How to read XML using STAX parser
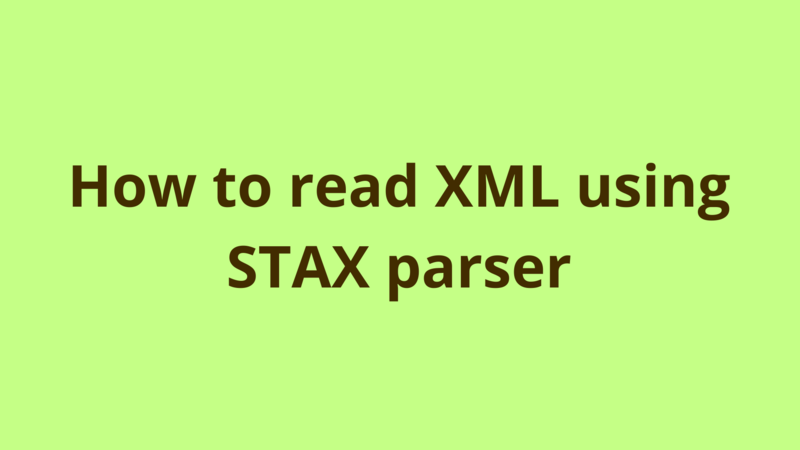
ADVERTISEMENT
Table of Contents
Introduction
STAX is yet another parser for reading and parsing XML documents in Java, it is very similar to SAX where they both parse the XML document on call and provide events to the developer in order to handle each read tag separately, unlike DOM which stores the XML as a document in the memory.
The main difference between SAX and STAX is that the former pushes the events to the developer through callback methods defined inside a handler while the latter allows the developer to pull events on request.
In this tutorial, we provide an example for parsing an XML document called Students.xml using STAX parser.
1- Students.xml
Consider we have the following Students.xml file:
<students>
<student graduated="true">
<id>1</id>
<name>Hussein</name>
</student>
<student>
<id>2</id>
<name>Alex</name>
</student>
</students>
2- Student.java
For mapping purposes, we create Student.java for populating each student element inside Students.xml:
package com.programmer.gate;
public class Student {
private int id;
private String name;
private boolean isGraduated;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public boolean isGraduated() {
return isGraduated;
}
public void setGraduated(boolean isGraduated) {
this.isGraduated = isGraduated;
}
}
3- STAX parser
In this section, we’re going to parse students.xml and populate a List of Student objects out of it.
STAX parses documents through event readers, each event refers to a read element. There are mainly 3 event types:
- START_ELEMENT: This event means that the parser starts parsing a new element in the document.
- END_ELEMENT: This event means that the parser finishes parsing a specific element in the document.
- CHARACTERS: This event means that the parser reads the text value of the currently parsed element.
Putting all together, we implement the following class for parsing students.xml and populating List of Student objects:
package com.programmer.gate;
import java.io.FileReader;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.stream.XMLEventReader;
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLStreamConstants;
import javax.xml.stream.events.Attribute;
import javax.xml.stream.events.Characters;
import javax.xml.stream.events.EndElement;
import javax.xml.stream.events.StartElement;
import javax.xml.stream.events.XMLEvent;
import org.xml.sax.SAXException;
public class ReadXMLWithSTAX {
public static void main(String[] args) throws ParserConfigurationException, SAXException {
try
{
XMLInputFactory factory = XMLInputFactory.newInstance();
XMLEventReader eventReader = factory.createXMLEventReader(new FileReader("students.xml"));
List<Student> students = new ArrayList<Student>();
Student student = null;
String elementValue = null;
while(eventReader.hasNext()) {
XMLEvent event = eventReader.nextEvent();
switch(event.getEventType()) {
case XMLStreamConstants.START_ELEMENT:
{
StartElement startElement = event.asStartElement();
String qName = startElement.getName().getLocalPart();
if (qName.equalsIgnoreCase("student")) {
student = new Student();
Iterator<Attribute> attributes = startElement.getAttributes();
if(attributes.hasNext())
{
Attribute attr = attributes.next();
String graduated = attr.getValue();
student.setGraduated(Boolean.valueOf(graduated));
}
}
break;
}
case XMLStreamConstants.CHARACTERS:
{
Characters characters = event.asCharacters();
elementValue = characters.getData();
break;
}
case XMLStreamConstants.END_ELEMENT:
{
EndElement endElement = event.asEndElement();
String qName = endElement.getName().getLocalPart();
if (qName.equalsIgnoreCase("student")) {
students.add(student);
}
if (qName.equalsIgnoreCase("id")) {
student.setId(Integer.valueOf(elementValue));
}
if (qName.equalsIgnoreCase("name")) {
student.setName(elementValue);
}
break;
}
}
}
for(Student s : students)
{
System.out.println("Student Id = " + s.getId());
System.out.println("Student Name = " + s.getName());
System.out.println("Is student graduated? " + s.isGraduated());
}
}
catch(Exception ex)
{
ex.printStackTrace();
}
}
}
When executing the above class, we get the following output:
Student Id = 1
Student Name = Hussein
Is student graduated? true
Student Id = 2
Student Name = Alex
Is student graduated? false
4- Source Code
You can download the source code from this repository: Read-XML
Summary
In this tutorial, we provide an example for parsing an XML document called Students.xml using STAX parser.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers