Pass data from JSP to Spring controller
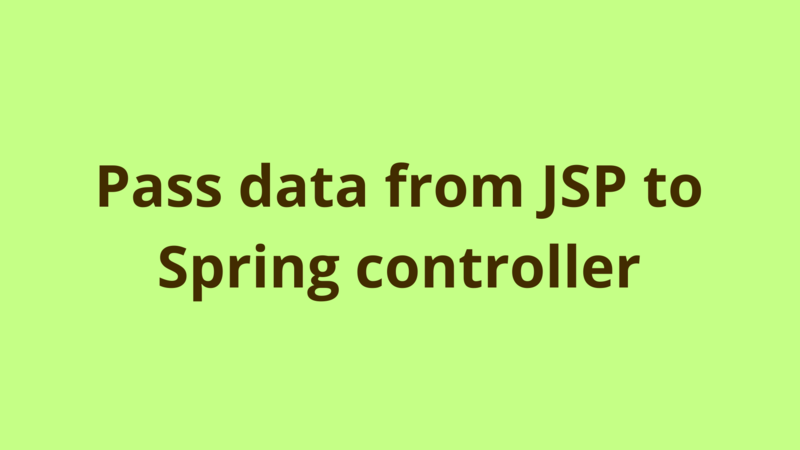
ADVERTISEMENT
Table of Contents
- Introduction
- 1- Pass form fields
- 2- Pass Query Parameters
- 3- Pass Path Parameters
- Summary
- Next Steps
Introduction
In this tutorial, we discuss several ways of passing data from a JSP view to a Spring controller.
1- Pass form fields
The most common scenario for sharing data between JSP views and controllers is through submitting user data to the server side.
Spring makes it very easy to handle user submitted data at the server side, all you have to do is define a Java bean which holds exactly the same field names defined in the JSP form.
In the following example, we ask the user to enter his credentials in order to validate them at the server side using our controller.
The JSP form looks very straightforward, we simply define a form with 2 fields (username and password), and we set this form to be submitted to the “/login” URL.
<form id="login" action="login" method="post">
<label>Username: </label>
<input type="text" name="username">
<br/>
<label>Password: </label>
<input type="text" name="password">
<br/>
<button>Submit</button>
</form>
It’s worth to mention that each input field should have a “name” attribute because Spring uses this attribute implicitly to match the form fields with the java class attributes.
At the server side, we create a java bean called “Credentials” as the following:
public class Credentials {
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
As noticed, the name of the class fields match exactly the name of the form fields.
Inside the controller, we define the login() method which should handle our request:
@RequestMapping(value = "/login", method = RequestMethod.POST)
public void login(Credentials credentials) {
System.out.println("Username= " + credentials.getUsername());
System.out.println("Password= " + credentials.getPassword());
}
The method is defined as “POST” and is set to accept a “Credentials” object, there is no need to annotate the object, by default Spring will compare the form fields with the class fields, if they don’t match then it throws a “400 Bad Request” error.
2- Pass Query Parameters
Redirecting to a different page along with passing filters is a very common thing to do inside your JSP page.
When redirecting to another page, you’re actually doing a GET request.
Suppose we know the user Id and need to redirect to the user details page for displaying the user information. We define a hyperlink as the following:
<a href="/getUserDetails?userId=123"> Get user details</a>
At the server side, we define a GET method which accepts the query parameter and populate the user object as the following:
@RequestMapping(value = "/getUserDetails", method = RequestMethod.GET)
public ModelAndView getUserDetails(@RequestParam int userId) {
ModelAndView model = new ModelAndView("/userDetails");
User user = new User();
user.setId(userId);
user.setFirstname("Alex");
user.setLastname("Alex surname");
model.addObject("user", user);
return model;
}
The above method reads the “userId” query parameter using @RequestParam annotation, then it populates a User object and redirects to a JSP page called userDetails.
We can then display the user information inside userDetails.jsp as the following:
<div>
<label>Id: ${user.id}</label>
<label>First name: ${user.firstname}</label>
<label>Last name: ${user.lastname}</label>
</div>
3- Pass Path Parameters
Another way of passing data in the URL is through path parameters. The path parameters are the parameters that are defined after the method URL without using “?” and attribute names.
Going back to our previous example, suppose we pass the userId as a path parameter instead of a query parameter, then we define our hyperlink as the following:
<a href="/getUserDetails/12345"> Get user details</a>
At the server side, we define our handler method as the following:
@RequestMapping(value = "/getUserDetails/{userId}", method = RequestMethod.GET)
public ModelAndView getUserDetails(@PathVariable int userId) {
ModelAndView model = new ModelAndView("/userDetails");
User user = new User();
user.setId(userId);
user.setFirstname("Alex");
user.setLastname("Alex surname");
model.addObject("user", user);
return model;
}
As noticed, we need to include the path parameter in the RequestMapping annotation inside curly braces “{}” and then define it as a parameter.
If you want to define multiple path parameters, you can simply define your @RequestMapping value as “/getUserDetails//” and then inside your handler method, you define 2 parameters named param1 and param2 alternatively.
Summary
In this tutorial, we discuss several ways of passing data from a JSP view to a Spring controller.
Next Steps
If you're interested in learning more about the basics of Java, coding, and software development, check out our Coding Essentials Guidebook for Developers, where we cover the essential languages, concepts, and tools that you'll need to become a professional developer.
Thanks and happy coding! We hope you enjoyed this article. If you have any questions or comments, feel free to reach out to jacob@initialcommit.io.
Final Notes
Recommended product: Coding Essentials Guidebook for Developers