Download file from a Web Application using Servlet
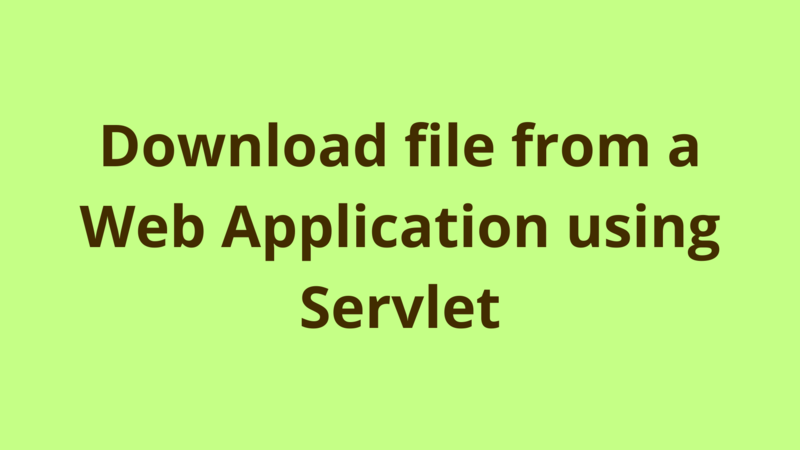
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows how to download a file from a web application using Servlet.
A typical task in most web applications is to download files stored at the server side to the client’s machine, here below we provide the common way of doing this with Servlet.
1- Download File Servlet
Below is an example of a typical Servlet which downloads files to the client’s machines.
@WebServlet("/download")
public class DownloadFileServlet extends HttpServlet{
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException{
String fileName = "pdf-sample.pdf";
FileInputStream fileInputStream = null;
OutputStream responseOutputStream = null;
try
{
String filePath = request.getServletContext().getRealPath("/WEB-INF/resources/")+ fileName;
File file = new File(filePath);
String mimeType = request.getServletContext().getMimeType(filePath);
if (mimeType == null) {
mimeType = "application/octet-stream";
}
response.setContentType(mimeType);
response.addHeader("Content-Disposition", "attachment; filename=" + fileName);
response.setContentLength((int) file.length());
fileInputStream = new FileInputStream(file);
responseOutputStream = response.getOutputStream();
int bytes;
while ((bytes = fileInputStream.read()) != -1) {
responseOutputStream.write(bytes);
}
}
catch(Exception ex)
{
ex.printStackTrace();
}
finally
{
fileInputStream.close();
responseOutputStream.close();
}
}
}
A brief description about the above sample code:
- Our Servlet is accessible through “/download” url, we make use of @WebServlet annotation provided by Servlet 3.0.
- A download file action is considered as a GET request, so we define our logic inside doGet() method.
- In this example, we’re downloading a static file called “pdf-sample.pdf” located under WEB-INF/resources. You can use the above code to download any file of any type.
- Reading a resource inside a web application is done through passing the relative path of the file to getRealPath() method of ServletContext.
- The content type attribute of the response is used to inform browser what type of file to download, if the content type is not set, then the file would be downloaded without extension.
- The content disposition attribute of the response is used to inform browser whether to render the response or download it, it can be of 2 values: inline or attachment.
- inline: browser renders the response as a normal html page.
- attachment: browser treats the response as a file and downloads it directly to the client’s machine. *At the end, the required file is written to the OutputStream of the response using InputStream.
Summary
This tutorial shows how to download a file from a web application using Servlet.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers