Building REST web service using Jersey
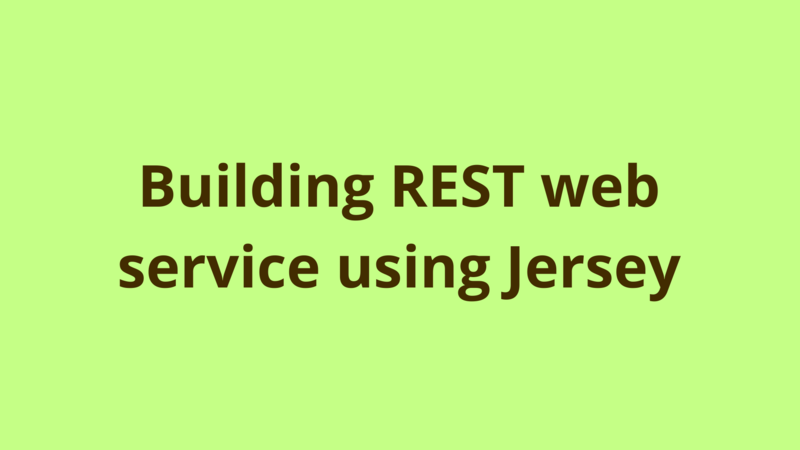
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Create maven web project
- 2. pom.xml
- 3. web.xml
- 4. MyResource.java
- 5. Deploy the service
- Summary
- Next Steps
Introduction
Since Java 5, JAX-RS specification has been announced in order to support building REST web services according to the REST architectural pattern.
JAX-RS is a set of interfaces or APIs which provides the building blocks for building REST services in java. Although you can’t build a fully functional RESTful service using these interfaces, there exists a lot of popular frameworks which implement them and can be used on top of JAX-RS to build a RESTful service.
One of the most popular JAX-RS frameworks is Jersey:
Jersey RESTful Web Services framework is open source, production quality, framework for developing RESTful Web Services in Java that provides support for JAX-RS APIs and serves as a JAX-RS (JSR 311 & JSR 339) Reference Implementation
In this tutorial, we provide a step-by-step guide for building a Jersey REST web service with Maven.
Prerequisites:
- Eclipse IDE (Mars release)
- Java 1.8
- Apache tomcat 8
1. Create maven web project
Open eclipse, then select File -> New -> Maven Project.
Keep the default selection in the first screen, then click “Next”.
In the next screen, search for “jersey-quickstart-web” archetype and select version 2.26 as the following:
In case you didn’t find the jersey archetype, then check this stackoverflow answer for how to add remote archetypes to eclipse.
Click “Next”.
In the final screen, fill the mandatory fields as the following:
- “Group Id”: denotes a unique “dot” separated group name, which is used by external projects that link to yours, this field is normally set as the company name.
- “Artifact Id”: denotes the name of the web project.
- The main package of the project is the concatenation of “Group Id” + “Artifact Id”
Click “Finish”.
Here we go, the structure of the generated project looks like the following:
2. pom.xml
By default, the jersey archetype adds the required jersey dependencies under pom.xml, so you don’t have to worry about adding them manually.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.glassfish.jersey</groupId>
<artifactId>jersey-bom</artifactId>
<version>${jersey.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<!-- use the following artifactId if you don't need servlet 2.x compatibility -->
<!-- artifactId>jersey-container-servlet</artifactId -->
</dependency>
<dependency>
<groupId>org.glassfish.jersey.inject</groupId>
<artifactId>jersey-hk2</artifactId>
</dependency>
<!-- uncomment this to get JSON support
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-binding</artifactId>
</dependency>
-->
</dependencies>
In case you want to use another Jersey version, just change the value of “jersey.version” attribute under “properties” field:
<properties>
<jersey.version>2.26</jersey.version>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
3. web.xml
The following web.xml is generated by default:
<?xml version="1.0" encoding="UTF-8"?>
<!-- This web.xml file is not required when using Servlet 3.0 container,
see implementation details http://jersey.java.net/nonav/documentation/latest/jax-rs.html -->
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<servlet>
<servlet-name>Jersey Web Application</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.programmer.gate.JerseyRestService</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Jersey Web Application</servlet-name>
<url-pattern>/rest/*</url-pattern>
</servlet-mapping>
</web-app>
Using “load-on-startup” attribute along with “org.glassfish.jersey.servlet.ServletContainer” servlet instructs the JVM to load all Jersey implementation classes on application startup. You can define the package of the implementation classes under “init-param” attribute:
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.programmer.gate.JerseyRestService</param-value>
</init-param>
In this tutorial, the JVM would try to load all jersey implementation classes defined under com.programmer.gate.JerseyRestService , you can always change this parameter as you want.
Another important attribute is the “servlet-mapping” which defines the URL of the requests to be processed by Jersey classes. In our example, all requests which are prefixed by /rest/* are processed by our service.
4. MyResource.java
By default, eclipse generates a Jersey implementation class called MyResource.java:
/**
* Root resource (exposed at "myresource" path)
*/
@Path("myresource")
public class MyResource {
/**
* Method handling HTTP GET requests. The returned object will be sent
* to the client as "text/plain" media type.
*
* @return String that will be returned as a text/plain response.
*/
@GET
@Produces(MediaType.TEXT_PLAIN)
public String getIt() {
return "Got it!";
}
}
- @Path: defines the relative path of the resource, clients access the resource through concatenating the value of the @Path annotation with the REST mapping URI, so this resource would process requests like: /rest/myresource
- @GET: defines a method which processes GET requests.
- @Produces(MediaType.TEXT_PLAIN): the meta type of the returned result, in this case it’s plain text.
5. Deploy the service
When deploying Jersey web service, always be aware of Jersey/JRE conflicts i.e. Jersey 2.26+ only works with JRE8+. If you try to run it with JRE7, the application wouldn’t start up and you’ll get “unsupported major.minor version” exception.
In our example, we deploy the web service on Tomcat 1.8/JRE8 (if you haven’t setup tomcat on eclipse, then follow this guide).
We then initiate a GET request to “MyResource” from the browser and this is the result:
That’s all, hope you find it useful ?
Summary
Since Java 5, JAX-RS specification has been announced in order to support building REST web services according to the REST architectural pattern.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers