How to read input from console in Java
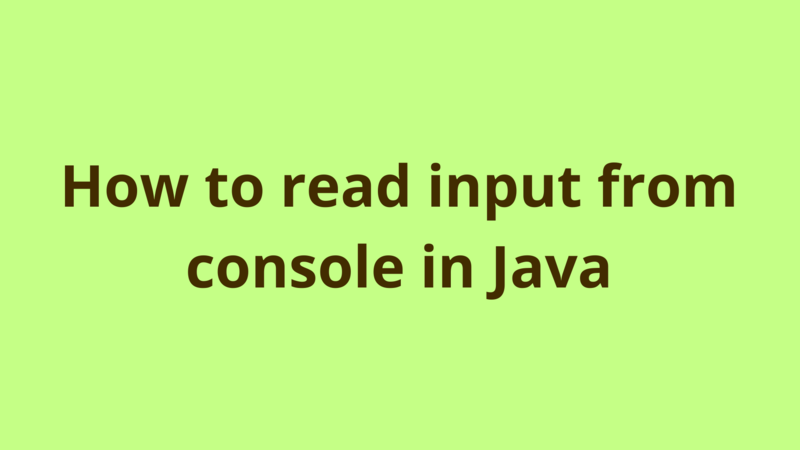
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows several ways for reading input from a console in Java.
Before listing the ways, it’s worth to define System.in as it’s used by most reading ways.
System.in is a static field of type InputStream representing the stream which corresponds to keyboard input or any other input source specified by the host machine.
1- BufferedReader
The oldest way of reading input from console in Java is through BufferedReader which is introduced in JDK 1.0. To do so, we use InputStreamReader and System.in as the following:
private static void readInputUsingBufferedReader() throws IOException
{
System.out.println("Please enter your Full name: ");
try (BufferedReader br = new BufferedReader(new InputStreamReader(System.in)))
{
String fullName = br.readLine();
System.out.println("Your full name is: " + fullName);
}
}
2- Scanner
The most popular way of reading input from console in Java is through Scanner object which is introduced in JDK 1.5. Scanner also uses System.in and provides several utility methods for reading input of specific types.
private static void readInputUsingScanner()
{
System.out.println("Please enter your Full name: ");
Scanner in = new Scanner(System.in);
System.out.println("Your full name is: " + in.nextLine());
}
Some of the common methods provided by Scanner:
- next(): this method reads the next String token as per the defined delimiter. (the default delimiter is space)
- nextLine(): this method reads the remaining input from the current cursor till the end of the line.
- nextInt(): this method reads the next integer provided in the input. If no integer is provided then InputMismatchException is thrown.
- nextDouble(): this method reads the next double provided in the input. If no double is provided then InputMismatchException is thrown.
3- System.console
This is the modern way of reading input from console in Java, it’s introduced in JDK 1.6 and allows developers to read input without using System.in. The drawback of using this technique is that it doesn’t work under IDE and only works in interactive environments.
private static void readInputUsingConsole() throws IOException
{
System.out.println("Please enter your Full name: ");
Console console = System.console();
String fullName = console.readLine();
System.out.println("Your full name is: " + fullName);
}
Summary
This tutorial shows several ways for reading input from a console in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers