Make a Random Password Generator | Beginner Java Project
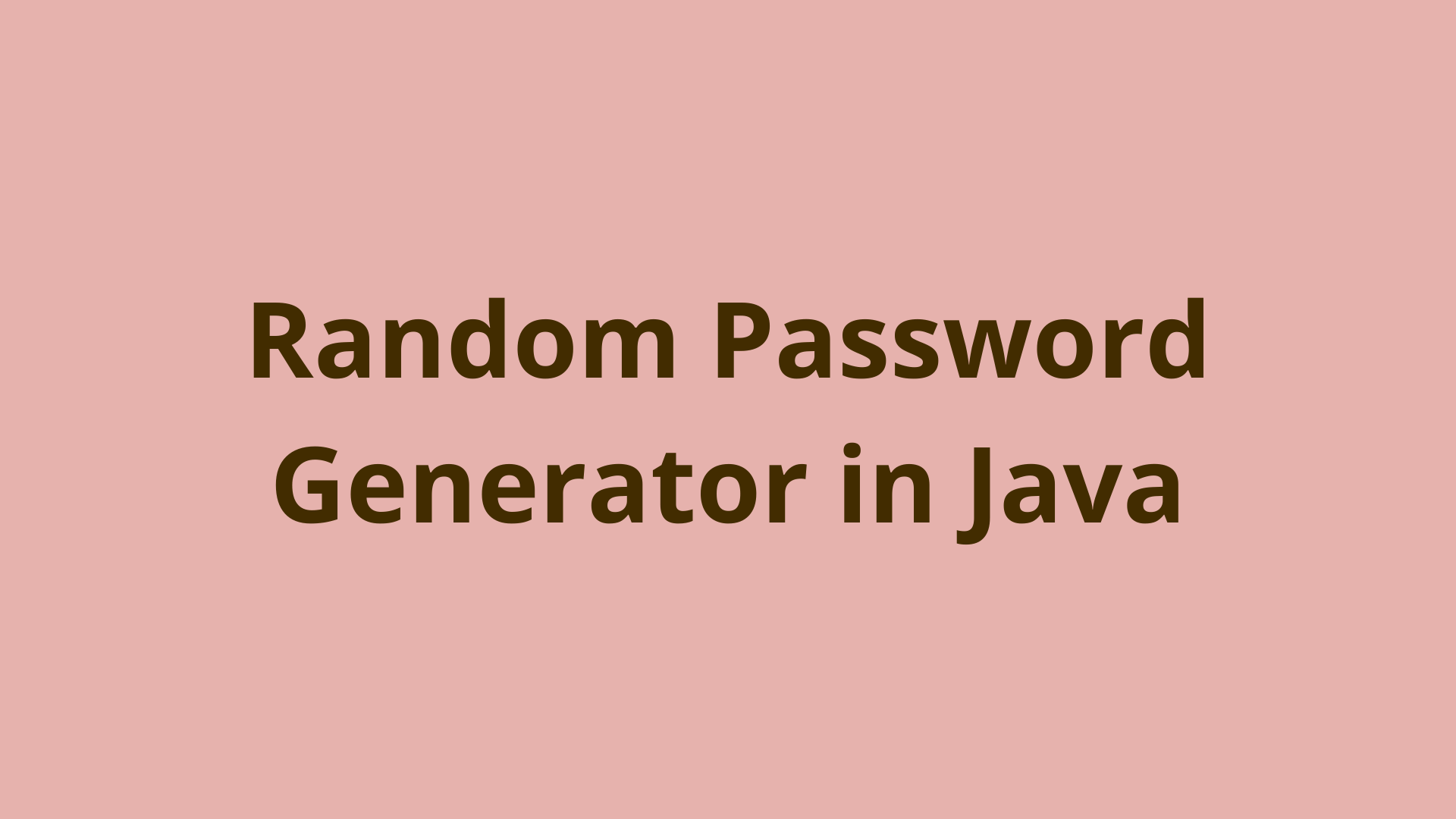
ADVERTISEMENT
Table of Contents
- Project Introduction
- Who is this project for?
- Project Demo
- Steps to Code the Project:
- How do we do each of these steps?
- Step 1: Ask the user to enter the total number of random passwords they want.
- Step 4: Create a random password by looping through the total number of passwords and looping through the length of the passwords.
Project Introduction
Today we will make a random password generator that makes passwords with random numbers and letters!
In order to do this we are going to use ASCII. ASCII is a language in which every possible character is represented by a number. This standard ensures that computers can communicate to each other about characters properly.
This is a more challenging project for beginners, but you certainly have the ability to do it as long as you are familiar with Java concepts such as conditionals, loops, functions, and random numbers.
Watch the tutorial video to see how we code this game step-by-step, and continue reading this post for more details.
Who is this project for?
This project falls under our Juni Java Level 1 coding class for kids. This beginner Java tutorial is for students that want a hard challenge project, about 50-75 lines of code long. You should review variables, conditionals, loops, and input and output beforehand to get the most out of this project.
Some other projects you can try first for more practice with beginner Java are our Java Tic Tac Toe and Java Rock Paper Scissors tutorials.
For learning outcomes, you'll get a lot of practice with functions, random numbers, ASCII, and arrays. This project is estimated to take you about 30-45 minutes, but you should move faster or slower at your own pace!
Project Demo
Before getting started, see how our finished project works for reference. Watch the video, or click run to see the project yourself!
import java.util.Scanner;
class Main {
public static void main(String[] args) {
//Create a Scanner (import it above!) and ask the user how many random passwords they want generated
Scanner in = new Scanner(System.in);
System.out.print("How many random passwords do you want to generate? ");
int total = in.nextInt();
//Ask the user how long they want their random passwords to be
System.out.print("How long do you want your random passwords to be? ");
int length = in.nextInt();
//Create an array of random passwords (Strings)
String[] randomPasswords = new String[total];
//Randomly generate passwords total number of times
for(int i = 0; i < total; i++) {
//Create a variable to store the random password
String randomPassword = "";
//Randomly generate a character for the password length number of times
for(int j = 0; j < length; j++) {
//Add a random lowercase or UPPERCASE character to our randomPassword String
randomPassword += randomCharacter();
}
//Add the random password to your array
randomPasswords[i] = randomPassword;
}
//Print out the random passwords array
printArray(randomPasswords);
}
//Create a function that prints out an array that is passed in as a parameter
public static void printArray(String[] arr) {
for(int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
//Create a function that randomly generates a letter (lowercase or uppercase) or number (0-9) using ASCII
//'0' - '9' => 48-57 in ASCII
//'A' - 'Z' => 65-90 in ASCII
//'a' - 'z' => 97-122 in ASCII
public static char randomCharacter() {
//We multiply Math.random() by 62 because there are 26 lowercase letters, 26 uppercase letters, and 10 numbers, and 26 + 26 + 10 = 62
//This random number has values between 0 (inclusive) and 62 (exclusive)
int rand = (int)(Math.random()*62);
//0-61 inclusive = all possible values of rand
//0-9 inclusive = 10 possible numbers/digits
//10-35 inclusive = 26 possible uppercase letters
//36-61 inclusive = 26 possible lowercase letters
//If rand is between 0 (inclusive) and 9 (inclusive), we can say it's a number/digit
//If rand is between 10 (inclusive) and 35 (inclusive), we can say it's an uppercase letter
//If rand is between 36 (inclusive) and 61 (inclusive), we can say it's a lowercase letter
if(rand <= 9) {
//Number (48-57 in ASCII)
//To convert from 0-9 to 48-57, we can add 48 to rand because 48-0 = 48
int number = rand + 48;
//This way, rand = 0 => number = 48 => '0'
//Remember to cast our int value to a char!
return (char)(number);
} else if(rand <= 35) {
//Uppercase letter (65-90 in ASCII)
//To convert from 10-35 to 65-90, we can add 55 to rand because 65-10 = 55
int uppercase = rand + 55;
//This way, rand = 10 => uppercase = 65 => 'A'
//Remember to cast our int value to a char!
return (char)(uppercase);
} else {
//Lowercase letter (97-122 in ASCII)
//To convert from 36-61 to 97-122, we can add 61 to rand because 97-36 = 61
int lowercase = rand + 61;
//This way, rand = 36 => lowercase = 97 => 'a'
//Remember to cast our int value to a char!
return (char)(lowercase);
}
}
}
What to keep in mind before you start: -Our program asks us to enter the number of random passwords we want and how long they should be. -Then it prints out the list of random passwords it generated. -Note that the random passwords consist of both uppercase and lowercase letters, as well as numbers!
Steps to Code the Project:
- Ask the user to enter the total number of random passwords they want.
- Ask the user how many characters long they want their passwords to be. (12-15 characters is a strong password length!)
- Create a function that generates a random character, which can be a number, a lowercase letter, or an uppercase letter.
- Create a random password by looping through the total number of passwords and looping through the length of the passwords.
- Store each random password you generate in an array.
- Create a function to print out your array of passwords.
How do we do each of these steps?
Step 1: Ask the user to enter the total number of random passwords they want.
-First we need to type import java.util.Scanner at the top of our code. -Next we can make a Scanner variable by typing Scanner in = new Scanner(System.in). -Finally let’s print out a prompt for the user to enter in the number of passwords they want generated and store their answer in an integer. -Hint: Make sure to use in.nextInt() to get the user input as an integer!
Step 2: Ask the user how many characters long they want their passwords to be.
-According to security experts, 12-15 characters is a strong password length! -We can print out a prompt for the user to enter in the length of their passwords and store their answer in an integer, just like what we did for the total number of passwords.
Step 3: Create a function that generates a random character, which can be a number, a lowercase letter, or an uppercase letter.
-According to our ASCII table, '0' - '9' is 48-57 in ASCII, 'A' - 'Z' is 65-90 in ASCII, and 'a' - 'z' is 97-122 in ASCII. This information will be super important later!
The first thing we want to do is to make a random number that represents all possible characters.
-There are 10 numbers, 26 lowercase letters, and 26 uppercase letters, so there are 10 + 26 + 26 = 62 possibilities of characters. -We can write (int)(Math.random() * 62) in order to get a random integer including 0 and excluding 62.
Now that we have established that our random number is between 0 and 61 (inclusive), we want to think about how we will split up the values of our random number to correspond to numbers, uppercase letters, and lowercase letters. -The 10 possible numbers can be between 0 and 9 (inclusive). The 26 possible uppercase letters can be between 10 and 35 (inclusive). The 26 possible lowercase letters can be between 36 and 61 (inclusive).
In other words, if our random number is between 0 and 9 (inclusive), we can say it's a number. If our random number is between 10 and 35 (inclusive), we can say it's an uppercase letter. If our random number is between 36 and 61 (inclusive), we can say it's a lowercase letter.
We can use conditionals to check if our random number corresponds to numbers, uppercase letters, and lowercase letters. Our goal is to shift the random number interval we currently have for numbers, uppercase letters, and lowercase letters to their appropriate ASCII intervals. -Numbers are 48-57 in ASCII, and we currently have them in the random number interval of 0-9, so we need to convert from 0-9 to 48-57. To do this, we can simply add 48 to our random number because 48-0 = 48. -Uppercase letters are 65-90 in ASCII, and we currently have them in the random number interval of 10-35, so we need to convert from 10-35 to 65-90. To do this, we can simply add 55 to our random number because 65-10 = 55. -Lowercase letters are 97-122 in ASCII, and we currently have them in the random number interval of 36-61, so we need to convert from 36-61 to 97-122. To do this, we can simply add 61 to our random number because 97-36 = 61.
Finally, we just need to cast our new integer value into a char and return it!
Step 4: Create a random password by looping through the total number of passwords and looping through the length of the passwords.
-Let’s make an outer for loop that will loop as many times as the total number of passwords. You can use the following notation to do so:
for(int i = 0; i < total; i++)
Inside our outer for loop, we can create a String variable to store our random password and set it to an empty String. Next let’s make an inner for loop that will loop as many times as the length of the password.You can use the following notation to do so:
for(int j = 0; j < length; j++)
Inside our inner for loop, we can call our random character function that we just created and add the random character to our random password String. You can say stringVariable += charVariable to append a character to a String!
Step 5: Store each random password you generate in an array.
-Before our outer for loop, let’s create an array to store each random password we generate. This array will store Strings in it and its length will be equal to the total number of random passwords that the user wants to generate. Once we finish generating each random password, let’s add it to our array.
Hint: Once we finish the inner for loop, we know that we have generated a random password, so right after our inner for loop (but still inside our outer for loop), we can say array[i] = randomPassword.
Step 6: Create a function to print out your array of passwords.
Our function should take in a String array and return void because all we are doing in our function is printing out each element of the array. You can use a for loop to loop through each element of the array and print it out!
This article originally appeared on junilearning.com.
I highly recommend also checking out some Java books, my favorite is Effective Java. Have a great day!
Final Notes
Recommended product: Coding Essentials Guidebook for Developers