Coding Conventions
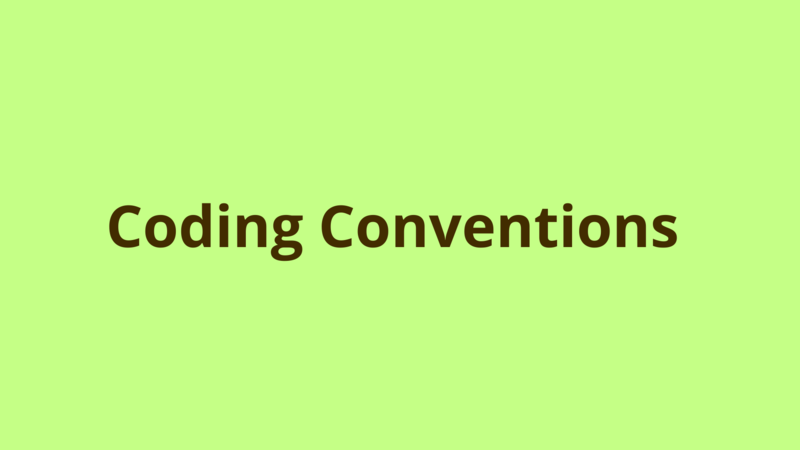
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Naming conventions
- 2. Packaging
- 3. Documentation
- 4. Logging
- 5. Code formatting
- 6. Coding techniques
- Summary
- Next Steps
Introduction
In this article, we talk about the standards and the best practices which make your code looks clean, elegant and most importantly understandable by humans. Most companies tend to apply strictly the coding conventions concept in their development process because normally the software is developed and maintained by several programmers, so it should be readable and understandable enough in order to make the life easier and simpler for the guy who maintains it in the future. In the following sections, i list the conventions that i usually follow when developing a maintainable software.
1. Naming conventions
Meaningful names help developers in understanding the business of the class/method without deeping into their details.
Use Pascal case standard for naming classes and Camel case for methods and variables. The names should reflect a business meaning or purpose , so avoid the names which doesn’t reflect the actual business behind the class/name/variable: class names like: Service.java, Bean.java, Manager.java doesn’t reflect the inner business of the class , however names like: ChargingService.java, ChargingManager.java, ChargingBean.java actually does. method name like convertToPDF() is more meaningful than convert(). variable name like document is more meaningful than d.
2. Packaging
It’s very important to divide your application into layers/packages, check this example for more details.
Divide your application into components/layers: presentation layer, service layer, business layer, data access layer, network layer. Create sub packages inside the layers and put all the related classes inside one package. The package names should reflect the business of the classes inside.
3. Documentation
Documenting the code helps a lot in modifying it later on, you have to put yourself in others’ shoes and trace comments and documentations all over your code so that you are sure that if someone else read your code, he can simply understand it.
Add java docs at the top of each class describing the purpose of the class and the responsibility of it. Add a description at the top of each method describing the business flow of the method , the input/output and the success/failure scenarios it handles. Add business and meaningful comments for complex algorithms or blocks written by yourself.
4. Logging
The first thing you look into when debugging an application is the logs, so it’s very important to trace meaningful logs as much as you can inside your application.
Add an entry log at the start of each method displaying the values of the method inputs, and add an exit log at the end of each method displaying the returning value of the method. Log all the exceptions which are thrown inside the application and never ignore any exception.
5. Code formatting
Following a common format in the whole application is very important, it makes your code look very consistent and makes its modification a lot easier.
6. Coding techniques
Following are the best coding practices for developing a clean and maintainable code:
- Program to an interface not to implementation, always assign variables to generic types in order to minimize the costs of changing their declaration to other concrete class in the future.
// instead of defining hashmap variable as the following:
HashMap<String,String> gradePerStudent = new HashMap<String,String>()
// Define it as:
Map<String,String> gradePerStudent = new HashMap<String,String>()
- Define business beans or classes specific for each module and force the module to only interact with other modules using its beans, for example, use DAOs for data access layer, DTOs for service layer and POJO beans for business layer.
- Provide toString() implementation for each bean, normally i declare it as the following:
public String toString()
{
// ReflectionToStringBuilder class from apache commons framework.
return ReflectionToStringBuilder.toString(this);
}
- Define a shared and common class for all the constants used inside the application, this makes their modification a lot easier and centralized.
- Avoid statically checking for numeric values in the code, however create a meaningful variable for each static value in the constants class.
if(action == 1)
{
// do conversion
}
// use this instead of the above
if(action == Constants.CONVERT_PDF)
{
// do conversion
}
- Avoid repeating yourself and put the duplicate functionalities into common utility classes.
- Use finally blocks to close the opened resources/connections used by the application, this is very important because most developers forget to close connections or resources after using them and this causes memory and socket leaks for the application.
- Before implementing any functionality, do some research on it and check if there exist any stable framework which supports it, this saves you much time and prevents you from reinventing the wheel.
- Avoid long classes/methods, methods should be 5-20 lines and classes should be at most 500 lines and each should have only a single responsibility, long methods can be divided into multiple private methods, and long classes can be separated into multiple classes.
- When writing if/else blocks, consider ordering the tests by likelihood of occurrence, always put the success checks at the top of the method, success scenarios usually occur a lot more than failure ones, this technique minimizes the occurrence of unexpected behaviors.
if(successCondition)
{
// success flow
}
else
{
// failure flow
}
- Avoid modifying the state of the method input, always create a local variable for each method input. The initial input value is most likely to be used in the method flow so this technique prevents it from being lost.
public void methodA(int argument1)
{
int argument1Local = argument1;
// use argument1Local instead of argument1 in the remaining flow
}
- Consider using ternary operators whenever possible instead of if/else blocks.
// instead of using the following if/else blocks
int x;
if(condition1)
{
x = 2;
}
else if(condition2)
{
x = 3;
}
else
{
x = 4;
}
// write the above nested if/else block in one line
x = condition1 ? 2 ? condition2 ? 3 : 4;
- Avoid multiple returns in simple methods , however you can use them in complicated ones, this minimizes the amount of changes when the return type is changed.
- Prefer switch-case over multiple sub nested if-else blocks.
- Use StringBuilder from apache commons for string concatenations and avoid using StringBuffer for multi threading and performance purposes, check here the differences between both of them.
- Don’t use an abstract class without abstract methods, the main purpose of using abstract classes is to centralize a common behavior and delegate some behavior to child classes. If there are no methods to be delegated then there is no need for abstraction.
- Avoid public states and expose the state of the class using getters/setters.
- Avoid static variables as possible.
- Use xml files instead of databases for light data store, xml files are more readable and more easier to be understood by human, so use them whenever there is no big data in the application.
Summary
In this article, we talk about the standards and the best practices which make your code looks clean, elegant and most importantly understandable by humans. Most companies tend to apply strictly the coding conventions concept in their development process because normally the software is developed and maintained by several programmers, so it should be readable and understandable enough in order to make the life easier and simpler for the guy who maintains it in the future. In the following sections, i list the conventions that i usually follow when developing a maintainable software.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers