How to send data from Javascript to Servlet
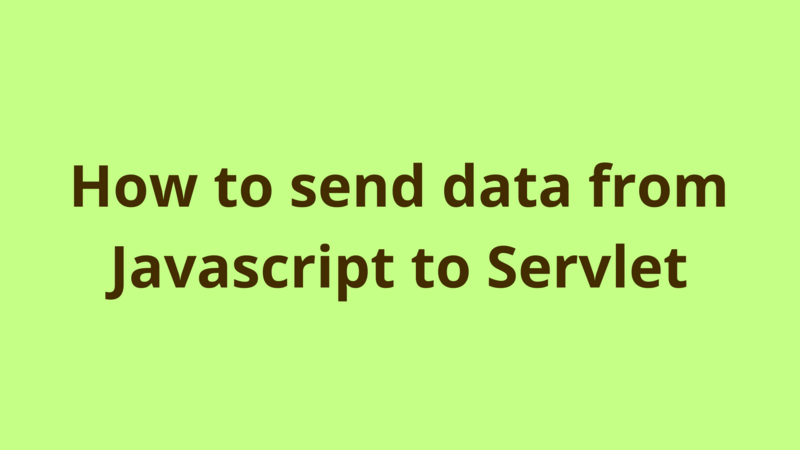
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Submit form programmatically
- 2. Pass data from Javascript to Servlet through AJAX
- Summary
- Next Steps
Introduction
In modern web applications, the data exchange between front-end and back-end is usually done dynamically through javascript.
In this tutorial, we explain 2 ways of exchanging data between javascript and java servlets, we also provide an example and business cases for the usage of each way.
1. Submit form programmatically
The first case is when you already have an HTML form on your page and you don’t want to pass the form data as is to the back-end, e.g. a typical scenario is that your servlet expects a dynamic field other than the form fields filled by the end-user.
To resolve this situation, the expected dynamic field is normally defined as a hidden form field that is set through javascript, alternatively, the form is submitted programmatically.
Consider the following HTML form:
<form action="/VehicleProcessor">
<input type="checkbox" name="vehicle" value="Bike"> I have a bike<br>
<input type="checkbox" name="vehicle" value="Car"> I have a car<br>
<input type="checkbox" name="vehicle" value="Motorcycle"> I have a motorcycle<br>
<input type="submit" value="Submit">
</form>
This is a typical form that asks the user for his preferred vehicle types and submits the request to the VehicleProcessor servlet.
In our example, we consider that VehicleProcessor is implemented in a generic way that doesn’t explicitly process each vehicle type, however, it expects a parameter called ‘selectedVehicles’ which represents a comma separated string of all the selected vehicles.
So we go back to our form and add a hidden form field called ‘selectedVehicles’ as the following:
<input type="hidden" name="selectedVehicles" id="selectedVehicles"/>
This field needs to be set dynamically through javascript before submitting the request to the backend, so we assign an id to our form in order to retrieve it programmatically and we add an onClick event on the submit button so that we process the form at the front-end before submitting it to the servlet.
Our form would finally look like the following:
<form id="vehicles" action="VehicleProcessor">
<input type="checkbox" name="vehicle" value="Bike"> I have a bike<br>
<input type="checkbox" name="vehicle" value="Car"> I have a car<br>
<input type="checkbox" name="vehicle" value="Motorcycle"> I have a motorcycle<br>
<input type="hidden" name="selectedVehicles" id="selectedVehicles"/>
<input type="submit" value="Submit" onClick="processVehicles()">
</form>
At the javascript side, we define a method called ‘processVehicles()’ which does the following:
- set the value of ‘selectedVehicles’ as a comma-separated String of all selected vehicles.
- submit the form.
<script>
function processVehicles()
{
var vehicleTypes = document.getElementsByName("vehicle");
var selectedVehicleTypes = [];
for (var i = 0; i < vehicleTypes.length; i++) {
if (vehicleTypes[i].checked) {
selectedVehicleTypes.push(vehicleTypes[i].value);
}
}
// Set the value of selectedVehicles to comma separated
// String of the selected vehicle types
var hiddenSelectedVehicles = document.getElementById("selectedVehicles");
hiddenSelectedVehicles.value = selectedVehicleTypes.join(",");
// jQuery
/* $("#selectedVehicles").value(selectedVehicleTypes.join(",")); */
// Submit the form using javascript
var form = document.getElementById("vehicles");
form.submit();
//jQuery
/* $("#vehicles").submit(); */
}
</script>
At the server-side, we retrieve the selectedVechicles as the following:
String vehicles = request.getParameter("selectedVehicles");
2. Pass data from Javascript to Servlet through AJAX
Another case is when you explicitly call a servlet method through javascript, the scenarios for this case are very common in every real application: e.g. filling a data table/grid with dynamic data retrieved from the database, handling click events .. and many more scenarios.
Calling a servlet method explicitly inside a javascript function is usually done through AJAX.
Following are the different ways to make AJAX requests through jQuery:
- $.get(URL,data,function(data,status,xhr),dataType): this method loads data from the server through HTTP GET request.
- attributes:
- URL: mandatory, defines the relative path of the servlet.
- data: optional, the data to be passed along with the request (although GET filters are recommended to be sent as a query string)
- function(data, status,xhr): the callback function which is called when the request succeeds.
- dataType: optional, the expected response type from the server.
- example:
- attributes:
$.get("GetVehicleTypes", function(data){
alert("Data: " + data);
});
- $.post(URL,data,function(data,status,xhr),dataType): this method submits a POST request to the server.
- attributes:
- URL: mandatory, defines the relative path of the servlet.
- data: optional, the data to be passed along with the request.
- function(data, status,xhr): the callback function which is called when the request succeeds.
- dataType: optional, the expected response type from the server.
- example:
- attributes:
$.post("OrderService",
{
itemId : '1',
itemName: 'item1',
cost : '100'
}, function(response, status) {
// notify user that his order is successfull
}
)
- $.ajax({name:value, name:value, … }): this is the basic method for performing an asynchronous ajax request, $.get() and $.post() methods are only shortcuts specific for GET and POST requests respectively, all ajax methods call $.ajax() implicitly, it can be used for both GET and POST requests. This method is mostly used for complex requests whenever you want to use extra attributes not supported in $.get() and $.post(), the most important attribute is using error() callback function for handling ajax errors.
- attributes:
- URL: mandatory, defines the relative path of the servlet.
- other optional attributes can be found here.
- example:
- attributes:
$.ajax({url: "RetrieveGridData", type: GET, async: false,
success: function(result){
// Fill the grid dynamically using result
},
error: function(data) {
alert('woops!');
}
});
In javascript, ajax requests are done using XMLHttpRequest, following are examples of GET and POST requests:
- GET request:
function ajaxGet(url, callback) {
var xmlDoc = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject("Microsoft.XMLHTTP");
xmlDoc.open('GET', url, true);
xmlDoc.onreadystatechange = function() {
if (xmlDoc.readyState === 4 && xmlDoc.status === 200) {
callback(xmlDoc);
}
}
xmlDoc.send();
}
- POST request:
function ajaxPost(url, data, callback) {
var xmlDoc = window.XMLHttpRequest ? new XMLHttpRequest() : new ActiveXObject("Microsoft.XMLHTTP");
xmlDoc.open('POST', url, true);
xmlDoc.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
xmlDoc.onreadystatechange = function() {
if (xmlDoc.readyState === 4 && xmlDoc.status === 200) {
callback(xmlDoc);
}
}
xmlDoc.send(data);
}
Summary
In modern web applications, the data exchange between front-end and back-end is usually done dynamically through javascript.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers