. You followed this by learning what the AttributeError: __enter__ is and why it occurs by simulating its exception. Finally, to bring all this information together you understood why the AttributeError: __enter__ is occurring for you and how you can prevent it in the future.
Next Steps
Running into more errors while working with Python? Check out our tutorials on the return outside function error or the Python SyntaxError.
You can read more about the AttributeError in Python's official documentation. This is a built-in exception, which means it comes as part of of the Python standard library. Your programs will be able to catch such errors out-of-the-box.
To learn more about the basics of Python, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
References
- Context Managers - https://book.pythontips.com/en/latest/context_managers.html
- Python Class Attribute: Class Attribute vs. Instance Attribute - https://dzone.com/articles/python-class-attributes-vs-instance-attributes
- Context Manager in Python - https://www.geeksforgeeks.org/context-manager-in-python/
Final Notes
Recommended product: Coding Essentials Guidebook for Developers