How to convert multi-page TIFF to PDF in Java
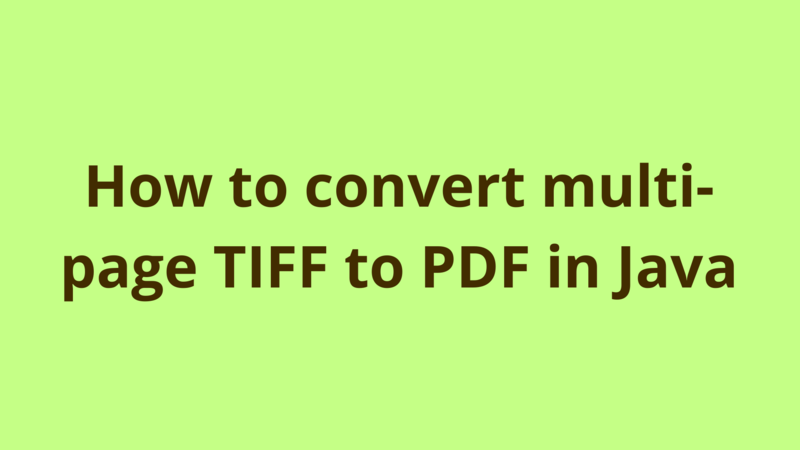
ADVERTISEMENT
Table of Contents
Introduction
This tutorial provides a very efficient way to convert a multi-page TIFF to PDF using iText library.
The below utility method accepts a multi-page TIFF file as an input and returns a PDF file as an output. In order to use it, you must add the iText library to your classpath.
public static File convertTIFFToPDF(File tiffFile)
{
File pdfFile = new File("C:\\Users\\user\\\\Desktop\\output.pdf");
try
{
RandomAccessFileOrArray myTiffFile = new RandomAccessFileOrArray(tiffFile.getCanonicalPath());
// Find number of images in Tiff file
int numberOfPages = TiffImage.getNumberOfPages(myTiffFile);
Document TifftoPDF = new Document();
PdfWriter pdfWriter = PdfWriter.getInstance(TifftoPDF, new FileOutputStream(pdfFile));
pdfWriter.setStrictImageSequence(true);
TifftoPDF.open();
Image tempImage;
// Run a for loop to extract images from Tiff file
// into a Image object and add to PDF recursively
for (int i = 1; i <= numberOfPages; i++) {
tempImage = TiffImage.getTiffImage(myTiffFile, i);
Rectangle pageSize = new Rectangle(tempImage.getWidth(), tempImage.getHeight());
TifftoPDF.setPageSize(pageSize);
TifftoPDF.newPage();
TifftoPDF.add(tempImage);
}
TifftoPDF.close();
}
catch(Exception ex)
{
ex.printStackTrace();
}
return pdfFile;
}
Happy Coding!
Summary
This tutorial provides a very efficient way to convert a multi-page TIFF to PDF using iText library.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers