Building REST web service using RESTEasy
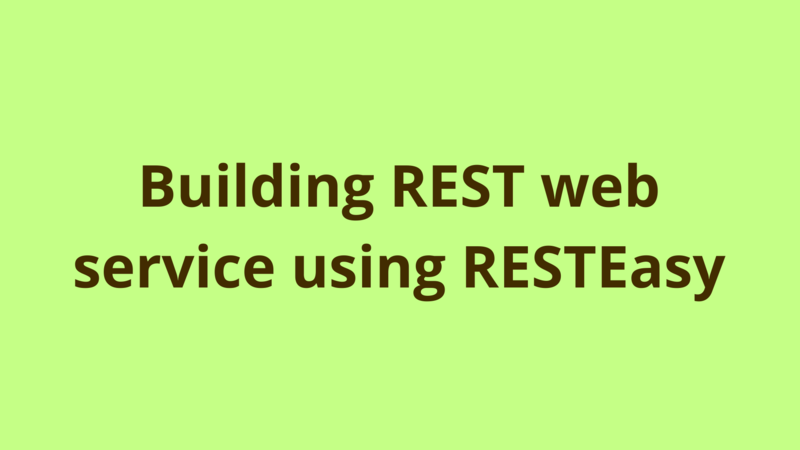
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Create maven web project
- 2. Add RESTEasy dependencies
- 3. RESTEasy Configuration
- 4. HelloWorld.java
- 5. Deploy the service
- Summary
- Next Steps
Introduction
RESTEasy is yet another popular framework which implements JAX-RS specification and is widely used for building REST web services in java.
In this tutorial, we provide a step-by-step guide for building a REST web service using RESTEasy framework, we provide different ways to configure RESTEasy with both Servlet 3.0 and Servlet 2.0.
Prerequisites:
- Eclipse IDE (Mars release)
- Java 1.8
- Apache tomcat 8
1. Create maven web project
Create a maven web project using this tutorial and name your project as RESTEasyRestService.
2. Add RESTEasy dependencies
Open pom.xml and declare the JBoss public repository as the following:
<repositories>
<repository>
<id>JBoss repository</id>
<url>https://repository.jboss.org/nexus/content/groups/public-jboss/</url>
</repository>
</repositories>
There are 2 different ways to add RESTEasy dependencies to your web application, this depends on the version of the servlet container you use.
2.1 Servlet 3.0
RESTEasy uses the ServletContainerInitializer integration interface in Servlet 3.0 containers to initialize an application and automatically scan for resources and providers, this configuration is compatible with RESTEasy 3.x versions.
In pom.xml, add the following dependencies:
<dependency>
<groupId>org.jboss.resteasy</groupId>
<artifactId>resteasy-jaxrs</artifactId>
<version>3.1.4.Final</version>
</dependency>
<dependency>
<groupId>org.jboss.resteasy</groupId>
<artifactId>resteasy-jaxb-provider</artifactId>
<version>3.1.4.Final</version>
</dependency>
<dependency>
<groupId>org.jboss.resteasy</groupId>
<artifactId>resteasy-servlet-initializer</artifactId>
<version>3.1.4.Final</version>
</dependency>
2.2 Older servlet containers
When using older servlet containers like (servlet 2.x) which are compatible with RESTEasy 2.x version, just add the following dependency to pom.xml:
<dependency>
<groupId>org.jboss.resteasy</groupId>
<artifactId>resteasy-jaxrs</artifactId>
<version>2.2.1.GA</version>
</dependency>
3. RESTEasy Configuration
The next step is to configure the application to load RESTEasy resources at the startup, again the configuration depends on the version of the servlet container you use.
3.1 Servlet 3.0
With Servlet 3.0, you should define a class which extends from JAX-RS Application class, it should look like the following:
package com.programmer.gate;
import java.util.HashSet;
import java.util.Set;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("rest")
public class HelloWorldApplication extends Application {
public HelloWorldApplication() {
}
@Override
public Set<Object> getSingletons() {
HashSet<Object> set = new HashSet<Object>();
return set;
}
}
- @ApplicationPath: defines the URL of the requests to be processed by RESTEasy classes. In our example, all requests which are prefixed by /rest/* are processed by our service.
- getSingletons(): this method returns a set of resources to be loaded on the startup of the application, if you want your application to load all RESTEasy resources then return an empty set as above.
2.2 Older servlet containers
When using older servlet containers like (servlet 2.x), you have to configure RESTEasy in web.xml as the following:
<context-param>
<param-name>resteasy.scan</param-name>
<param-value>true</param-value>
</context-param>
<context-param>
<param-name>resteasy.servlet.mapping.prefix</param-name>
<param-value>/rest</param-value>
</context-param>
<listener>
<listener-class>
org.jboss.resteasy.plugins.server.servlet.ResteasyBootstrap
</listener-class>
</listener>
<servlet>
<servlet-name>resteasy-servlet</servlet-name>
<servlet-class>
org.jboss.resteasy.plugins.server.servlet.HttpServletDispatcher
</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>resteasy-servlet</servlet-name>
<url-pattern>/rest/*</url-pattern>
</servlet-mapping>
- resteasy.scan: when set to true, the JVM scans for both @Provider and JAX-RS resource classes (@Path, @GET, @POST etc..) inside WEB-INF/classes and registers them. (Default value is false)
- resteasy.servlet.mapping.prefix: this parameter should be set if the url-pattern of the RESTEasy servlet is not /*
- ResteasyBootstrap: The ResteasyBootstrap listener is responsible for initializing some basic components of RESTeasy as well as scanning for annotation classes in your application. It receives configuration options from
elements. - servlet-mapping: defines the URL of the requests to be processed by RESTEasy classes. In our example, all requests which are prefixed by /rest/* are processed by our service.
4. HelloWorld.java
Now that we setup RESTEasy in our application, we are ready to implement our resources.
In this example, we create a very simple resource called HelloWorld.java under “src/main/java” and we expose it using the JAX-RS generic annotations as the following:
package com.programmer.gate;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("helloWorld")
public class HelloWorld {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello from programmer gate!";
}
}
- @Path: defines the relative path of the resource, clients access the resource through concatenating the value of the @Path annotation with the REST mapping URI, so this resource would process requests like: /rest/helloWorld
- @GET: defines a method which processes GET requests.
- @Produces(MediaType.TEXT_PLAIN): the meta type of the returned result, in this case it’s plain text.
5. Deploy the service
We then initiate a GET request to “helloWorld” from the browser and here is the result:
That’s all, hope you find it useful ?
Summary
RESTEasy is yet another popular framework which implements JAX-RS specification and is widely used for building REST web services in java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers