How to Remove Local Untracked Files in Git Working Directory
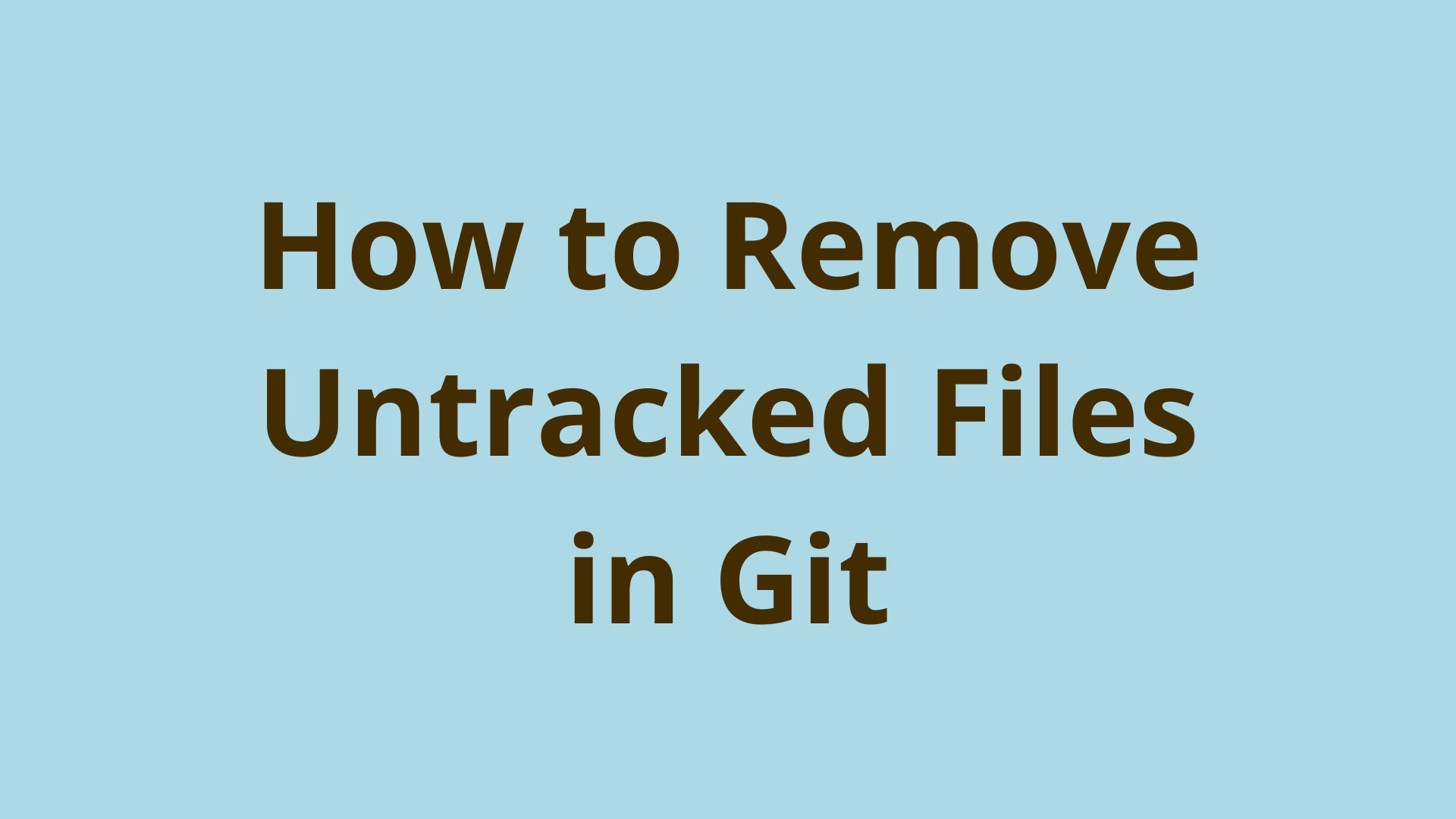
ADVERTISEMENT
Table of Contents
- What are Git Untracked Files?
- Types of Untracked Files in Git
- How Do I Delete Untracked Files in Git?
- Using .gitignore to Handle Untracked Files in Git
- Manually Delete Untracked Files in Git
- git clean – The Git Command for Removing Untracked Files
- Can You Undo Git Clean?
- Can You Git Stash Untracked Files?
- Does git reset Remove Untracked Files?
- Summary
- Next Steps
- References
What are Git Untracked Files?
Git is a version control system used for tracking code changes in software development projects. However, not all files are officially tracked by Git. An untracked file is a file that exists in Git's working directory that hasn't been added to the staging area or committed yet.
Oftentimes when running the git status
command, Git will report untracked files as follows:
> git status
git status
On branch master
Your branch is up to date with 'origin/master'.
Untracked files:
(use "git add <file>..." to include in what will be committed)
filename.ext
We tell Git to track files using the git add
and git commit
commands. This tells Git to record the current content (or changed content) of these files in the repository, and to take notice when we make future modifications to these files in the working directory.
In fact, every file in a Git repository begins its life as an untracked file.
Most untracked files will be staged and committed. Others will simply be ignored by including them in the .gitignore
file. However, some files and directories will remain untracked, and in certain instances it can be useful to delete these untracked files from your Git repo.
In this article, we'll discuss different types of untracked files, whether you should remove them, and some different methods for dealing with them including .gitignore, manual deletion, and git clean
.
Types of Untracked Files in Git
Here are some examples of types of untracked files in Git:
- A new file containing local code that you developed for the codebase
- A local project configuration file created by your IDE, such as a
.project
file for Eclipse - A collection of local dependency files, such as the
/node_modules/
directory in Node.JS - Local build files such as the
/target/
directory in Java projects - Local OS files such as the
.DS_Store
file in MacOS
The action that we want to take on an untracked file often depends on the type of file we are dealing with.
Now that we've seen the common types of untracked files, let's look at some options for handling them.
How Do I Delete Untracked Files in Git?
Here is a list of ways to handle untracked files in Git, which will prevent them from showing up in the "untracked files" section when running the git status command:
- The untracked file is added to Git staging area using
git add
and committed usinggit commit
- The untracked file name, path, or pattern is included in the
.gitignore
file - The untracked file is manually deleted from the filesystem (or moved out of the Git repo)
- The untracked file is deleted using the
git clean
command - The untracked file is saved using the
git stash
command - The untracked file is deleted using the
git reset --hard
command
We'll cover each of these situations in the sections below, and mention which type of files from the previous section each applies to.
Using .gitignore to Handle Untracked Files in Git
Oftentimes, we get sick of seeing a long list of untracked files in the git status
output, but we don't want to commit or delete those files.
This applies to several untracked files types mentioned above, including local project configuration files specific to the IDE in use, local dependencies, local build files, and local OS files.
We can tell Git to ignore these files without deleting them, via the hidden .gitignore file. Specifying files and directories in the .gitignore
file tells Git you don't want these files tracked, which will prevent them from showing up when you run git status
and also prevent them from being added or committed accidentally.
It's important to note that if a file is committed and later added to the .gitignore
file, this file will continue to be tracked by Git. In this case, running git rm --cached filename.ext
will prevent Git from tracking files that were previously committed, and enable the new .gitignore
entry to take effect.
Remember that the .gitignore
file itself does need to be added and committed to Git, which is kind of meta!
Manually Delete Untracked Files in Git
Certain types of untracked files can simply be deleted manually. This works best for one-off deletions of files that won't automatically be recreated by your IDE, local build process, or operating system. These files can be removed from your file system explorer or from the command-line.
In MacOS, Windows, and GUI Linux environments, simply browse into your project root and delete the untracked files as needed. If desired you can click and drag the untracked file out of your project folder.
If you want to use the command-line in MacOS or Linux, you can remove files manually using rm path/to/filename.ext
or move them to your Desktop using mv filename.ext ~/Desktop/
.
In Windows, you can do the same from the CMD terminal using del /f filename.ext
or move filename.ext destination_path
.
Although removing untracked files manually is possible, Git helps streamline this process by providing the git clean
command to delete untracked files.
git clean – The Git Command for Removing Untracked Files
Git provides the git clean command to efficiently remove untracked files from your repo.
A simple git clean
command with no options will throw an error:
> git clean
fatal: clean.requireForce defaults to true and neither -i, -n, nor -f given; refusing to clean
Since git clean
will permanently delete untracked project files or directories, Git requires you to include one or more options to confirm your intent to delete. Here’s a look at commonly used options for git clean
:
-d
Specifies that you would like to recurse into untracked directories.-f
Tells Git that you are ready and aware that untracked files will be permanently deleted.-n
Gives you a safe dry run, showing you what would be done if you ran the command.-q
Will run the command without reporting any feedback unless there is an error.-X
Removes only files that are specified in your .gitignore file.
When removing untracked files from your project with git clean, you should always start with a dry-run.
Here’s a simple example of a dry-run that simulates the removal of untracked files:
> git clean -n
Would remove filename.ext
If you’d like to simulate the removal of untracked directories along with your files, git clean
can do this recursively like so:
> git clean -n -d
Would remove filename.ext
Would remove testdir/
If everything looks good, and you're ready to permanently remove your untracked files, simply replace the -n
option with -f
.
To remove all untracked files only:
> git clean -f
Removing filename.ext
Remove all untracked files and directories:
> git clean -f -d
Removing filename.ext
Removing testdir/
Using git clean
to delete all files specified in .gitignore
can be done with git clean -f -X
. Directories can be included using git clean -f -d -X
.
Note that by design, git clean -f
ignores untracked files and directories specified in .gitignore.
Can You Undo Git Clean?
No, you cannot simply undo a completed git clean command as the files were deleted from your filesystem.
Keep in mind that files removed using git clean cannot be easily restored. Always consider this before running the command.
If you're hesitant to permanently delete your untracked files, consider stashing them as we'll now discuss.
Can You Git Stash Untracked Files?
If the idea of permanent deletion scares you, consider using git stash instead, which allows you to save untracked files (along with uncommitted file modifications) to a temporary "stash" for later use.
Although git stash
has many use-cases, it's an easy and safe way to clean untracked files from your Git working directory. To stash untracked files in Git, use the command git stash -u
.
And if at any point you'd like to restore them to your working directory, simply run git stash pop
. Note that if you stash multiple times, you can either "pop" them one-by-one or use git stash pop stash@{x}
(where x
is the index of the desired stash entry) to specify the stash to pop.
Does git reset Remove Untracked Files?
You need to be careful using git reset
, because in certain cases it can delete untracked files.
The git reset
command is used to move git HEAD to a different commit, while optionally removing staged changes and cleaning out the working directory. This is most commonly used to restore a branch to a previous state.
By default, git reset doesn't impact untracked files. However, in certain cases, git reset
can remove untracked files.
Using git reset
without options is equivalent to supplying the default flag --mixed
. This could unstage changes (including unstaging a newly staged file back to an untracked file in the working tree), but they will still be present in the working directory.
The command git reset --soft
will not affect the staging area or working tree, and therefore can be used without concern of deleting any untracked files.
On the other hand, git reset --hard
will reset your working directory to the specified commit, unstage any changes in the pipeline, and remove untracked files.
In a nutshell, doing a hard git reset can remove untracked files.
Summary
In this article, we reviewed what untracked files are in Git, the types of untracked files, and several methods to remove them. We discussed using .gitignore to hide untracked files that you don't want to commit, when you might want to manually delete Git untracked files, and using the git clean command.
We saw that git clean cannot be easily undone, and finished up by covering the impact of git stash and git reset on untracked files.
Next Steps
If you're interested in learning more about how Git works under the hood, check out our Baby Git Guidebook for Developers, which dives into Git's code in an accessible way. We wrote it for curious developers to learn how Git works at the code level. To do this we documented the first version of Git's code and discuss it in detail.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
References
- Git SCM - https://git-scm.com/docs/gitignore
- Oracle Docs - https://docs.oracle.com/cd/E88353_01/html/E37839/git-clean-1.html
Final Notes
Recommended product: Decoding Git Guidebook for Developers