Git Status Command | Uses, Applications & Related Commands
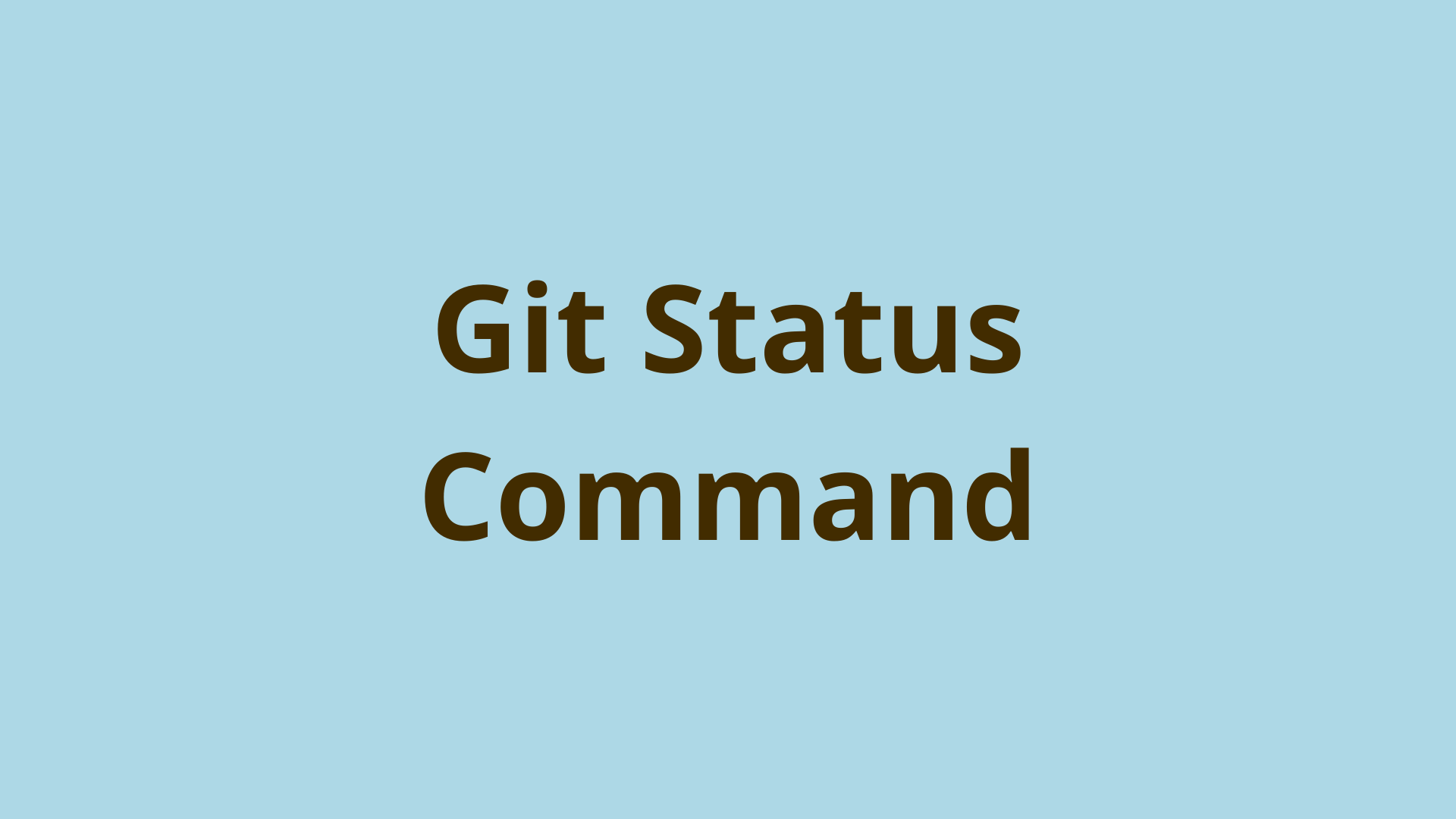
ADVERTISEMENT
Table of Contents
- What does Git status do?
- What is the output of the Git status command?
- When should I use Git status?
- Which files have I moved to the Git staging area?
- Git status options
- git status -s, --short
- git status --show-stash
- git status --renames, --no-renames
- Summary
- Next Steps
- References
What does Git status do?
One of the first commands you'll use when learning Git is git status
.
The git status command provides useful information about the current state of your Git repository. It outputs the active branch, remote sync status, and a list of modified files in the working directory and staging area. It doesn't include details of your project's commit history.
By default, running git status
in your project displays the following details:
- Which local branch is currently checked out in the working directory
- Whether that branch is in sync with it's remote tracking branch, which can help you know to either run
git push
orgit pull
- Changes to be committed: Lists tracked files moved to the staging area with
git add
- Changes not staged for commit: Lists tracked files containing local modifications in the working directory
- Untracked files: Lists untracked files in the working directory that are not included in the
.gitignore
file
In this article, we'll discuss each of these points in-depth so you understand how to use this information. We'll also cover when to use git status, and provide some git status examples. Finally we'll go over some lesser-known command-line options for git status usage and mention some related Git commands.
What is the output of the Git status command?
If you run git status
outside of any Git repository, you'll probably get the following fatal Git error:
> git status
fatal: not a git repository (or any of the parent directories): .git
So make sure you use cd <your-project>
to navigate into your development project before running git commands.
Running the command git status
provides the following output by default:
(1) On branch master
(2) Your branch is up to date with 'origin/master'.
(3) Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: filename1.ext
(4) Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: filename2.ext
(5) Untracked files:
(use "git add <file>..." to include in what will be committed)
filename3.ext
As you can see, we added the numbers (1) - (5) to the output corresponding to the bullet points in the previous section. Note that the current Git branch (1) and branch syncing status (2) will show up any time you execute git status.
Also note that Changes to be committed (3), Changes not staged for commit (4), and Untracked files (5), will only be displayed if a file exists and meets the conditions for that section.
When should I use Git status?
Git status is your best friend when you ask yourself any of the following questions.
Which Git branch am I on?
The first piece of data git status tells you is what branch you're on, such as On branch master
or On branch dev
or On branch featureBranch1
.
This tells you what branch name is currently checked out in the working directory. This is also called the active branch.
You can double-check this by running the git branch
command. Git branch will list the local branches in your Git repository, and put an asterisk next to the currently active branch:
> git branch
* master
dev
featureBranch1
Is my Git branch in sync with the remote branch?
The second piece of info git status provides is the active branch syncing status with its remote tracking branch.
A remote tracking branch is local copy of each of your branches that Git creates behind the scenes. This helps Git manage syncing branches when you connect to a remote repository via git fetch, git pull, or git push.
Most of the time, git status will tell you Your branch is up to date with 'origin/master'.
. This means your active branch is in-sync with its remote tracking branch.
If you make one new commit locally and then run git status
again, git will report Your branch is ahead of 'origin/master' by 1 commit.
This simply tells you that you have 1 new local commit that hasn't been pushed to the remote repository with git push
yet. After running git push
or git push origin <branch-name>
, git status will again tell you your branch is up to date with its remote tracking branch.
Which files have I moved to the Git staging area?
The third datapoint that git status reports is a list of "Changes to be committed". This is a list of files that have been added to the staging area using the git add <filename.ext>
command:
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: filename1.ext
In this example, git status tells us that filename1.ext
has local modifications that have been staged and are ready to be included in the next commit using the git commit
command.
Git also provides a suggestion to run the git restore --staged <file>
command to unstage the file if desired. This will remove it from the staging area and move it back to the working directory. Don't worry, your local file changes won't be lost.
Which files have I made local changes to?
The fourth section that git status displays is "Changes not staged for commit". This is a list of tracked Git files that have local modifications, but have not been staged:
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: filename2.ext
Git also suggests two additional commands that could be useful in this scenario. The git add <file>
command can be used to stage the file changes for the next commit.
The git restore <file>
command can be used to discard local changes to the file. This essentially resets the file to its state in the previous commit. Note that running git restore
this way on a non-staged file WILL cause your changes in the file to be lost, so be careful.
Are there any untracked files in my Git working directory?
The fifth and final section that git status provides is "Untracked files":
Untracked files:
(use "git add <file>..." to include in what will be committed)
filename3.ext
This list includes Git untracked files, which are usually new code files that haven't been added with git add
yet. The output suggests running git add <file>
if this file should be staged for the next commit.
By default, git status won't display untracked files that are ignored in the .gitignore file.
Note that just because a file is untracked, doesn't mean you want to commit it. Many types of files related to the local environment usually aren't committed. These include local dependencies such as the node_modules/
directory, local OS files like MacOS .DS_Store
, local build files like the Java target/
directory, and more. These are usually ignored by adding them to the hidden .gitignore
file.
Git status options
Now we'll discuss some useful git status
command-line options that you may not be aware of.
git status --ignored
The --ignored
option tells Git status to display an additional section showing files that Git ignores based on the .gitignore
file:
> git status --ignored
On branch master
Your branch is up to date with 'origin/master'.
...
Ignored files:
(use "git add -f <file>..." to include in what will be committed)
.DS_Store
.classpath
.project
.settings/
target/
The example above shows a set of files in a Java Eclipse project that are typically ignored in Git.
git status </directory/filename.ext>
One or more directories or filenames can be supplied after the git status
command which will only list matching files in the output:
> git status filename1.ext filename2.ext
On branch master
Your branch is up to date with 'origin/master'.
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: filename1.ext
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: filename2.ext
Note that in the example above, only info related to the filenames listed in the command is displayed.
git status -s, --short
The git status -s
or git status --short
options can be used to show a compact version of the command's output:
> git status -s
MM filename1.ext
M filename2.ext
?? filename3.ext
Staged files are prefixed with the symbol MM
, unstaged changes are prefixed with just one M
, and untracked files are indicated using ??
symbol.
git status -b, --branch
The git status -s -b
or git status --short --branch
option tells Git to display the branch and tracking info even when the command is run using the shortened (-s
) format:
> git status --short --branch
## master...origin/master
MM filename1.ext
M filename2.ext
?? filename3.ext
Note that the branch and tracking info is a short-form version due to the presence of the --short
flag in addition to --branch
.
git status --show-stash
The git status --show-stash
option displays the number of stash entries that are currently in the Git stash:
> git status --show-stash
...
Your stash currently has 4 entries
This line is displayed at the end of the output.
git status --porcelain
The git status --porcelain
option provides basically the same short-form output as git status -s
, but the --porcelain
flag guarantees that the output format will remain stable across different versions of Git.
This can be useful if you need to use the output of git status in scripts or for automation, since you can be sure the format won't chance in the future.
git status -v, --verbose
The git status -v
option displays a textual representation of the changes in the Git files in addition to the filenames. The essentially amounts to adding the output of git diff
to the output.
git status --renames, --no-renames
By default, Git will automatically detect file renames for tracked files and display those changes when you run Git status. The git status --no-renames
option disables rename tracking in the command output. This means that Git will report a rename as a tracked file deletion and a new untracked file.
We recommend learning the git mv command to rename files in Git.
Summary
In this article, we discussed the purpose and usage of the git status
command. We covered when to use Git status, what it displays by default, and how to use some lesser-known options to enhance your development experience.
Next Steps
If you're interested in learning more about how Git works under the hood, check out our Baby Git Guidebook for Developers, which dives into Git's code in an accessible way. We wrote it for curious developers to learn how Git works at the code level. To do this we documented the first version of Git's code and discuss it in detail.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
References
- Git SCM - https://git-scm.com/docs/git-status
Final Notes
Recommended product: Decoding Git Guidebook for Developers