Java – Convert InputStream to File
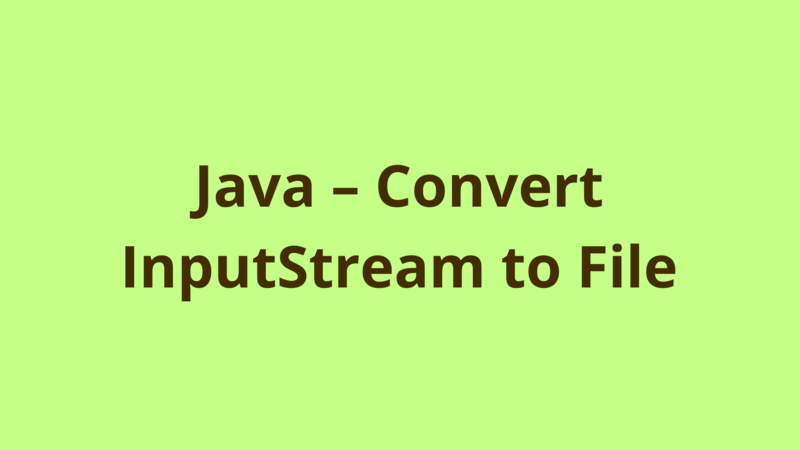
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows several ways to convert InputStream to File in Java.
1- Common way
The common way for converting InputStream to File is through using OutputStream.
You can’t directly create a File object from InputStream. However, you can read the InputStream and write it to a File using FileOutputStream as the following:
public static void convertInputStreamToFileCommonWay(InputStream is) throws IOException
{
OutputStream outputStream = null;
try
{
File file = new File("C:\\Users\\user\\Desktop\\test\\output.txt");
outputStream = new FileOutputStream(file);
int read = 0;
byte[] bytes = new byte[1024];
while ((read = is.read(bytes)) != -1) {
outputStream.write(bytes, 0, read);
}
}
finally
{
if(outputStream != null)
{
outputStream.close();
}
}
}
2- java.nio (Java 8)
Using nio packages exposed by Java 8, you can write an InputStream to a File using Files.copy() utility method.
public static void convertInputStreamToFileNio(InputStream is) throws IOException
{
String outputFile = "C:\\Users\\user\\Desktop\\test\\output.txt";
Files.copy(is, Paths.get(outputFile));
File file = new File(outputFile);
}
3- Apache commons library
Apart from JDK, you can use apache commons library for converting InputStream to File as the following:
public static void convertInputStreamToFileCommonsIO(InputStream is) throws IOException
{
OutputStream outputStream = null;
try
{
File file = new File("C:\\Users\\user\\Desktop\\test\\output.txt");
outputStream = new FileOutputStream(file);
IOUtils.copy(is, outputStream);
}
finally
{
if(outputStream != null)
{
outputStream.close();
}
}
}
Summary
This tutorial shows several ways to convert InputStream to File in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers