Convert JSON to Java Object
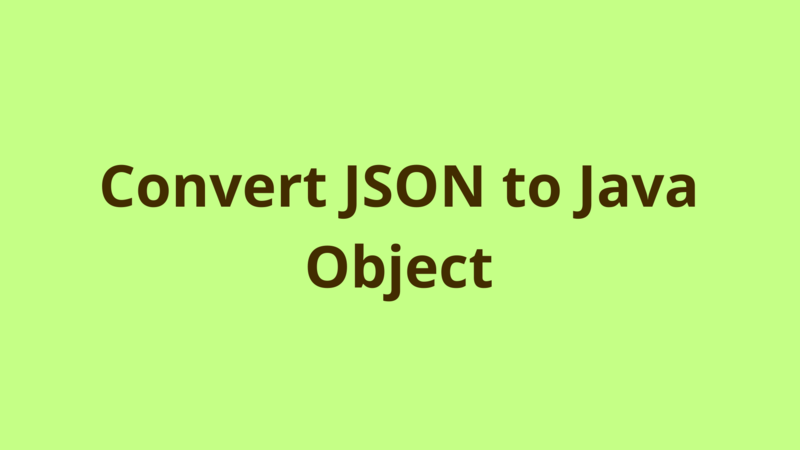
ADVERTISEMENT
Table of Contents
Introduction
This tutorials shows 2 ways for converting a JSON string to a Java object.
1- Gson
Gson is the most popular library for converting JSON string to Java objects.
With Gson, you can do the conversion in one line as the following:
public static Student convertUsingGson(String jsonStr)
{
Gson gson = new Gson();
Student student = gson.fromJson(jsonStr, Student.class);
return student;
}
Some points to be considered when using Gson:
- If the JSON string holds an invalid object attribute, then Gson implicitly ignores it.
- If the JSON string misses some object attributes, then only the attributes included in the JSON are converted, other attributes take their default values.
2- Jackson
Another common library used for converting JSON String to Java object is Jackson.
With Jackson, you do the conversion as the following:
public static Student convertUsingJackson(String jsonStr)
{
Student student = null;
try
{
ObjectMapper mapper = new ObjectMapper();
student = mapper.readValue(jsonStr, Student.class);
}
catch(Exception ex)
{
System.out.println("Error while converting JSON string to Student object.");
ex.printStackTrace();
}
return student;
}
Some points to be considered when using Jackson:
- If the POJO doesnβt contain a default constructor, then the conversion fails.
- If the JSON string holds any invalid object attribute, then the conversion fails unless you add explicitly at the POJO level this annotation:
@JsonIgnoreProperties(ignoreUnknown = true)
- If the JSON string misses some object attributes, then only the attributes included in the JSON are converted, other attributes take their default values.
- To convert a JSON array to a List of objects use:
List<Student> students = mapper.readValue(jsonStr, new TypeReference<List<Student>>(){});
- To convert a JSON String to a HashMap use:
Map<String, Object> studentsMap = mapper.readValue(jsonStr, new TypeReference<Map<String,Object>>(){});
Summary
This tutorials shows 2 ways for converting a JSON string to a Java object.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers