How to create a zip file in Java
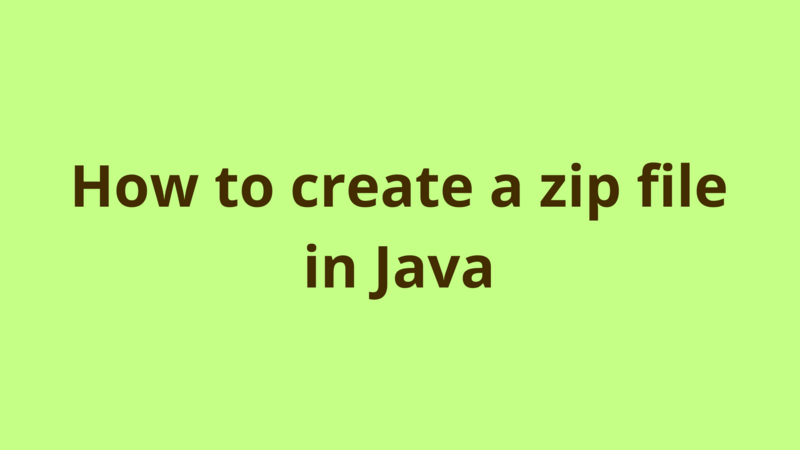
ADVERTISEMENT
Table of Contents
Introduction
In this tutorial, we show how to create a zip file from multiple files in Java.
1- ByteArrayOutputStream & ZipOutputStream
Using ByteArrayOutputStream and ZipOutputStream classes provided by the JDK, you can generate a zip file out of multiple files.
The following utility method accepts a list of File objects and generates a zip file as a byte array:
public byte[] zipFiles(List<File> files){
byte[] result = null;
try (ByteArrayOutputStream fos = new ByteArrayOutputStream(); ZipOutputStream zipOut = new ZipOutputStream(fos);) {
for (File fileToZip : files) {
try (FileInputStream fis = new FileInputStream(fileToZip);) {
ZipEntry zipEntry = new ZipEntry(fileToZip.getName());
zipOut.putNextEntry(zipEntry);
IOUtils.copy(fis, zipOut);
}
}
zipOut.close();
fos.close();
result = fos.toByteArray();
}
catch (Exception ex)
{
ex.printStackTrace();
}
return result;
}
That’s it.
Summary
In this tutorial, we show how to create a zip file from multiple files in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers