Deploy Spring Boot application on external Tomcat
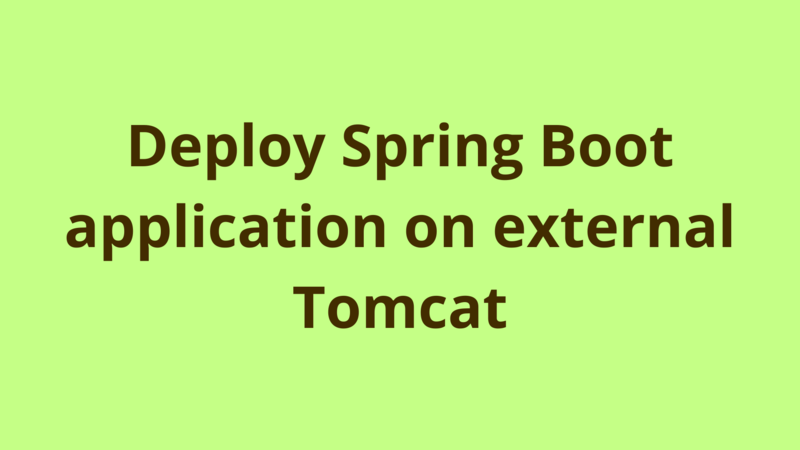
ADVERTISEMENT
Table of Contents
Introduction
Spring Boot provides an embedded servlet container which makes it very easy to setup and deploy web applications, by default all Spring Boot applications are exported as a runnable jar file and developers would only need to run this jar file on a java environment without the need to worry about installing and setting up servlet containers.
However, the embedded server may not be suitable for all production environments especially when the infrastructure is already set up and administrators need to have full control on the server, in this situation Spring Boot applications must run on external and separate servlet containers.
In this tutorial, we provide the required steps in order to deploy a Spring Boot web application on external Tomcat.
In order to understand what is Spring Boot and how do we create a Spring Boot web application, refer to “Introducing Spring Boot” tutorial.
Step #1
Add the following dependency to pom.xml in order to tell Spring Boot not to use its embedded Tomcat.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
Step #2
Change the packaging property to war in pom.xml.
<properties>
<packaging>war</packaging>
</properties>
Step #3
Replace your initializer class with the following:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.support.SpringBootServletInitializer;
@SpringBootApplication
public class Application extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(Application.class);
}
public static void main(String[] args) throws Exception {
SpringApplication.run(Application.class, args);
}
}
Then define it as a starting class in pom.xml:
<properties>
<start-class>com.programmer.gate.Application</start-class>
</properties>
Here is a full working pom.xml:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.programmer.gate</groupId>
<artifactId>SpringBootRestService</artifactId>
<version>1.0</version>
<packaging>war</packaging>
<properties>
<start-class>com.programmer.gate.Application</start-class>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.8.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
Step #4
Now that your Spring Boot application is ready to be deployed on external Tomcat, in order to export a war file from your application:
- Right click pom.xml -> run-as -> Maven install
- Maven generates a war file inside target folder
That’s it, for clarifications please leave your thoughts in the comments section below.
Summary
Spring Boot provides an embedded servlet container which makes it very easy to setup and deploy web applications, by default all Spring Boot applications are exported as a runnable jar file and developers would only need to run this jar file on a java environment without the need to worry about installing and setting up servlet containers.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers