How to send data from Java Servlet to JSP
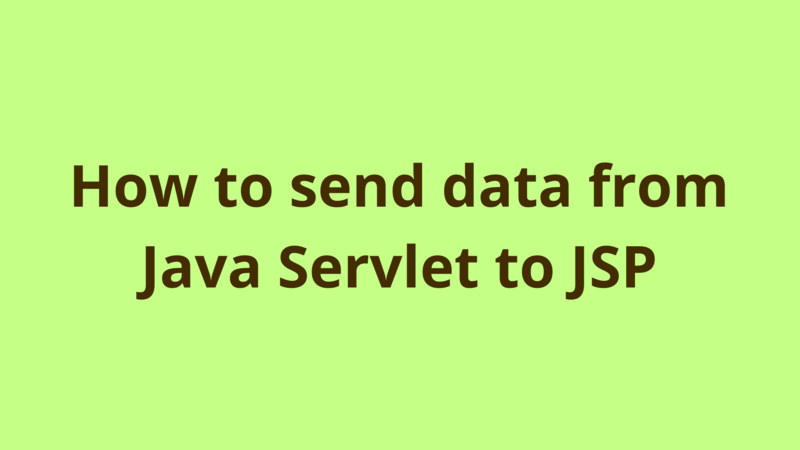
ADVERTISEMENT
Table of Contents
- Introduction
- 1. Using HttpServletRequest
- 2. Redirect to JSP using query string
- 3. Passing Objects from servlet to JSP
- 4. Passing ArrayList from Servlet to JSP
- 5. Passing HashMap from Servlet to JSP
- Summary
- Next Steps
Introduction
JSP is mostly used as the view component in any Java-based MVC application, its main usage is to present dynamic data processed and generated from server-side controllers like a servlet.
In this tutorial, we explain the different ways of sending data from a Java servlet to JSP, along with several examples specific for passing data types as objects, arrays, lists, and maps.
1. Using HttpServletRequest
The common way of passing data from servlet to JSP is through defining attributes in the HTTP request and then forwarding it to the corresponding JSP, this is done on the server-side using one of the following techniques:
- request.setAttribute(Name,Value)
- This technique binds the attribute to the current request, the attribute is only visible to the current request and it keeps alive as far as the request is resolved or keeps being dispatched from servlet to servlet, this technique is very useful in web applications whenever you need to set dynamic attributes specific to the request cycle and need not be available anymore after the request.
- At the server-side, set the attribute in the request then forward the request to the JSP page like the following:
request.setAttribute("name", "Hussein Terek");
request.getRequestDispatcher("home.jsp").forward(request, response);
* In JSP, you can access the attribute like:
<h3> My name is ${name}</h3>
<!-- OR -->
<h3> My name is request.getAttribute("name")</h3>
* Notice the use of ${ATTRIBUTE_NAME} syntax which implicitly checks for the attribute name in the servlet request and replaces it with its value, if attribute not found then an empty string is returned.
- request.getSession().setAttribute(Name,Value)
- This technique binds the attribute to the user’s session, it’s used to provide a state to a set of related HTTP requests, session attributes are only available to those servlets which join the session and they’re automatically killed whenever the session ends. This technique is normally used for passing contextual information like ID, language .. etc
- At the server-side, add the attribute to the request session then forward the request to the JSP page like the following:
request.getSession().setAttribute("name","Hussein Terek");
request.getRequestDispatcher("home.jsp").forward(request, response);
* In JSP, you can access it like:
<h3> My name is request.getSession().getAttribute("name")</h3>
- getServletContext().setAttribute(Name,Value)
- This technique binds the attribute to the whole context of the application, the attribute is available to all servlets (thus JSP) in that context. By definition, a context attribute exists locally in the VM where it is defined, so it isn’t available on distributed applications. Context attributes are meant for infrastructure, such as shared connection pools.
- At the server-side, add the attribute to the context then forward the request to the JSP page like the following:
getServletContext().setAttribute("name","Hussein Terek");
request.getRequestDispatcher("home.jsp").forward(request, response);
* In JSP, you can access it like:
<h3> My name is getServletContext().getAttribute("name")</h3>
2. Redirect to JSP using query string
The second way of passing data from servlet to JSP is through redirecting the response to the appropriate JSP and appending the attributes in the URL as a query string.
- At the server-side, redirect the response to the JSP page and append the parameters directly in the URL as the following:
response.sendRedirect( "home.jsp?name=Hussein Terek" );
- In JSP, you can access the parameter like:
<h3> My name is <%= request.getParameter("name") %></h3>
This technique is mostly used when you want to pass a reasonable number of simple attributes.
3. Passing Objects from servlet to JSP
In order to pass a business object or POJO from servlet to JSP, you can pass it as an attribute using the setAttribute() method described above.
Following is an example for passing a Student object from servlet to JSP:
At the server-side, the Student object is set as a request attribute:
Student student = new Student();
student.setId("1");
student.setName("Hussein");
student.setAge("25");
request.setAttribute("student", student);
request.getRequestDispatcher("home.jsp").forward(request, response);
In JSP, we print the different attributes of the Student object like the following:
<h3> Student information: </h3>
Id: ${student.id} <br/>
Name: ${student.name} <br/>
Age: ${student.age}
Output:
4. Passing ArrayList from Servlet to JSP
We modify the previous example so that we pass a list of Student objects, again we use the setAttribute() method at the server-side in order to pass the list from servlet to JSP.
List<Student> students = new ArrayList<Student>();
Student student = new Student();
student.setId("1");
student.setName("Hussein");
student.setAge("25");
Student student2 = new Student();
student2.setId("2");
student2.setName("Alex");
student2.setAge("30");
students.add(student);
students.add(student2);
request.setAttribute("students", students);
request.getRequestDispatcher("home.jsp").forward(request, response);
In JSP, we iterate over the list of Student objects as the following:
<h3> Students Information </h3>
<% List<Student> students = (ArrayList<Student>)request.getAttribute("students");
for(Student student : students)
{
out.print("Id: " + student.getId());
out.print("<br/>");
out.print("Name: " + student.getName());
out.print("<br/>");
out.print("Age: " + student.getAge());
out.print("<br/>");
out.print("<br/>");
}
%>
It is worth mentioning that it’s necessary to import List, ArrayList, and Student objects at the top of the JSP page in order to be able to use them inside JSP:
<%@page import="java.util.ArrayList"%>
<%@page import="java.util.List"%>
<%@page import="com.programmer.gate.Student"%>
The output of the above JSP is:
5. Passing HashMap from Servlet to JSP
Suppose that we decided to pass the students in a HashMap instead of ArrayList, to do so we again use the setAttribute() method and we pass the HashMap as the following:
Map<String, Student> students = new HashMap<String, Student>();
Student student = new Student();
student.setId("1");
student.setName("Hussein");
student.setAge("25");
Student student2 = new Student();
student2.setId("2");
student2.setName("Alex");
student2.setAge("30");
students.put("1", student);
students.put("2", student2);
request.setAttribute("students", students);
request.getRequestDispatcher("home.jsp").forward(request, response);
In JSP, we traverse the HashMap as the following:
<h3> Students Information </h3>
<% Map<String,Student> students = (HashMap<String, Student>)request.getAttribute("students");
for(Entry<String, Student> entry : students.entrySet())
{
Student student = entry.getValue();
out.print("Id: " + student.getId());
out.print("<br/>");
out.print("Name: " + student.getName());
out.print("<br/>");
out.print("Age: " + student.getAge());
out.print("<br/>");
out.print("<br/>");
}
%>
In order to use Entry, Map, and HashMap objects in the JSP file, we import them at the top using:
<%@page import="java.util.HashMap"%>
<%@page import="java.util.Map"%>
<%@page import="java.util.Map.Entry"%>
<%@page import="com.programmer.gate.Student"%>
The output of the above JSP looks like this:
Summary
JSP is mostly used as the view component in any Java-based MVC application, its main usage is to present dynamic data processed and generated from server-side controllers like a servlet.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers