How to modify XML using DOM in Java
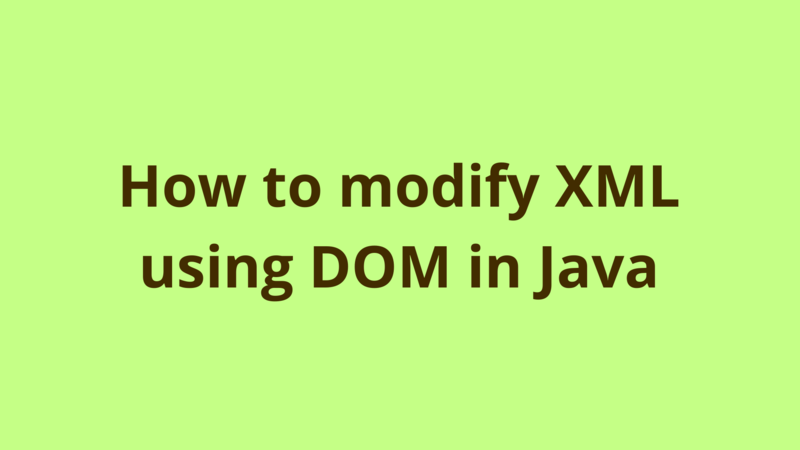
ADVERTISEMENT
Table of Contents
- Introduction
- 1- Students.xml
- 2- Add attribute
- 3- Modify existing element
- 4- Add an element
- 5- Delete existing element
- 6- Demo
- 7- Source Code
- Summary
- Next Steps
Introduction
Although the main purpose of XML documents is to store lightweight data for faster processing, they are still used in some small applications as a data store which requires dynamic modification. In this article we show how to modify an XML document using DOM in Java.
You can still refer to previous articles which cover how to create and parse XML documents using DOM.
1- Students.xml
Consider we have the following XML document:
<students>
<student graduated="yes">
<id>1</id>
<name>Hussein</name>
</student>
<student>
<id>2</id>
<name>Alex</name>
</student>
</students>
Throughout this tutorial, we’re going to do the following modifications on the above XML:
- Add an attribute to an existing element.
- Modify the content of an existing element.
- Add a child element to an existing element.
- Delete an existing element
2- Add attribute
In the following code block we set a specific student as graduated through iterating over the student elements and calling setAttribute() on the required student.
private static void setGraduatedStudent(Document doc, int id) {
NodeList students = doc.getElementsByTagName("student");
for(int i=0; i < students.getLength();i++)
{
Element studentNode = (Element) students.item(i);
int studentId = Integer.valueOf(studentNode.getElementsByTagName("id").item(0).getTextContent());
if(studentId == id)
{
studentNode.setAttribute("graduated", "true");
}
}
}
3- Modify existing element
In the following code block we modify the first name of a specific student. To do so, we iterate over student elements and call setTextContent() on the “name” element of the appropriate student.
private static void modifyStudentFirstName(Document doc, int id, String updatedFirstName) {
NodeList students = doc.getElementsByTagName("student");
for(int i=0; i < students.getLength();i++)
{
Element studentNode = (Element) students.item(i);
int studentId = Integer.valueOf(studentNode.getElementsByTagName("id").item(0).getTextContent());
if(studentId == id)
{
Element studentName = (Element) studentNode.getElementsByTagName("name").item(0);
studentName.setTextContent(updatedFirstName);
}
}
}
4- Add an element
In the following code block we add a new child element called “lastName” to a specific student. To do so, we iterate over student elements and when we find the requested student, we create an element using doc.createElement() and then append it to the student element using appendChild().
private static void setStudentLastName(Document doc, int id, String lastName) {
NodeList students = doc.getElementsByTagName("student");
for(int i=0; i < students.getLength();i++)
{
Element studentNode = (Element) students.item(i);
int studentId = Integer.valueOf(studentNode.getElementsByTagName("id").item(0).getTextContent());
if(studentId == id)
{
Element lastNameElement = doc.createElement("lastName");
lastNameElement.setTextContent(lastName);
studentNode.appendChild(lastNameElement);
}
}
}
5- Delete existing element
In the following code block, we delete the “lastName” element of a specific student. To do so, we iterate over student elements and call removeChild() on the “lastName” element of the requested student.
private static void removeStudentLastName(Document doc, int id) {
NodeList students = doc.getElementsByTagName("student");
for(int i=0; i < students.getLength();i++)
{
Element studentNode = (Element) students.item(i);
int studentId = Integer.valueOf(studentNode.getElementsByTagName("id").item(0).getTextContent());
if(studentId == id)
{
Element studentLastName = (Element) studentNode.getElementsByTagName("lastName").item(0);
studentNode.removeChild(studentLastName);
}
}
}
6- Demo
For demo purposes, we create a main method which reads students.xml document and modifies it using the above methods as the following:
public static void main(String[] args) throws Exception {
File xmlFile = new File("students.xml");
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(xmlFile);
setGraduatedStudent(doc, 2);
modifyStudentFirstName(doc,2,"Alexa");
setStudentLastName(doc,1,"Terek");
removeStudentLastName(doc,1);
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer();
DOMSource source = new DOMSource(doc);
StreamResult result = new StreamResult(new File("students.xml"));
transformer.transform(source, result);
}
After executing the above main method, students.xml would look like the following:
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<students>
<student graduated="true">
<id>1</id>
<name>Hussein</name>
</student>
<student graduated="true">
<id>2</id>
<name>Alexa</name>
</student>
</students>
7- Source Code
You can download the source code from this repository: Read-XML
Summary
Although the main purpose of XML documents is to store lightweight data for faster processing, they are still used in some small applications as a data store which requires dynamic modification. In this article we show how to modify an XML document using DOM in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers