How to change the default port of Spring Boot application
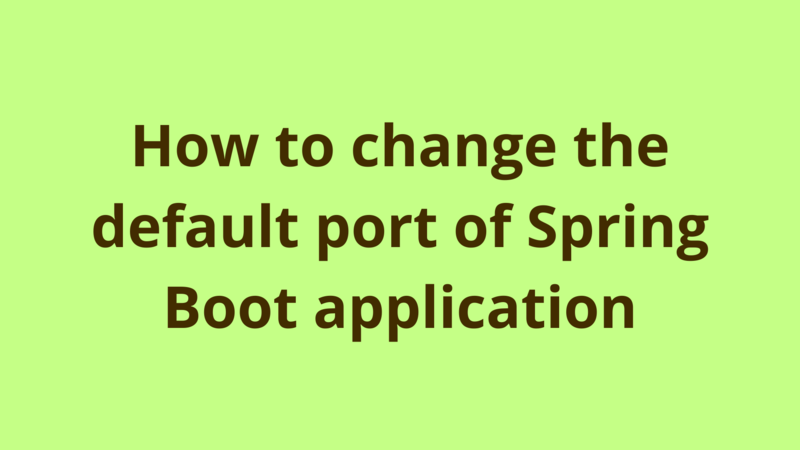
ADVERTISEMENT
Table of Contents
Introduction
By default, Spring Boot applications run on an embedded Tomcat via port 8080. In order to change the default port, you just need to modify server.port attribute which is automatically read at runtime by Spring Boot applications.
In this tutorial, we provide the common ways of modifying server.port attribute.
1- application.properties
Create application.properties file under src/main/resources and define server.port attribute inside it:
server.port=9090
2- EmbeddedServletContainerCustomizer
You can customize the properties of the default servlet container through implementing the EmbeddedServletContainerCustomizer interface as the following:
package com.programmer.gate;
import org.springframework.boot.context.embedded.ConfigurableEmbeddedServletContainer;
import org.springframework.boot.context.embedded.EmbeddedServletContainerCustomizer;
public class CustomContainer implements EmbeddedServletContainerCustomizer {
@Override
public void customize(ConfigurableEmbeddedServletContainer container) {
container.setPort(9090);
}
}
The port defined inside the CustomContainer always overrides the value defined inside application.properties.
3- Command line
The third way is to set the port explicitly when starting up the application through the command line, you can do this in 2 different ways:
- java -Dserver.port=9090 -jar executable.jar
- java -jar executable.jar –server.port=9090
The port defined using this way overrides any other ports defined through other ways.
Summary
By default, Spring Boot applications run on an embedded Tomcat via port 8080. In order to change the default port, you just need to modify server.port attribute which is automatically read at runtime by Spring Boot applications.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers