Git Cheat Sheet: 12 Essential Git Commands for Intermediate Users
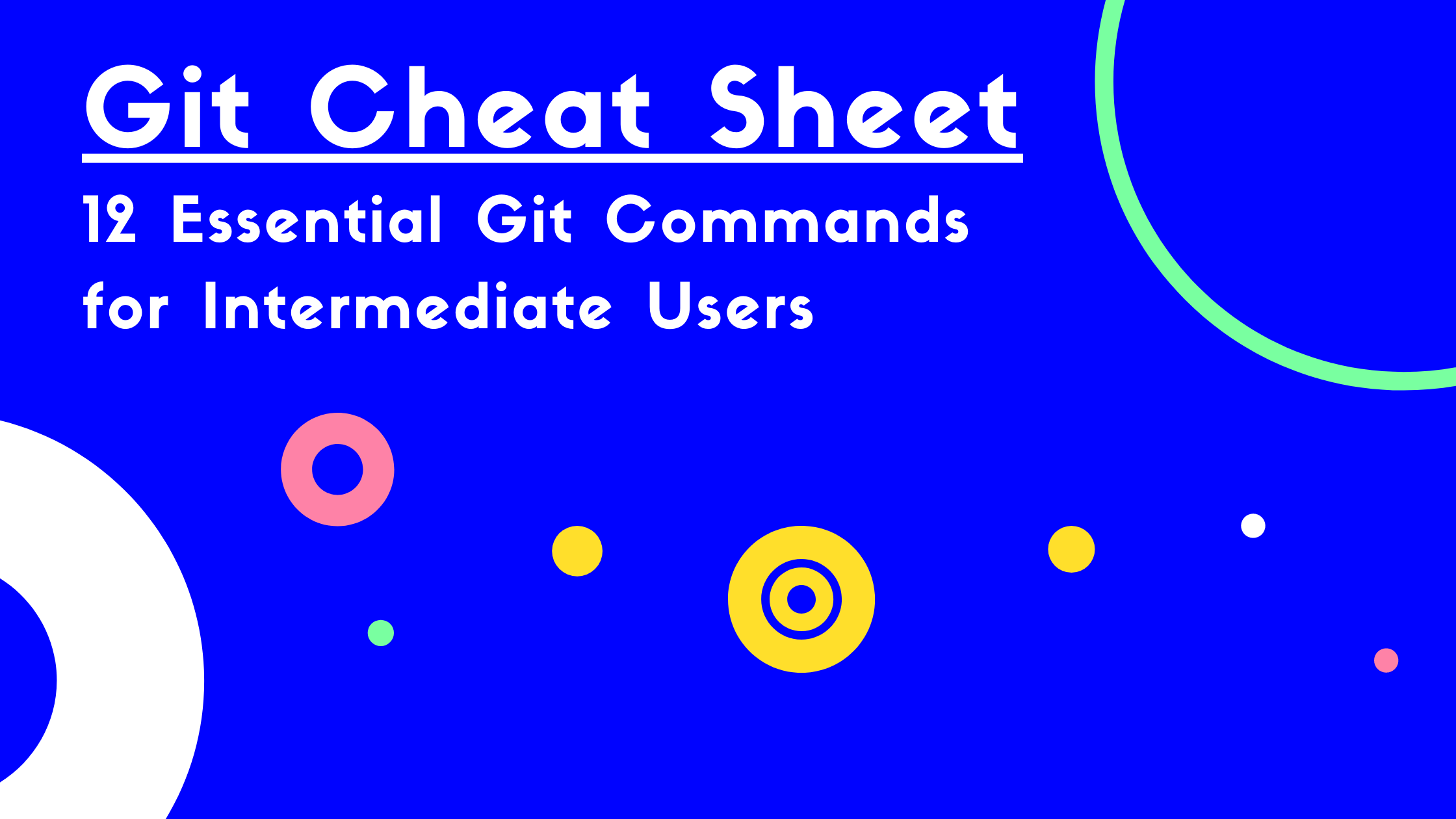
ADVERTISEMENT
Table of Contents
- Introduction
- 1) git config
- 2) git mv
- 3) git rm
- 4) git diff
- 5) git reset
- 6) git tag
- 7) git rebase
- 8) git cherry-pick
- 9) git bisect
- 10) git revert
- 11) git fetch
- 12) git blame
- Conclusion
Introduction
In a previous post, we discussed some essential Git commands for beginners. However, those commands barely scratch the surface of the capabilities that Git provides.
In this article, we’ll discuss 12 essential Git commands for intermediate users.
1) git config
The git config command is used to set Git configuration values. With this command, users can set configurations at three different levels:
- The local level: Within a specific Git repository (stored in the
.git/config
file in your project root). - The global level: For the current user in the operating system.
- The system level: Across all users for the operating system on your machine.
By default, the git config
command changes settings at the local level. This command can be used to set information like your Git user name, email address, default text editor (such as Vim), default merge behavior, terminal output appearance, and aliases.
For example, the current user’s name and email can be set with:
git config --global user.name "Your Name"
git config --global user.email "name@example.com"
The default text editor that Git uses for certain operations can be set to Vim with:
git config --global core.editor "vim"
To list all current values for configuration settings:
git config --list --show-origin
2) git mv
The git mv command is used to move and/or rename a file in a Git repository. The command moves the file in the working directory and updates the Git index for the old and new file paths.
git mv path/to/old_file_name.ext path/to/new_file_name.ext
Note that the file will also be renamed/moved on your filesystem, so this command kills two birds with one stone. However, the history of the old file is all tracked in Git so it can be retrieved at any time if you realize you need the old version.
3) git rm
The git rm command is used to remove a file from a Git repository. It deletes the file in the working directory and removes the file from the Git index.
To remove a specific file:
git rm path/to/filename.ext
Note that this command will delete the file from your filesystem. However, the history of the deleted file is tracked in Git so it can be retrieved at any time if you realize you need it later.
4) git diff
The git diff command shows changes between commits, branches, and other code states. By default, git diff
displays changes made in the working tree relative to the staging index.
This command can also be used to compare the difference between branches:
git diff branch1 branch2
or to compare the difference between two commits:
git diff commitID1 commitID2
To compare a specific file, developers can also add a third filename argument:
git diff commitID1 commitID2 file_to_compare.ext
5) git reset
The git reset command is used to restore a branch to a previous state. When given a commit, branch, or other ref, the command moves the branch and HEAD refs to point to that ref. If no ref argument is provided, the refs are pointed to the HEAD by default.
This command provides three operating modes: soft, mixed, and hard. With the soft command, the refs are updated, but the staged commits and working directory are left unchanged. With the mixed command, (the default option), the index is reset and the staged changes are moved back to the working directory. With the hard option, the index and the working directory are both reset and all changes are lost.
To reset the staging area to match the most recent commit without losing any data, use the command:
git reset
To erase all current changes and reset the working directory to match the most recent commit:
git reset --hard
6) git tag
The git tag command is used to create a human-readable tag (such as a version number) that references a particular commit in the repository. A tag is technically a ref, similarly to a branch name, but tags are usually static in that they point to a single commit, whereas branch names are dynamic in that they track the tip of a branch as new commits are added. In general, tags are useful to capture marked releases.
To create a tag for the currently checked out Git commit:
git tag tag_name
7) git rebase
The git rebase command allows users to move a series of commits to a new base commit. If the user provides a ref argument, the branch is rebased on that branch. Otherwise, the branch is rebased on the remote branch by default:
git rebase origin
The rebase command also provides an --onto
option that performs a rebase starting from a specified commit. This option can be useful for feature development workflows. For example, consider a repo with three branches: master
, feature1
, and feature2
, where feature1
is based on master
and feature2
is based on feature1
. If feature1
is merged into master
before feature2
is ready, feature2
might need to be rebased onto master
. This rebase can be performed using the command:
git rebase --onto master feature1 feature2
By adding an -i flag to the rebase command, users can perform an interactive rebase. In an interactive rebase, users can also combine, split, reorder, remove, and edit commits.
8) git cherry-pick
The git cherry-pick command takes one or more commits and applies them to an existing branch. This command provides a quick way to add commits to multiple branches without performing a rebase.
To cherry-pick an individual commit to the current branch:
git cherry-pick commitID
Users can also cherry-pick a range of commits. By default, cherry-pick does not include the first commit but does include the last:
git cherry-pick oldest_commit...newest_commit
To make the cherry-pick inclusive of the first and last commit, this command can be updated as follows:
git cherry-pick oldest_commit^...newest_commit
9) git bisect
The git bisect command is used to isolate a specific commit by performing a binary search through the commit history. In particular, this command is useful for identifying which commit introduced a bug.
To run this command, identify a past commit where the problem does not occur, denoted here as "good_commit_id." While on the recent branch, run:
git bisect start
git bisect bad
git bisect good good_commit_id
Git will perform a binary search through the commit history. It will do this by checking out a commit midway between the current commit and the last good commit. At this point, it's up to you to check or test your code to see if the bug still exists. If the bug is still preset, run the command git bisect bad
and git bisect good
if it does not.
Once found, the search will return the commit ID of the first bad commit. From here, the user can examine the commit that introduced the bug. The command git bisect reset
can then be used to restore the branch to its previous state before performing the search.
This provides a quick way for developers to track down the source of a bug, since splitting the commit history in half each time exponentially reduces the number of commits that need to be manually checked.
10) git revert
The git revert
command is used to undo changes from a previous commit. The command takes a commit ID and creates a new commit on the current branch that inverses the changes in that commit. This approach allows users to undo a change without rewriting history. It will result in a new commit at the tip of your branch with undoes the changes in the reverted commit.
git revert commitID
11) git fetch
The git fetch command download refs (both tags and branches) from a remote repository. It downloads newly created refs and completes the history of refs already stored locally. Unlike git pull
, the git fetch
command does not update your local repository’s working state and it does not perform a merge. By default, the git fetch
command fetches all refs for the current repository.
To fetch all refs from the remote repository, use the command:
git fetch origin
To instead fetch the refs from a specific branch:
git fetch origin branch_name
12) git blame
With the git blame command, users can view when each line in a file was last modified and who was responsible for that modification. This tool is useful for understanding the history of a file, and it can help users determine who to refer to for questions or advice about code changes.
To view this information for a specific file:
git blame path/to/filename.ext
Conclusion
Although not required to use Git at the most basic level, these intermediate commands can help users can improve their productivity with Git. They provide a robust set of functionality that will help make you a well rounded developer, which generally means solving problems in creative ways.
If you want to learn about Git in more depth, check out our top Git books.
If you're interested in learning more about how Git works under the hood, check out our Baby Git Guidebook for Developers, which dives into Git's code in an accessible way. We wrote it for curious developers to learn how Git works at the code level. To do this we documented the first version of Git's code and discuss it in detail.
We hope you enjoyed this post! Feel free to shoot me an email at jacob@initialcommit.io with any questions or comments.
Final Notes
Recommended product: Decoding Git Guidebook for Developers