Build SOAP service with JAX-WS
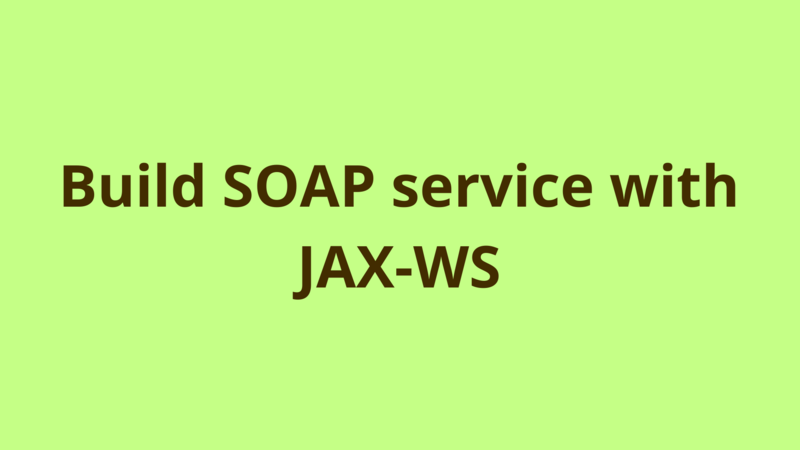
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Create standalone Java project
- 2. Create service endpoint
- 3. Create service implementation
- 4. Publish the service
- 5. Test the service
- Summary
- Next Steps
Introduction
JAX-WS is an API used for building and consuming XML web services in Java, it was first released in Java EE5.
In this tutorial, we provide a step-by-step guide on how to build SOAP web service with JAX-WS and finally publishing it using Endpoint built-in class.
Prerequisites:
- Eclipse IDE (Neon release)
- Java 1.8
1. Create standalone Java project
Open eclipse, then select Java -> New -> Java Project
Name your project as JAXWSSoapServiceUsingEndpoint then click Finish.
2. Create service endpoint
The first step is to create an interface which exposes the different methods provided by our web service, so we create a new class called HelloWorldService under com.programmer.gate package.
package com.programmer.gate;
import javax.jws.WebMethod;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import javax.jws.soap.SOAPBinding.Style;
@WebService
@SOAPBinding(style = Style.RPC)
public interface HelloWorldService {
@WebMethod
public String sayHello();
}
Following are the annotations used to make our interface act as a service endpoint:
- @WebService: this annotation defines our interface as a service endpoint.
- @SOAPBinding: this annotation defines the style of the request body messages, there are 2 types: RPC & Document, the default style is Document. The main difference between them is that with document style , the request body can be fully validated using XML parser, however RPC style messages are wrapped with a wrapper element. Document styles are recommended for invoking operations with complicated requests/responses.
- @WebMethod: this annotation exposes our method sayHello() as a web service operation.
3. Create service implementation
The next step is to define the implementation class which encapsulates our business logic, so we create a new class called HelloWorldServiceImpl under com.programmer.gate.
package com.programmer.gate;
import javax.jws.WebService;
@WebService(endpointInterface = "com.programmer.gate.HelloWorldService")
public class HelloWorldServiceImpl implements HelloWorldService{
public String sayHello() {
return "Hello from Programmer Gate ..";
}
}
In order to mark our class as an endpoint implementation, we:
- Let the class implements the HelloWorldService interface.
- Annotate the class with @WebService
- Define the exact path of the endpoint interface under endpointInterface attribute.
4. Publish the service
Now that we build our very simple SOAP service with the help of JAX-WS, our final step is to publish it and make it accessible to external environments.
For this purpose, we use Endpoint class provided by JAX-WS.
This class publishes our service to the built-in http server provided by the JRE without the need for any external application/web servers.
Our publisher main class looks like the following:
package com.programmer.gate;
import javax.xml.ws.Endpoint;
public class HelloWorldPublisher{
public static void main(String[] args) {
Endpoint.publish("http://localhost:9090/soap/hello", new HelloWorldServiceImpl());
}
}
Here we publish our service and make it accessible through “http://localhost:9090/soap/hello” and define HelloWorldServiceImpl to parse the requests recieved by the service.
5. Test the service
After publishing the service and running the main class, the service wsdl can be accessed through “http://localhost:9090/soap/hello?wsdl”.
It is worth to mention that in order to test the service, you should create a client application or use any web service client tools like: SoapUI.
Here below is a screenshot from SoapUI:
Summary
JAX-WS is an API used for building and consuming XML web services in Java, it was first released in Java EE5.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers