Java – Convert List to Array
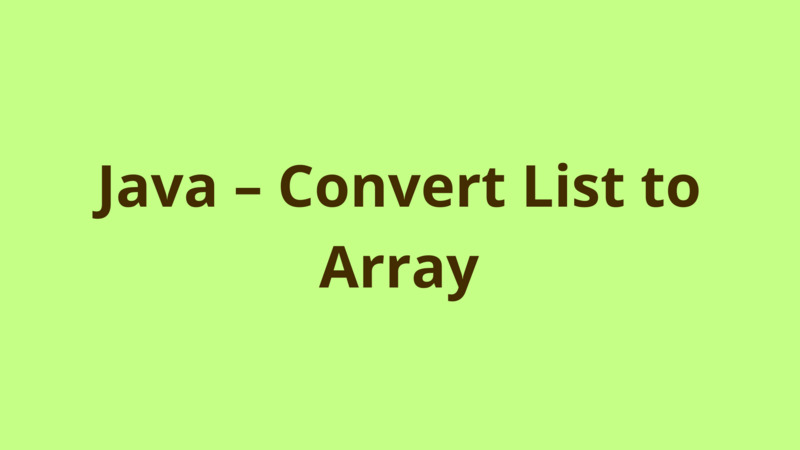
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows several ways to convert a List to Array in Java.
1- toArray()
List provides a utility method called toArray() which accepts an empty array and populates it with the elements of the array list.
public static String[] convertListToArrayUsingToArray(List<String> names)
{
String[] namesArr = new String[names.size()];
namesArr = names.toArray(namesArr);
return namesArr;
}
In the above example, we initialize an array with the same number of elements as the input list, then we populate it using toArray() method.
2- Traditional way
The other way of converting a List to Array is to do it manually through iterating over the elements of the list and filling up an array as the following:
public static String[] convertListToArrayTraditionalWay(List<String> names)
{
String[] namesArr = new String[names.size()];
for(int i=0 ; i<names.size(); i++)
{
namesArr[i] = names.get(i);
}
return namesArr;
}
3- Java 8
With Java 8, you can convert a List to Array in one line using stream() and toArray() utility methods.
public static String[] convertListToArrayJava8(List<String> names)
{
String[] namesArr = names.stream().toArray(String[]::new);
return namesArr;
}
In the above example, we convert the names list into a stream using stream() method and then collect the stream into a new array using toArray().
Summary
This tutorial shows several ways to convert a List to Array in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers