How to upload files with Spring MVC
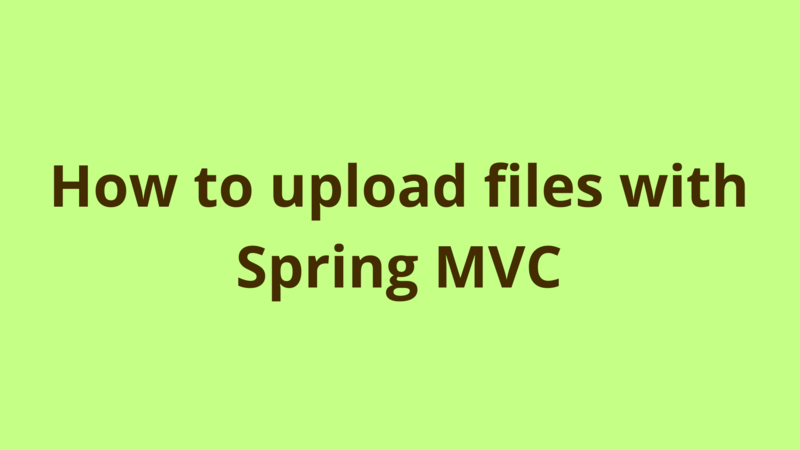
ADVERTISEMENT
Table of Contents
Introduction
In this tutorial, we discuss how to upload files with Spring MVC.
If you’re more interested in doing it the old way using Servlets, then check “How to upload files to a Servlet” tutorial.
1- Upload.html
Before digging into the controller, let’s see how would the HTML form looks like:
<form id="upload" method="POST" action="upload" enctype="multipart/form-data">
<input type="file" id="file" name="file" />
<br/>
<input type="submit" id="uploadFile" value="Upload" />
</form>
The above is a very simple HTML form which only holds an input file along with a submit button.
As noticed, we set the encoding type to “multipart/form-data” and define “/upload” API to handle the request.
2- Controller
I n order to parse the uploaded files at the server-side, you have to create a controller method that maps the “/upload” requests, the method should:
- Consume “multipart/form-data” request type.
- Has an input argument of type MultipartFile[].
The below method parses the uploaded files and saves them to the file system:
@RequestMapping(value = "/upload", method = RequestMethod.POST, consumes = "multipart/form-data")
public void upload(@RequestParam("file") MultipartFile[] filesToBeUploaded, ModelMap modelMap, HttpServletResponse response) {
for(MultipartFile fileToBeUploaded : filesToBeUploaded)
{
File output = new File("C:\\Users\\user\\Desktop\\output\\" + fileToBeUploaded.getOriginalFilename());
try(FileOutputStream outputStream = new FileOutputStream(output); InputStream is = fileToBeUploaded.getInputStream();)
{
int read = 0;
byte[] bytes = new byte[1024];
while ((read = is.read(bytes)) != -1) {
outputStream.write(bytes, 0, read);
}
}
catch(Exception ex)
{
ex.printStackTrace();
}
}
}
As noticed, we parse the uploaded file using getInputStream() method and we get its name through getOriginalFilename() method.
P.S: If you feel confused on the above try/catch syntax, check “Try with resources” tutorial.
Summary
In this tutorial, we discuss how to upload files with Spring MVC.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers