Eclipse – Generate a SOAP client from WSDL
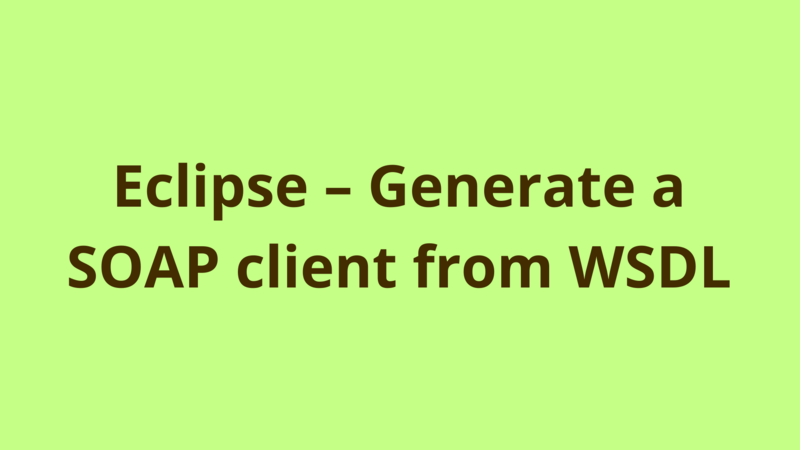
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Create a stand-alone project
- 2. Create a new web service client
- 3. Client.java
- Summary
- Next Steps
Introduction
This tutorial provides a step by step guide on how to generate a SOAP client from WSDL using Eclipse.
1. Create a stand-alone project
If you’re going to generate the SOAP client classes inside an existing project, then you can skip this step.
If you’re starting from scratch, then create a new standard stand-alone project and name it as WebServiceClient, we will later use this project when generating our SOAP client classes.
2. Create a new web service client
Select the project that we create in step 1 by clicking on the project name. Then go to File -> New -> Other and search for “Web Service Client”:
Click Next.
In the next page, put the URL of your WSDL, if you have the WSDL locally on your machine, you can copy it under your project and locate it using browse.
For testing purposes, we’re going to use a sample online calculator service that is located under “http://www.dneonline.com/calculator.asmx?wsdl“.
Keep all the settings as is then click Finish.
3. Client.java
After clicking on “Finish”, the client classes will be generated under your selected project using their own package as the following:
The last step is to consume the web service using these generated classes, in order to do so, we create a main class called Client.java which simply calls the add() function of the calculator service in order to add 2 numbers:
package org.tempuri;
public class Client {
public static void main(String[] args){
try
{
CalculatorLocator locator = new CalculatorLocator();
CalculatorSoapStub stub = (CalculatorSoapStub)locator.getCalculatorSoap();
int result = stub.add(2, 4);
System.out.println("Result is: " + result);
}
catch(Exception ex)
{
ex.printStackTrace();
}
}
}
As noticed, to consume a SOAP service, follow the below order:
- Create an instance of the Locator.
- Get an instance of the Stub through the Locator.
- Finally, call the requested method through the Stub.
Summary
This tutorial provides a step by step guide on how to generate a SOAP client from WSDL using Eclipse.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers