ClassNotFoundException vs NoClassDefFoundError
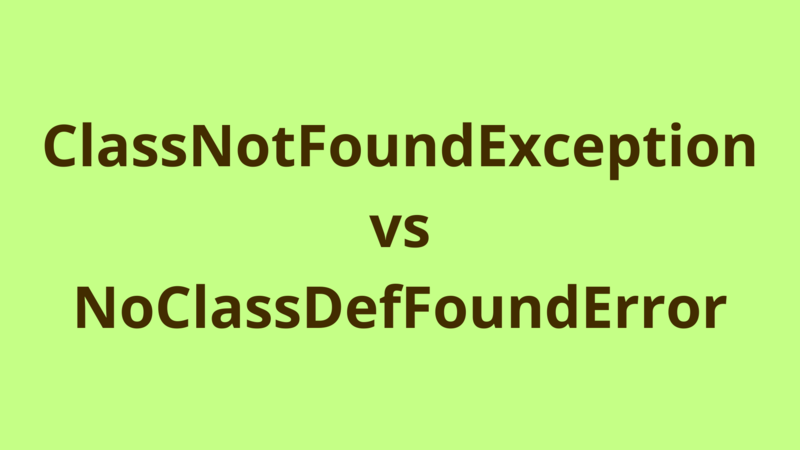
ADVERTISEMENT
Table of Contents
Introduction
ClassNotFoundException and NoClassDefFoundError occur when a required class is not found in the classpath at runtime.
In this tutorial, we resolve the conflict between these 2 exceptions and explain the different scenarios of reproducing each one of them.
1- ClassNotFoundException
As per java doc, this exception is thrown when trying to instantiate a class using one of these methods: forName(), findSystemClass(), loadClass() but the class definition doesn’t exist in the classpath.
A common scenario for reproducing this exception is to load the oracle driver before opening a connection to an oracle database:
public static Connection getConnection(String db_url,String user_name,String password)
{
Connection connection = null;
try
{
Class.forName("oracle.jdbc.driver.OracleDriver");
connection = DriverManager.getConnection(db_url,user_name,password);
}
catch (Exception e)
{
e.printStackTrace();
}
return connection;
}
It’s worth to mention that ClassNotFoundException is a checked exception, which means that eclipse or any other IDE would ask the developer to handle it explicitly at the compile time.
2- NoClassDefFoundError
This error is thrown when an application tries to use a class using new operation or through a static call but the class definition doesn’t exist in the classpath.
Ideally, IDE wouldn’t compile an application if a specific class is not found in the classpath. So this error means that the requested class was found during the compile time but missed at runtime.
Common scenarios:
- Your application uses a class A which doesn’t exist in the classpath.
- Your application uses a jar which implicitly uses a class A and class A doesn’t exist in the classpath.
- Your application uses a jar called A.jar which implicitly uses another jar called B.jar and B.jar doesn’t exist in your application’s classpath.
It’s worth to mention that this exception is not checked and can’t be handled at the compile time.
In short, both errors imply that some class is not found in the classpath and normally the missing class is displayed along with the exception stack trace. So in order to resolve the exception, you should add the missing class or jar to your application’s classpath.
Summary
In short, both errors imply that some class is not found in the classpath and normally the missing class is displayed along with the exception stack trace. So in order to resolve the exception, you should add the missing class or jar to your application’s classpath.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers