How to write to a plain text file in Java
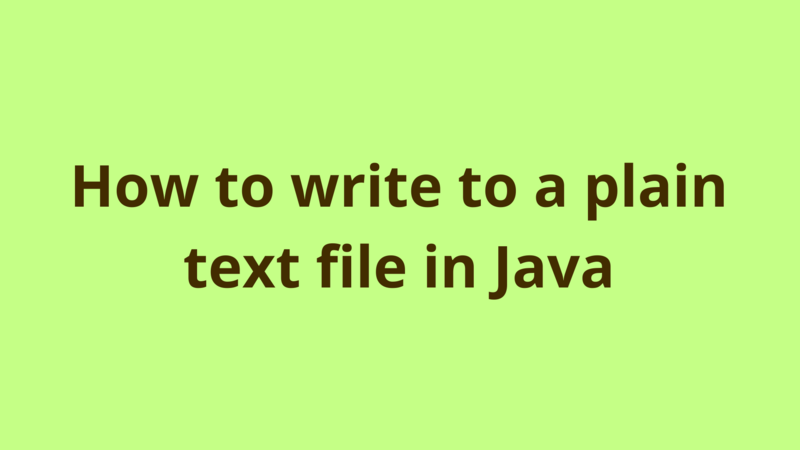
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows several ways to write content to a text file in Java.
The techniques used below are pure JDK and donβt use external libraries.
1- BufferedWriter
The most common and efficient way to write content to a file in Java is through using BufferedWriter as the following:
private static void writeFileUsingBufferedWriter()
{
try(Writer writer = new BufferedWriter(new OutputStreamWriter(new FileOutputStream("new-file.txt"), "UTF-8")))
{
writer.write("First Line");
writer.write("\n");
writer.write("Second Line");
}
catch(IOException ex)
{
ex.printStackTrace();
}
}
This technique is very efficient for writing huge data to a text file.
2- Java nio
Using nio package which is introduced in JDK 7, you can write content to a text file in one line using Files.write() as the following:
private static void writeFileUsingFiles()
{
List<String> lines = Arrays.asList("First Line", "Second Line");
Path file = Paths.get("new-file.txt");
try
{
Files.write(file, lines, Charset.forName("UTF-8"));
}
catch(IOException ex)
{
ex.printStackTrace();
}
}
Summary
This tutorial shows several ways to write content to a text file in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers