Java – Convert List to Map
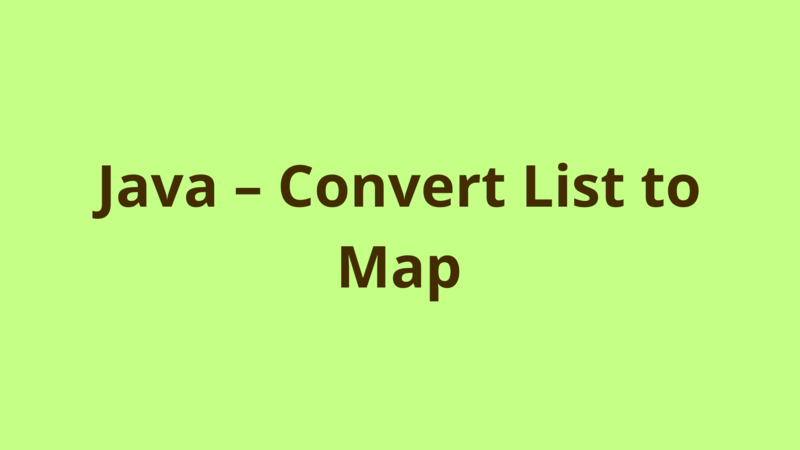
ADVERTISEMENT
Table of Contents
Introduction
This tutorial shows the different ways to convert a List to Map in Java.
1- Java 7 & before
With Java 7 and older releases, the only way to convert a List to Map is to iterate over the list and populate the map manually.
In the following example, we implement a utility method which accepts a list of Student objects and populates out of it a HashMap with id as a key and name as a value.
public static Map<Integer,String> listToHashmapJava7Below(List<Student> students)
{
Map<Integer, String> studentsMap = new HashMap<Integer, String>();
for(Student student : students)
{
studentsMap.put(student.getId(), student.getName());
}
return studentsMap;
}
2- Java 8
With Java 8, you can convert a List to Map in one line using stream() and Collectors.toMap() utility methods.
public static Map<Integer,String> listToHashmapJava8(List<Student> students)
{
Map<Integer, String> studentsMap = students.stream().collect(Collectors.toMap(Student :: getId, Student :: getName));
return studentsMap;
}
Collectors.toMap() method collects a stream as a Map and uses its arguments to decide what key/value to use.
2.1- Java 8 – Handle duplicate keys
Collectors.toMap() fails when converting a list with duplicate items.
In order to handle duplicates, you can pass a third argument which informs toMap() which value to consider when facing duplicate items.
In the following example, we decide to consider the old value or in another meaning to keep the existing value without update each time the map faces a duplicate:
public static Map<Integer,String> listToHashmapJava8WithDuplicates(List<Student> students)
{
Map<Integer, String> studentsMap = students.stream().collect(Collectors.toMap(Student :: getId, Student :: getName
, (oldValue, newValue) -> oldValue));
return studentsMap;
}
If you want to override the existing value on duplicates, use (oldValue, newValue) -> newValue
2.2- Java 8 – Preserve list order
In order to preserve the order of the list items inside the Map, you can pass another parameter to Collectors.toMap() which decides what type of map to use. LinkedHashMap is well known in preserving the order of its entries.
public static Map<Integer,String> listToHashmapJava8PreserveOrder(List<Student> students)
{
Map<Integer, String> studentsMap = students.stream().collect(Collectors.toMap(Student :: getId, Student :: getName
, (oldValue, newValue) -> oldValue,LinkedHashMap::new));
return studentsMap;
}
Summary
This tutorial shows the different ways to convert a List to Map in Java.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers