How to return a custom class from Hibernate query
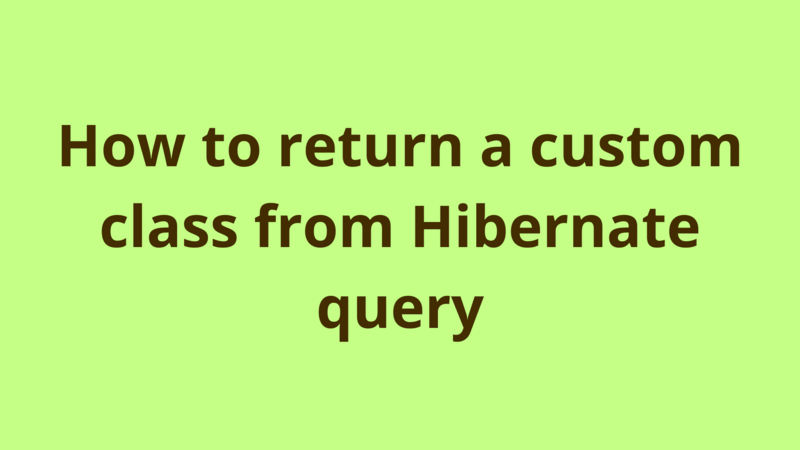
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Several Entities with common attributes
- 2. Custom class
- 3. Using a Constructor in HQL
- Summary
- Next Steps
Introduction
Hibernate is one of the most popular ORM frameworks in Java. Through Hibernate, developers deal with Java POJOs for doing CRUD operations on the database.
In order to return a Java POJO from a Hibernate query, you can simply run this HQL“FROM <ENTITY_CLASS>” which in turn returns an object of type <ENTITY_CLASS>.
This looks awesome and saves the developer from mapping the entities manually. However, in some scenarios, this would become a bit cumbersome and repetitive especially when you have many entities with the same attributes or even when you need to retrieve only some specific attributes from a very large entity.
1. Several Entities with common attributes
Suppose you have 3 lookup tables “PRIVACY”, “PRIORITY” and “DOCUMENT_TYPE” each holding 2 columns “ID” and “DESCRIPTION”.
Using “FROM < ENTITY_CLASS >” syntax, the developer must create a service method for every single entity in order to retrieve its data.
2. Custom class
In order to make it generic, we create a custom POJO named as GenericLookup which holds “id” and “description” fields as the following:
package com.analytics.db.model;
import java.io.Serializable;
public class GenericLookup implements Serializable{
private static final long serialVersionUID = 1L;
private String id;
private String description;
public GenericLookup(String id, String description) {
this.id = id;
this.description = description;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
It’s worth to mention that the copy constructor is very important in this example as will be shown later in this tutorial.
3. Using a Constructor in HQL
After we create the custom class, we can simply create one service method for the 3 entities as the following:
public List<GenericLookup> findQuery(String entity) {
List<GenericLookup> data = getCurrentSession().createQuery("select new com.programmer.gate.model.GenericLookup(id,description) from com.programmer.gate.model." + entity).list();
return data;
}
As noticed we use the copy constructor of the GenericLookup class in the HQL and we pass the entity name dynamically through the method input. This way we implement a generic service method that populates a List< GenericLookup> by the entity name.
Summary
Hibernate is one of the most popular ORM frameworks in Java. Through Hibernate, developers deal with Java POJOs for doing CRUD operations on the database.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers