How to upload files to a servlet
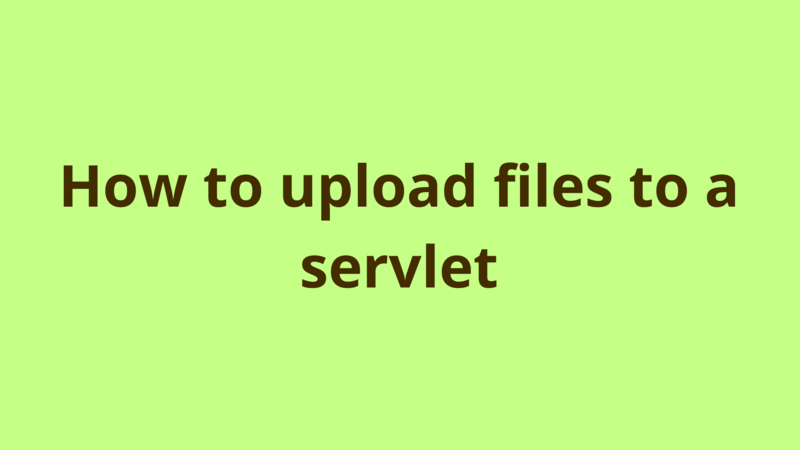
ADVERTISEMENT
Table of Contents
- Introduction
- 1- Upload.html
- 2- Servlet 3.1 (Tomcat 8)
- 3- Servlet 3.0 (Tomcat 7)
- 4- Servlet 2.5 (Tomcat 6)
- Summary
- Next Steps
Introduction
In this tutorial, we discuss how to upload files to a servlet.
Parsing the uploaded file in the servlet depends strictly on the used version of the servlet API, here below we explain how to do this in versions 3.1, 3.0 and 2.5 respectively.
1- Upload.html
Before digging into the servlet, let’s see how would the HTML form looks like:
<form id="upload" method="POST" action="FileUpload" enctype="multipart/form-data">
<input type="file" id="file" name="file" />
<br/>
<input type="submit" id="uploadFile" value="Upload" />
</form>
The above is a very simple HTML form which only holds an input file along with a submit button.
As noticed, we set the encoding type to “multipart/form-data” and define “FileUpload” servlet to handle the request.
2- Servlet 3.1 (Tomcat 8)
In order to parse the uploaded file in Servlet 3.1, first of all, you have to annotate your servlet with @MultipartConfig as the following:
@WebServlet("/FileUpload")
@MultipartConfig
public class FileUpload extends HttpServlet{
}
Inside your doPost(), you can parse the uploaded file using request.getPart() method and read the uploaded file name using getSubmittedFileName().
In the following example, we read the uploaded file and write it in the file system:
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Part partAttr = req.getPart("file");
InputStream is = partAttr.getInputStream();
File file = new File("C:\\Users\\user\\Desktop\\output\\" + partAttr.getSubmittedFileName());
FileOutputStream outputStream = new FileOutputStream(file);
int read = 0;
byte[] bytes = new byte[1024];
while ((read = is.read(bytes)) != -1) {
outputStream.write(bytes, 0, read);
}
}
3- Servlet 3.0 (Tomcat 7)
With Servlet 3.0, we can read the uploaded file just as we did with Servlet 3.1.
The only difference between the 2 versions is how to read the uploaded file name, the getSubmittedFileName() method is not supported in Servlet 3.0.
So, in order to read the uploaded file name, we create a custom utility method which parses the request header and read the file name from within it:
private static String getSubmittedFileName(Part part) {
for (String cd : part.getHeader("content-disposition").split(";")) {
if (cd.trim().startsWith("filename")) {
String fileName = cd.substring(cd.indexOf('=') + 1).trim().replace("\"", "");
return fileName.substring(fileName.lastIndexOf('/') + 1).substring(fileName.lastIndexOf('\\') + 1);
}
}
return null;
}
4- Servlet 2.5 (Tomcat 6)
Well, with Servlet 2.5, things are a bit different, there is no predefined API to read the uploaded files in this version.
So if you’re still using this version and want to read the uploaded files, we recommend the Apache Commons library.
In order to use Apache Commons, you have to add commons-fileupload-1.4.jar and commons-io-2.6.jar to your classpath.
After that, you can parse the uploaded file as below:
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
try {
List<FileItem> items = new ServletFileUpload(new DiskFileItemFactory()).parseRequest(req);
for (FileItem item : items) {
String fileName = FilenameUtils.getName(item.getName());
InputStream is = item.getInputStream();
File file = new File("C:\\Users\\user\\Desktop\\output\\" + fileName);
FileOutputStream outputStream = new FileOutputStream(file);
int read = 0;
byte[] bytes = new byte[1024];
while ((read = is.read(bytes)) != -1) {
outputStream.write(bytes, 0, read);
}
}
} catch (FileUploadException e) {
throw new ServletException("Cannot parse multipart request.", e);
}
}
Summary
In this tutorial, we discuss how to upload files to a servlet.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers