Deploy JAX-WS service on tomcat
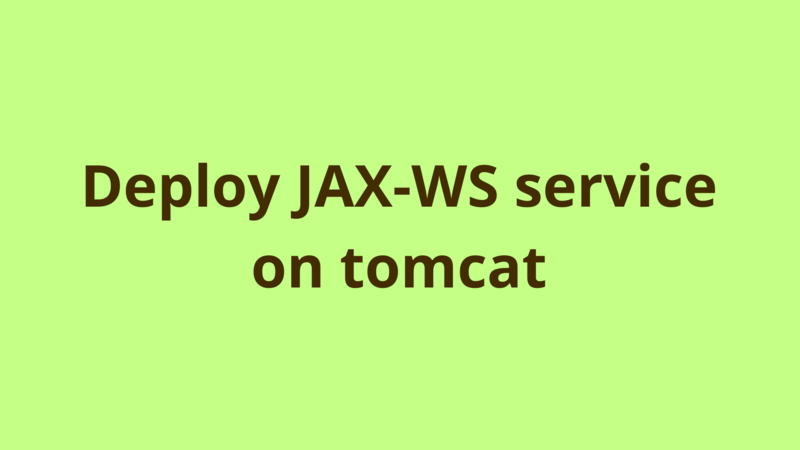
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Create Maven web project
- 2. Add JAX-WS dependency
- 3. Create service endpoint
- 4. Create service implementation
- 5. Add servlet listener to web.xml
- 6. Create sun-jaxws.xml
- 7. Test the service
- Summary
- Next Steps
Introduction
This tutorial provides a step-by-step guide on how to build and deploy JAX-WS web service on Tomcat.
Throughout this tutorial, we create a very simple SOAP web service and finally deploy it on Tomcat application server.
Prerequisites:
- Eclipse IDE (Neon release)
- Java 1.8
- Apache Tomcat 8
1. Create Maven web project
Create a maven web project using this tutorial and name your project as JAXWSSoapServiceUsingTomcat.
The structure of the generated project looks like the following:
2. Add JAX-WS dependency
After creating the web project, the first step is to add JAX-WS dependency into pom.xml, here we go:
<dependency>
<groupId>com.sun.xml.ws</groupId>
<artifactId>jaxws-rt</artifactId>
<version>2.3.0</version>
<type>pom</type>
</dependency>
3. Create service endpoint
The next step is to create an interface which exposes the different methods provided by our web service, so we create a new class called HelloWorldService under com.programmer.gate package.
package com.programmer.gate;
import javax.jws.WebMethod;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import javax.jws.soap.SOAPBinding.Style;
@WebService
@SOAPBinding(style = Style.RPC)
public interface HelloWorldService {
@WebMethod
public String sayHello();
}
4. Create service implementation
The next step is to define the implementation class which encapsulates our business logic, so we create a new class called HelloWorldServiceImpl under com.programmer.gate.
package com.programmer.gate;
import javax.jws.WebService;
@WebService(endpointInterface = "com.programmer.gate.HelloWorldService")
public class HelloWorldServiceImpl implements HelloWorldService{
public String sayHello() {
return "Hello from Programmer Gate ..";
}
}
For more details about the different JAX-WS annotations used in the above classes, check our previous complete tutorial.
5. Add servlet listener to web.xml
Now that we create the service interface and implement the business details of the service.
The next step is to define the JAX-WS servlet listener under web.xml and inform tomcat to automatically load and map our service on startup. Here we go:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems,
Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/j2ee/dtds/web-app_2_3.dtd">
<web-app>
<listener>
<listener-class>
com.sun.xml.ws.transport.http.servlet.WSServletContextListener
</listener-class>
</listener>
<servlet>
<servlet-name>hello</servlet-name>
<servlet-class>
com.sun.xml.ws.transport.http.servlet.WSServlet
</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>hello</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
<session-config>
<session-timeout>120</session-timeout>
</session-config>
</web-app>
6. Create sun-jaxws.xml
The final step is to define sun-jaxws.xml file.
This file describes the different endpoints defined under your application and it’s required when deploying your service on non-Java EE5 servlet containers. Its role is to tell web.xml to which endpoint a servlet request must be dispatched.
So we create sun-jaxws.xml under WEB-INF as the following:
<?xml version="1.0" encoding="UTF-8"?>
<endpoints
xmlns="http://java.sun.com/xml/ns/jax-ws/ri/runtime"
version="2.0">
<endpoint
name="HelloWorldService"
implementation="com.programmer.gate.HelloWorldServiceImpl"
url-pattern="/hello"/>
</endpoints>
Here we’re saying that every request corresponding to /hello servlet is handled by HelloWorldService endpoint which is implemented by HelloWorldServiceImpl.
7. Test the service
Now that out application is ready to be published on Tomcat. After publishing it and starting tomcat, you can access the service wsdl through “http://localhost:9090/soap/hello?wsdl” knowing that 9090 is the tomcat port.
It is worth to mention that in order to test the service, you should create a client application or use any web service client tools like: SoapUI.
Here below is a screenshot from SoapUI:
Summary
This tutorial provides a step-by-step guide on how to build and deploy JAX-WS web service on Tomcat.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers