Java – Convert InputStream to String
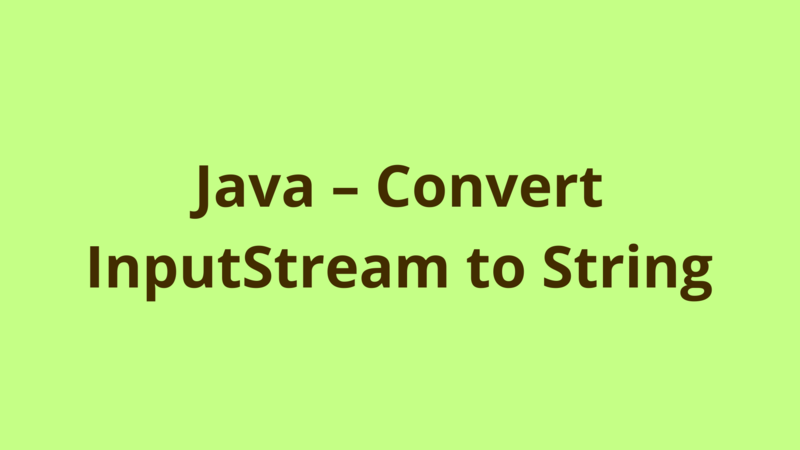
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows several ways to convert an InputStream to a String in Java.
1- BufferedReader
The common way for generating a String out of an InputStream is through using BufferedReader as the following:
public static String convertInputStreamToStringBufferredReader(InputStream is) throws IOException
{
StringBuilder output = new StringBuilder();
try(Reader reader = new BufferedReader(new InputStreamReader(is, Charset.forName(StandardCharsets.UTF_8.name()))))
{
int c = 0;
while((c = reader.read()) != -1){
output.append((char) c);
}
}
return output.toString();
}
2- Scanner
Another common way is to use a Scanner object as the following:
public static String convertInputStreamToStringScanner(InputStream is) throws IOException
{
String output = null;
try(Scanner scanner = new Scanner(is, StandardCharsets.UTF_8.name()).useDelimiter("\\A"))
{
output = scanner.hasNext() ? scanner.next() : "";
}
return output;
}
3- Java 8
With Java 8, you can generate a String out of an InputStream using Collectors and collect() utility method:
public static String convertInputStreamToStringJava8(InputStream is) throws IOException
{
String output = null;
try(BufferedReader reader = new BufferedReader(new InputStreamReader(is)))
{
output = reader.lines().collect(Collectors.joining("\n"));
}
return output;
}
4- Apache Commons library
Apart from JDK, you can use Apache Commons library for converting an InputStream to String using 2 ways:
1st way:
public static String convertInputStreamToStringCommonsIO(InputStream is) throws IOException
{
StringWriter writer = new StringWriter();
IOUtils.copy(is, writer, "UTF-8");
String output = writer.toString();
return output;
}
2nd way:
public static String convertInputStreamToStringCommonsIO(InputStream is) throws IOException
{
String output = IOUtils.toString(is, StandardCharsets.UTF_8.name());
return output;
}
Summary
This tutorial shows several ways to convert an InputStream to a String in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers