Git Config | Setup Git Environment
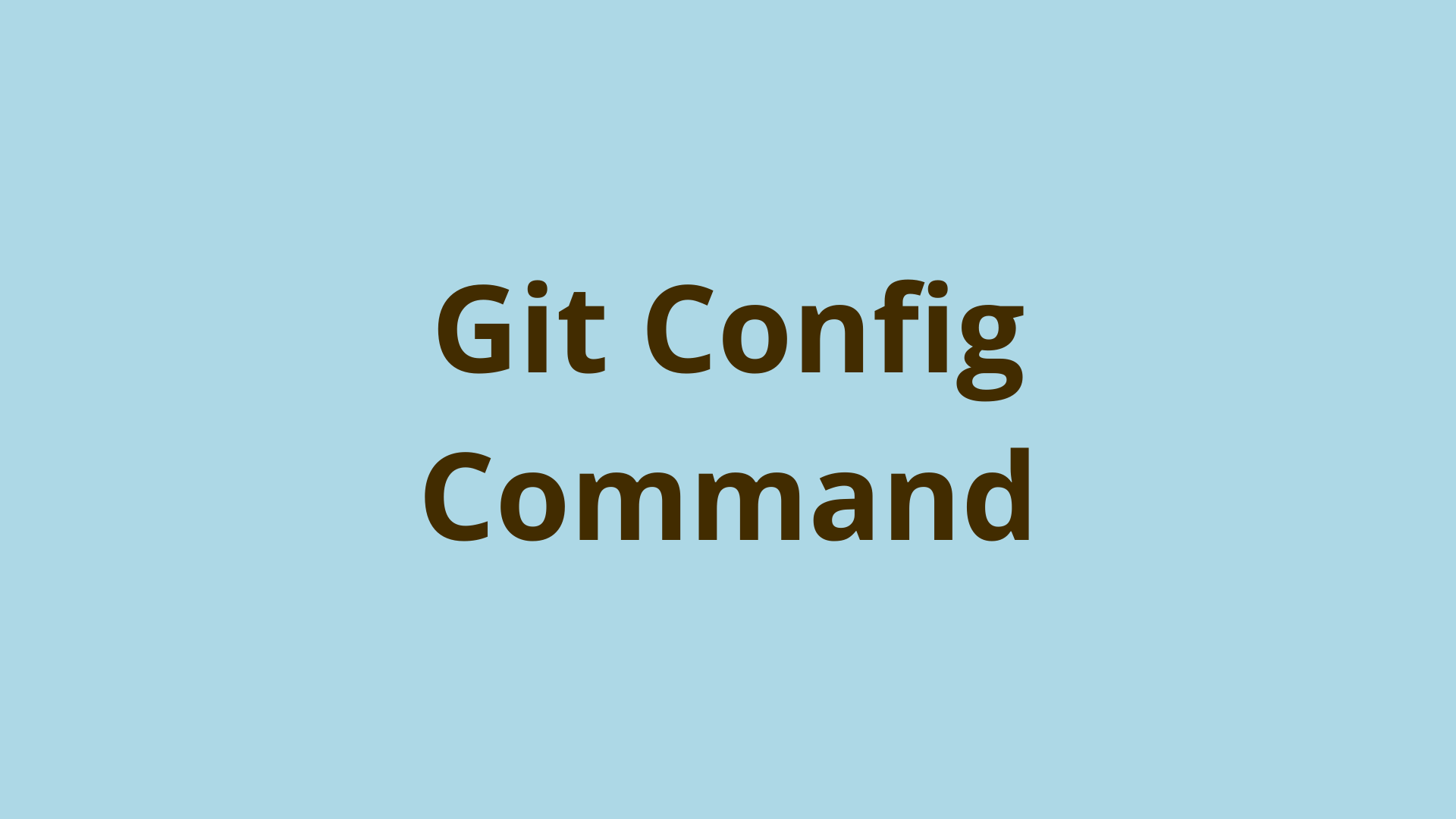
ADVERTISEMENT
Table of Contents
- Introduction
- Installing Git
- Adding Git to the System Path
- What is git config?
- Where is the Git config file stored?
- First Time Git Setup - git config username and email
- Git Text Editor Configuration Settings
- How Do I See My git config?
- How Do You Open the Default Git Config File?
- Change Settings and Values in Git Config
- Create Your First Git Repository and Branch
- Using Git Config with GitHub
- Summary
- Next Steps
- References
Introduction
In order to develop with Git, you'll need to set up your local Git environment, which is typically a 4 part process:
- Install Git on your machine
- Ensure Git can be found on the system path
- Configure some important Git settings using the
git config
command - Initialize a new repo or clone your repo from a remote like GitHub
In this article, we'll touch on these steps, with a focus on the git config command and its various features. You'll see that Git's convenient configuration settings can help automate the Git workflow. As you learn more about Git you'll discover a variety of customization and automation tools, another of which are Git command hooks. But in this post we'll keep it simple and stick to the main Git config features.
Installing Git
Naturally, a prerequisite for configuring Git is getting it installed. On Mac or Linux you can install Git through your operating system's default package manager. Simply copy and paste the command appropriate for your machine:
- macOS:
brew install git
- Ubuntu/Debian:
sudo apt-get install git
- CentOS/Red Hat:
yum install git
On Windows you can download Git from git-scm, where you can also find a lot of helpful information from members of the Git community. Once installed you can open up a terminal window and type in git --version
to see the version installed. It should look something like this:
$ git --version
git version 2.30.1 (Apple Git-130)
Adding Git to the System Path
Git is usually automatically configured with environment variable defaults during installation.
If you get an error running the Git --version command, it's possible that Git was not added to the system path during installation. Adding Git to the system path ensures that your OS knows where to find the Git executables when you run Git commands from the terminal.
Depending on you operating system, you may need to add Git to the path in Windows, add Git to the path on macOS and Linux.
What is git config?
The git config
command is one of the most important beginner Git commands. It is used to set or change various Git configurations and settinsg that
Running the bare bones command git config
with no parameters or flags will display the git config usage and common command line options.
Git's configurations can either be applied globally for your logged in user, or locally to the specific Git repo you're working in. By default, configurations are applied locally within your current Git repo that you navigated into.
We will be covering the main flags that you should know so that you can effectively configure Git to work well for you.
Where is the Git config file stored?
As mentioned above, there are a few ways to specify configurations for Git, the two main ones being locally (the default) and globally.
Within a specific Git repository, the configurations are stored inside the hidden .git/
folder in a Git config file called config
. So the path to this file is your_project/.git/config
.
The global Git configuration file for the currently logged-in OS user can be found in that user's home folder ~/.gitconfig
:
- macOS/Linux: ~/.gitconfig
- Windows: C:\Users\username.gitconfig
In Git, configuration settings are scoped similarly to most programming languages. This means that settings that are defined locally within a specific Git repository will take precedence over fields that are defined globally at the user level.
When running git config, you can use the "--global" flag to apply settings globally, or the "--local flag" to apply settings locally. You can also use "-f filename" to specify a specific configuration file to use.
First Time Git Setup - git config username and email
Before you set off making a bunch of repositories there are a few configuration items you will probably want to set first. The main two would be to set your Git username and Git email address- i.e. to git config username and email. You will most likely want these fields to remain the same across repositories so it makes sense to set them globally.
Username Setting Configuration
Git includes a diverse set of intuitive commands for developers. The git config setting "user.name" allows you to set the username for all of the Git repositories you work with on your machine:
$ git config --global user.name <user name>
This git config username will be the commit author that shows up in your Git commits, so that other developers know who made the each commit.
Email Setting Configuration
Similarly the git config setting "user.email" allows you to set the email associated with each commit you make:
$ git config –global user.email <email address>
Git Text Editor Configuration Settings
Configuration is not limited just to Git username and email. Git provides a number of other settings you can configure. One of the most important is Git's default text editor. This editor will be used whenever Git opens a text editor in your workflow, such as when writing commit messages without the -m
flag.
To specify Git's editory, use the core.editor setting:
git config --global core.editor <editor>
If you choose not to set a preferred editor git will default to the OS default text editor, which is often the Vim text editor on a Unix/Linux system. Other popular choices are emacs, vs code, sublime, atom, and others.
Git allows customization of many other core options including color scheme, diff colors, aliases, and more.
How Do I See My git config?
After you have defined some Git settings using git config, you may be curious how to view Git config. In most cases, this is pretty easy to check. If you receive an error message upon submitting the git config command, then the setting was not applied. Otherwise it likely was saved.
However, there is a better way to check. Executing the git config --list
command will show a neat list of all the defined Git settings. This is a convenient way to double check that you did not make any typos or accidentally set your personal email when you should have set your business one. Assuming the only commands you ran are the git config global ones above and you are not browsed within a Git repository, the output of git config --list will look something like this:
$ git config --list
user.name=YOUR NAME HERE
user.email=YOUREMAIL@XYZ.COM
One thing to be aware of is that default variables will not be displayed. If for example you did not set a preferred text editor using the command showed above you will not see when you run the list command.
When running git config --list
inside a repository, the output will combine both the global and local settings into a single list so that you can see every setting in effect, such as:
$ git config --list
user.name=YOUR NAME HERE
user.email=YOUREMAIL@YOUREMAIL.COM
remote.origin.url=git@github.com:GitHubUserName/RepositoryName.git
remote.origin.fetch=+refs/heads/*:refs/remotes/origin/*
branch.master.remote=origin
branch.master.merge=refs/heads/master
Lastly, if you are just interested in the value of a particular Git config setting you can use the --get FIELD
to retrieve the value of a specific field. It is a nice and clutter free way to check one item. To check for your user name only you would run git config --global --get user.name
which would simply print your user name to the console:
$ git config --global --get user.name
YOUR USERNAME
How Do You Open the Default Git Config File?
Another way is to open up your config file in the editor. To do this you can use the -e
flag. You can use this command in conjunction with the --global
flag to open up the global config file. The command looks like this git config --global -e
and the output will look like so:
$ git config --global -e
[user]
name = YOUR NAME HERE
email = YOUREMAIL@YOUREMAIL.COM
You can also use the -e
flag with the --local
flag. Doing so will open up the Git local config file.
Note that using the --local flag outside of a Git repository will throw the error: "fatal: --local can only be used inside a git repository"
Do not be surprised if your local file has some additional configuration that you did not explicitly specify yourself. If for example you you are working with a tool like GitHub you will like see sections related to Git remotes and Git branches, like:
$ git config --local -e
...
[remote “origin”]
url = git@github.com:GitHubUserName/RepositoryName.git
fetch = +refs/heads/*:refs/remotes/origin/*
[branch "master"]
remote = origin
merge = refs/heads/master
...
Some of this Git configuration gets auto-generated for you by Git, and is perfectly normal. It often gets created when you when you create new branches or sync your repository up with a remote repository, hosted on GitHub or another Git hosting service like BitBucket.
If you want to manually open a local Git configuration file in a text editor, you can run a command like:
$ cd your_project/
$ vim .git/config
If you want to manually open a global Git configuration file in a text editor, you can run a command like:
$ cd your_project/
$ vim ~/.gitconfig
Change Settings and Values in Git Config
In the event you made an error or two when setting a Git config field for the first time do not worry. Reassigning the field is simply as easy as rerunning the command and providing the new value. If for example you made a typo in your name the first time, simply run git config --global user.name "New Name"
to update your Git username.
You should try to memorize a few simple commands for configure some basic fields like your Git username, Git email address, and Git text editor. While you probably will not need to edit your username outside of fixing a typo, things like your email address and preferred editors might change with time.
If you want to delete a Git config that you added previously, you can use the --unset
flag and provide the field you want to delete, such as git config --unset user.name
. The specified Git config will be removed. If you want to delete multiple Git configs at once, you can use --unset-all
to remove all of them using one command.
If you want to remove an entire section (such as the whole user section) you can use git config --global --remove-section user
to delete both the user.name and user.email fields. If you instead want to rename the section to say profile you can use git config --global --rename-section user profile
:
profile.name=YOUR NAME HERE
profile.email=YOUREMAIL@YOUREMAIL.COM
Note that you can technically open up the config file yourself and make these edits manually. However, it is generally recommended that you just run Git's commands to set and edit fields and let the config file be updated automatically.
Create Your First Git Repository and Branch
Now that you are done getting Git installed and have set your global Git configs, you can go ahead and create your first repository.
You will need to create a new directory on your computer and within that directory run the git init
command to initialize a new Git repository. The git init command creates Git's hidden .git/
directory which contains the repository for your new code project.
You can then begin creating files and subdirectories that contain your project's code. Once you're ready to save your changes using Git, you can use the git add command to add your files to the Git staging area in preparation for your initial commit. You can add all your files at once by using git add .
To commit the changes in the staging area, you will want to run the git commit -m "Commit message"
command. This commit message will be attached to the commit, along with your the username and email you configured earlier with git config. If you are familiar with GitHub’s user interface you will see this commit message next to all of the files added that get pushed up with this commit.
Once you make your first commit, your first branch (called master
or main
by default) is officially created!
Using Git Config with GitHub
In order to link your local Git repository with a service like GitHub, you will need to create a repository on GitHub. GitHub will provide you with some commands you can copy, paste, and run locally. In this scenario you are looking for the one that looks like git remote add origin <repository URL>
. You can then run a git push origin main
to push up to the main branch.
You can alternatively work the other way around and create the repository on GitHub first. When creating a repository on GitHub you will have some options to start off with a few generated files like a README. You will then be given a git clone command that looks like git clone <repository URL>
. You will want to paste that command into the whatever directory you would like to work in. From here you can proceed to work on your project and follow the same steps to add, commit, and push code.
There are a few things you should be aware of. If you follow the second workflow you do not need to run the git add remote command. Secondly, while this is not required, many developers like to have a workspace directory containing all of their Git projects. Each project should be its own Git repository.
Summary
In this article, we discussed setting up a new environment using the Git version control system, or VCS. We started by explaining how to install Git on your operating system and checking that the Git commands are available on your system path.
Then we focused on the Git config command. We saw how it can be used locally and globally to apply useful Git config settings, such as your Git user name, Git email, and Git text editor.
We learned where Git's various config files are stored, how to list, update, and delete specific settings.
Next Steps
If you're interested in learning more about how Git works under the hood, check out our Baby Git Guidebook for Developers, which dives into Git's code in an accessible way. We wrote it for curious developers to learn how Git works at the code level. To do this we documented the first version of Git's code and discuss it in detail.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
References
- Git SCM, Getting Started Installing Git - https://git-scm.com/book/en/v2/Getting-Started-Installing-Git
- Git SCM, Download Git - https://git-scm.com/downloads
- Stackoverflow, Adding Git to Path in Windows - https://stackoverflow.com/questions/26620312/git-installing-git-in-path-with-github-client-for-windows
- Stackoverflow, Adding Git to Path in macOS and Linux - https://stackoverflow.com/questions/5545715/how-do-i-add-usr-local-git-bin-to-the-path-on-mac-osx
Final Notes
Recommended product: Decoding Git Guidebook for Developers