How to iterate a Map in Java
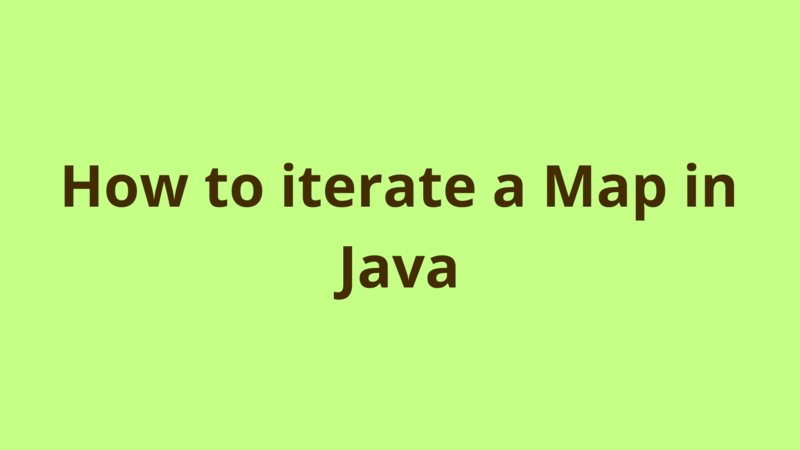
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows several ways for iterating a Map in Java.
1- Entry Set
The common way for iterating a Map in Java is through entrySet() method as the following:
private static void iterateMapUsingEntrySet(Map<String, Double> studentGrades)
{
for(Entry<String, Double> entry : studentGrades.entrySet())
{
System.out.println("Key = " + entry.getKey());
System.out.println("Value = " + entry.getValue());
}
}
2- Iterator
You can also use entrySet() along with iterator() methods to iterate a Map through an Iterator:
private static void iterateMapUsingIterator(Map<String, Double> studentGrades)
{
Iterator entries = studentGrades.entrySet().iterator();
for(Entry entry = (Entry) entries.next(); entries.hasNext();)
{
System.out.println("Key = " + entry.getKey());
System.out.println("Value = " + entry.getValue());
}
}
3- keySet()
Using keySet() method, you can retrieve all the keys of the map and then get their corresponding value:
private static void iterateMapUsingKeySet(Map<String, Double> studentGrades)
{
for(String key : studentGrades.keySet())
{
System.out.println("Key = " + key);
System.out.println("Value = " + studentGrades.get(key));
}
}
4- values()
You can use values() method to retrieve all the values as a Collection without their corresponding keys.
private static void iterateMapUsingValues(Map<String, Double> studentGrades)
{
for(Double value : studentGrades.values())
{
System.out.println("Value = " + value);
}
}
5- Java 8
With Java 8, you can iterate over a Map through one line using forEach() method:
private static void iterateMapUsingJava8(Map<String, Double> studentGrades)
{
studentGrades.forEach((key,value) ->
{
System.out.println("Key = " + key);
System.out.println("Value = " + value);
});
}
Summary
This tutorial shows several ways for iterating a Map in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers