git clean | Delete untracked files in Git
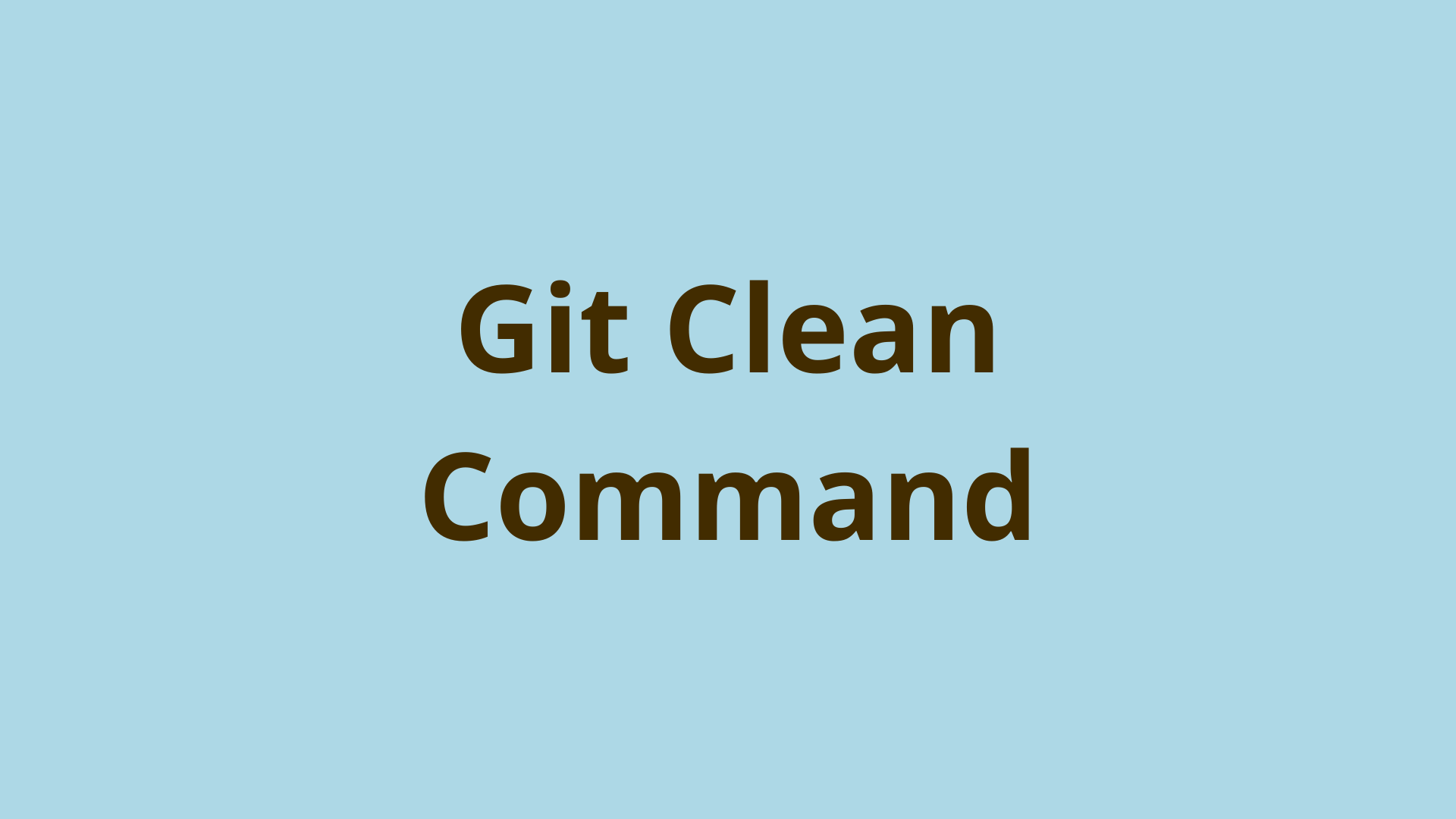
ADVERTISEMENT
Table of Contents
- Introduction
- What is the git clean command?
- What is an untracked file in Git?
- fatal: clean.requireForce defaults to true and neither -i, -n, nor -f given; refusing to clean
- Git clean with no untracked files
- Dry run with git clean -n
- Force cleaning with git clean --force
- Is Git clean recursive?
- Git clean interactive mode with git clean -i
- Clean ignored files with git clean -x
- Does git clean delete files?
- Does git clean remove stash?
- Can you undo git clean?
- Git clean config clean.requireForce
- How do I clean up Git?
- Next steps
- References
Introduction
Once in a while Git users will run into a situation where many unneeded untracked files are present within a Git repository working directory.
This can occur if files were accidentally copied to that location, if build files names and extensions are not included in the .gitignore
file, or for a variety of other reasons.
Regardless of how they got there, Git provides a quick and easy command to delete all untracked files in one fell swoop.
In this article, we'll explain how to use the git clean command to remove untracked files from your Git working directory.
What is the git clean command?
The git clean
command allows you to efficiently clean up untracked files in your Git repo. It comes with a variety of command-line flags to help you git clean untracked files using different methods and in different situations.
What is an untracked file in Git?
In Git, an untracked file is a file in your repo that Git doesn't know about yet. This is because git add has not been used on that file yet. In addition, ignored files in the .gitignore file are usually untracked as well and Git will not report them when running Git status.
The simplest way to list Git untracked files is using the git status command:
$ git status
On branch main
Untracked files:
(use "git add <file>..." to include in what will be committed)
newfile.txt
From the git status output you can see that the file newfile.txt
is in the "Untracked files" section.
fatal: clean.requireForce defaults to true and neither -i, -n, nor -f given; refusing to clean
By default, if you just run git clean
on its own in your repo with no flags, you'll get this fatal error message fatal: clean.requireForce defaults to true and neither -i, -n, nor -f given; refusing to clean:
$ git clean
fatal: clean.requireForce defaults to true and neither -i, -n, nor -f given; refusing to clean
The reason for this is that there is a default Git clean configuration setting called clean.requireForce
that prevents Git from deleting untracked files without the user specifying additional flags to the git clean command.
This protects the user from accidentally deleting all their untracked files if they are just testing git clean
.
Git clean with no untracked files
If there are no untracked files in your current directory (or in your project working directory as a whole), Git clean won't display any output at all.
Dry run with git clean -n
The safest way to use the git clean command is to start by doing a dry run. This will show you exactly which files Git would delete, without doing anything you might regret.
Let's imagine we have a brand new repository with one new untracked file in it called newfile.txt
.
You can do a git clean dry run using the git clean --dry-run or git clean -n flag:
$ git clean -n
Would remove newfile.txt
If we add a second untracked file, both show up in the git clean output:
$ git clean -n
Would remove newfile.txt
Would remove newfile2.txt
If we use the git add command to add newfile.txt
to the Git staging area, it is now a tracked file and will no longer show up in the git clean dry run output:
$ git clean -n
Would remove newfile2.txt
Force cleaning with git clean --force
Now that we know what Git would delete by using the --dry-run or -n flag, we can rest assured that only the untracked file newfile2.txt
will be removed when cleaning Git.
This can be executed by using the git clean --force or git clean -f flag:
$ git clean -f
Removing newfile2.txt
Be careful when force cleaning Git files since it might not be so easy to retrieve an untracked file's content once removed.
Is Git clean recursive?
By default, git clean is NOT recursive and will only remove untracked files in the current directory. However, the git clean -d flag can be used to make git clean recursive. In this case all of your nested repo subdirectories will be checked for untracked files.
If you don't want to use the -d
flag, you can browse into a specific directory and run git clean within it to target files in that directory.
Another option is to explicitly specify the directory to clean in the form:
$ git clean -n <path-to-directory-1> <path-to-directory-2>
Multiple directories can be cleaned simultaneously as in the example above.
Git clean interactive mode with git clean -i
There is a cool option available with Git clean that not many users are aware of - interactive mode.
Git clean interactive mode provides you with a selection interface in the command line to specifically tell Git which untracked files to clean.
Git clean's interactive mode is enabled using the git clean -i flag:$ git clean -i
Would remove the following items:
newfile.txt newfile2.txt test/newfile4.txt
*** Commands ***
1: clean 2: filter by pattern 3: select by numbers 4: ask each 5: quit 6: help
What now>
First, this displays the untracked files Git could remove - not that interactive mode will recursively display all untracked files within nested subdirectories even if the -d
flag is not specified. Also note that you do not need to specify the force -f
flag when running git clean interactively.
Next, the prompt displays six options to choose from:
- clean: clean out the listed untracked files
- filter by pattern: Selectively ignore files from cleaning that match certain patterns
- select by numbers: Select specific files to clean by name and number
- ask each: Iterate through all untracked files and ask y/N whether each should be deleted
- quit
- help
You can type of these numbers after the What now>
prompt and press -n
flag along with the interactive flag -i
until you get used to it.
Clean ignored files with git clean -x
By default, git clean won't touch any untracked files that are ignored in the .gitignore
file. However, you can change that by using the git clean -x flag:
$ git clean -x -i
This can be useful for quickly getting rid of ignored build files that are included in the .gitignore file and might be otherwise annoying to delete.
If you want to ONLY delete ignored untracked files and not others, you can capitalize the -X as follows:
$ git clean -X -i
Note that we supplied the -i
flag as protection so you control exactly what will be deleted, however if you have done it a few times and know it's safe, you can use the -f
flag instead.
Does git clean delete files?
Yes, git clean deletes untracked files based on the flags supplied when running the command. As mentioned, if no flags are supplied git clean throws an error by default, and using the dry run flag -n will list files to be deleted without actually removing them.
Does git clean remove stash?
No git clean will not touch the stash. If you want to remove your stashed items, you can use git stash clear
.
Can you undo git clean?
Most of the time, there is no good way to restore deleted untracked files in Git. That is why you need to be very careful when removing untracked content in general, since it is one of the few ways to actually lose work with Git.
Git clean config clean.requireForce
Git has many configuration settings that are initially set to sane default values for user convenience or protection. Git stores local config settings in the file .git/config
and user level Git configs in the ~/.gitconfig
file.
If you want to disable Git's builtin protection for the git clean command, you can set add the following setting adjustment to your git config file:
clean.requireForce = false
However, this is not recommended since it can lead to accidental file deletion - please take care when changing the default clean.requireForce Git configuration.
How do I clean up Git?
So to summarize, the Git clean command is typically used by doing a dry-run with the -n
flag, or simply using the interactive mode -i
flag right off the bat.
Once you are sure that the resulting operation, based on Git's output, will not accidentally remove anything unintended, you can rerun Git clean with the -f
flag to actually perform the deletion.
We covered various scenarios and command line options in this article, which should be everything you need to get going with the git clean
command!
Next steps
If you're interested in learning more about how Git works under the hood, check out our Baby Git Guidebook for Developers, which dives into Git's code in an accessible way. We wrote it for curious developers to learn how Git works at the code level. To do this, we documented the first version of Git's code and discuss it in detail.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
References
- https://git-scm.com/docs/git-clean
Final Notes
Recommended product: Decoding Git Guidebook for Developers