Convert Java object to JSON
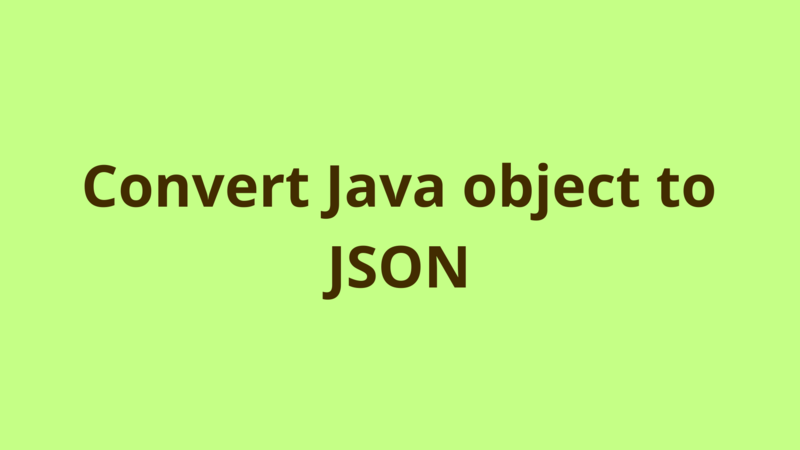
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows 2 ways for converting Java objects to JSON.
This kind of conversion is normally done via third-party libraries as itβs not supported by the JDK itself and requires a hard work to do it manually.
1- Gson
The most popular library used for converting Java objects to JSON is the Google Gson library.
Using Gson, you can get a JSON String out of an object through one line as the following:
public static String convertUsingGson(Student student)
{
Gson gson = new Gson();
String studentJson = gson.toJson(student);
return studentJson;
}
All you have to do is to include gson jar in the classpath.
P.S: Itβs worth to mention that toJson() method accepts also Hashmap, ArrayList and Arrays.
2- Jackson
Another popular library is Jackson.
In order to convert Java objects to JSON using Jackson, you have to include 3 libraries: jackson-annotations, jackson-core and jackson-databind.
Here is the way:
public static String convertUsingJackson(Student student)
{
String studentJson = "";
try
{
ObjectMapper mapper = new ObjectMapper();
studentJson = mapper.writeValueAsString(student);
}
catch(Exception ex)
{
System.out.println("Error while converting Student object to Json");
ex.printStackTrace();
}
return studentJson;
}
P.S: writeValueAsString() method accepts also Hashmap, ArrayList and Arrays.
Summary
This tutorial shows 2 ways for converting Java objects to JSON.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers